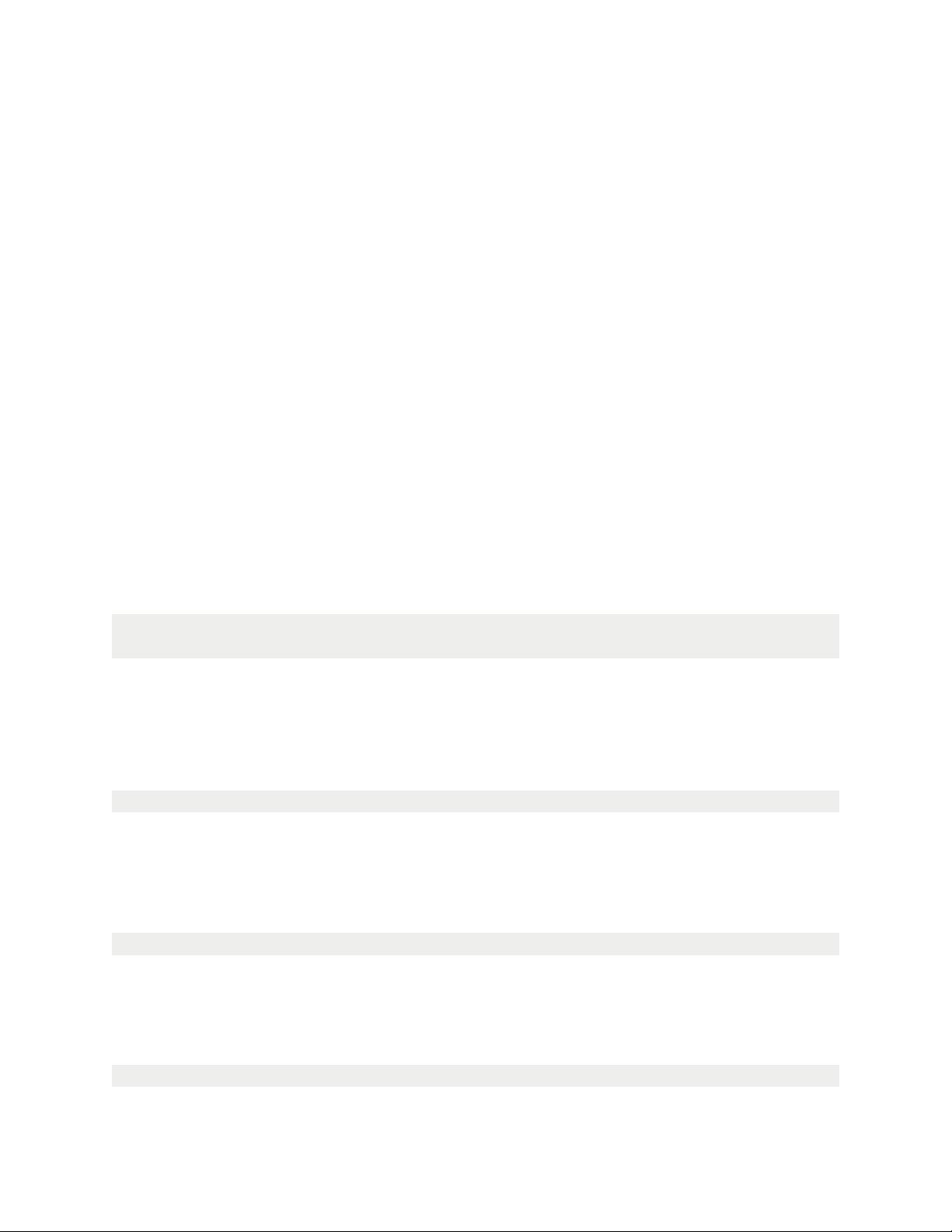
Although this is important to understand, don’t worry if things aren’t yet clear. It won’t take more than a couple of
inserts to see what this truly means. Ultimately, the point is that a collection isn’t strict about what goes in it (it’s
schema-less). Fields are tracked with each individual document. The benefits and drawbacks of this will be explored
in a future chapter.
Let’s get hands-on. If you don’t have it running already, go ahead and start the mongod server as well as a mongo
shell. The shell runs JavaScript. There are some global commands you can execute, like help or exit. Commands
that you execute against the current database are executed against the db object, such as db.help() or db.stats().
Commands that you execute against a specific collection, which is what we’ll be doing a lot of, are executed against
the db.COLLECTION_NAME object, such as db.unicorns.help() or db.unicorns.count().
Go ahead and enter db.help(), you’ll get a list of commands that you can execute against the db object.
A small side note: Because this is a JavaScript shell, if you execute a method and omit the parentheses (), you’ll
see the method body rather than executing the method. I only mention it so that the first time you do it and get a
response that starts with function (...){ you won’t be surprised. For example, if you enter db.help (without the
parentheses), you’ll see the internal implementation of the help method.
First we’ll use the global use helper to switch databases, so go ahead and enter use learn. It doesn’t matter that the
database doesn’t really exist yet. The first collection that we create will also create the actual learn database. Now
that you are inside a database, you can start issuing database commands, like db.getCollectionNames(). If you do
so, you should get an empty array ([ ]). Since collections are schema-less, we don’t explicitly need to create them.
We can simply insert a document into a new collection. To do so, use the insert command, supplying it with the
document to insert:
db.unicorns.insert({name: 'Aurora',
gender: 'f', weight: 450})
The above line is executing insert against the unicorns collection, passing it a single parameter. Internally MongoDB
uses a binary serialized JSON format called BSON. Externally, this means that we use JSON a lot, as is the case with
our parameters. If we execute db.getCollectionNames() now, we’ll see a unicorns collection.
You can now use the find command against unicorns to return a list of documents:
db.unicorns.find()
Notice that, in addition to the data you specified, there’s an _id field. Every document must have a unique _id field.
You can either generate one yourself or let MongoDB generate a value for you which has the type ObjectId. Most of
the time you’ll probably want to let MongoDB generate it for you. By default, the _id field is indexed. You can verify
this through the getIndexes command:
db.unicorns.getIndexes()
What you’re seeing is the name of the index, the database and collection it was created against and the fields included
in the index.
Now, back to our discussion about schema-less collections. Insert a totally different document into unicorns, such as:
db.unicorns.insert({name: 'Leto',
12