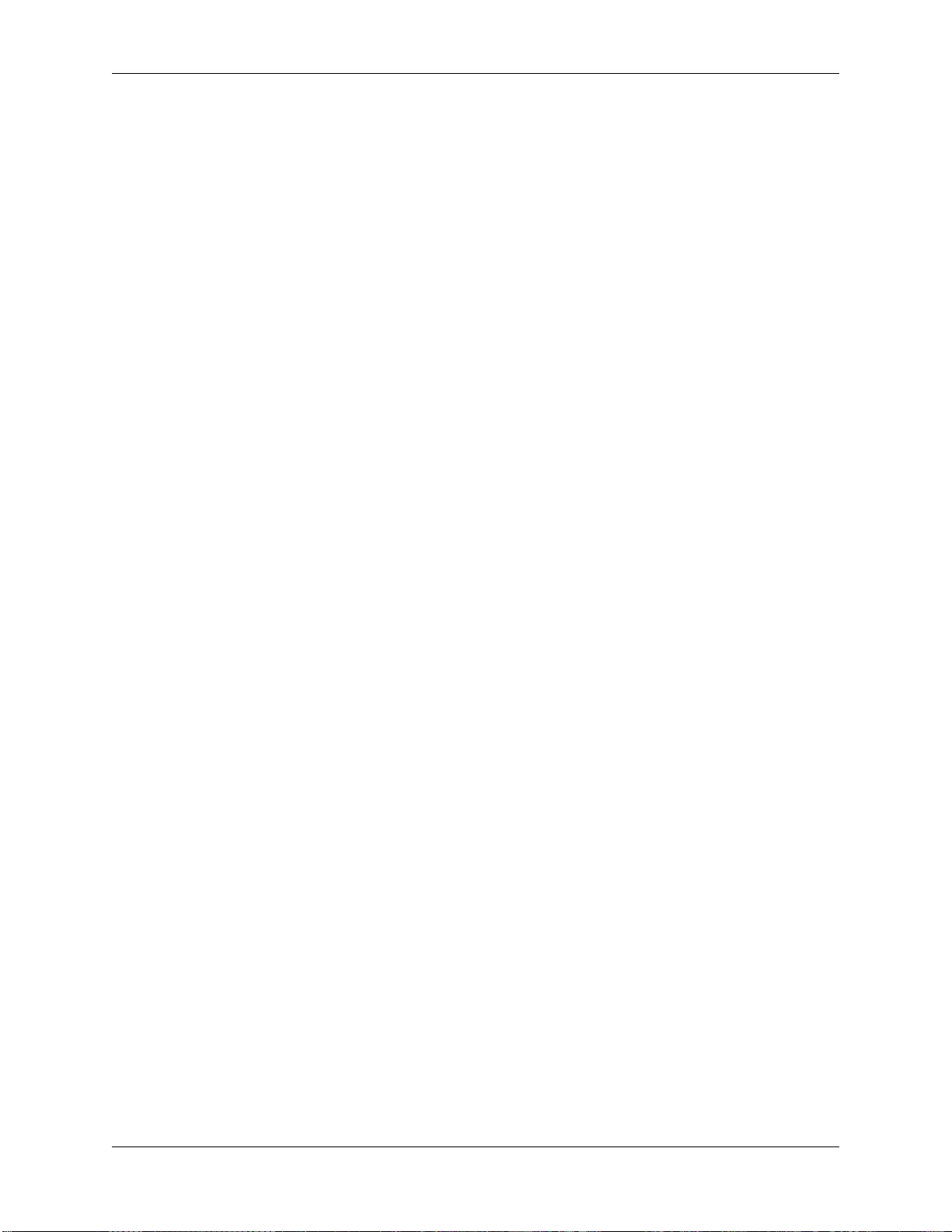
Python Tutorial, Release 2.7
encoding. The list of possible encodings can be found in the Python Library Reference, in the
section on codecs.
根据这个声明,Python 会尝试将文件中的字符编码转为 encoding 编码。并且,它 尽可能的将指定的编
码直接写成 Unicode 文本。在 Python 库参考手册 中 codecs 部份可以找到可用的编码列表(推荐使用
utf-8 处理中文--译者注)。
For example, to write Unicode literals including the Euro currency symbol, the ISO-8859-15
encoding can be used, with the Euro symbol having the ordinal value 164. This script will print
the value 8364 (the Unicode codepoint corresponding to the Euro symbol) and then exit:
例如,可以用 ISO-8859-15 编码可以用来编写包含欧元符号的 Unicode 文本, 其编码值为 164。这个脚
本会输出 8364 (欧元符号的 Unicode 对应编码) 然后退出
# -*- coding: iso-8859-15 -*-
currency = u"€"
print ord(currency)
If your editor supports saving files as UTF-8 with a UTF-8 byte order mark (aka BOM), you can
use that instead of an encoding declaration. IDLE supports this capability if Options/General/
Default Source Encoding/UTF-8 is set. Notice that this signature is not understood in older
Python releases (2.2 and earlier), and also not understood by the operating system for script
files with #! lines (only used on Unix systems).
如果你的文件编辑器可以将文件保存为 UTF-8 格式,并且可以保存 UTF-8 字节序标记 (即 BOM - Byte
Order Mark),你可以用这个来代替编码声明。IDLE可以通过设定 Options/General/Default Source
Encoding/UTF-8 来支持它。需要注意的是旧 版 Python 不支持这个标记(Python 2.2或更早的版本),
也同样不去理解由操 作系统调用脚本使用的 #! 行(仅限于 Unix系统)。
By using UTF-8 (either through the signature or an encoding declaration), characters of most
languages in the world can be used simultaneously in string literals and comments. Using non-
ASCII characters in identifiers is not supported. To display all these characters properly,
your editor must recognize that the file is UTF-8, and it must use a font that supports all the
characters in the file.
使用 UTF-8 内码(无论是用标记还是编码声明),我们可以在字符串和注释中 使用世界上的大部分语言。
标识符中不能使用非 ASCII 字符集。为了正确显示 所有的字符,你一定要在编辑器中将文件保存为 UTF-8
格式,而且要使用支持 文件中所有字符的字体。
2.2.4 The Interactive Startup File 交互式环境的启动文件
When you use Python interactively, it is frequently handy to have some standard commands executed
every time the interpreter is started. You can do this by setting an environment variable named
PYTHONSTARTUP to the name of a file containing your start-up commands. This is similar to the
.profile feature of the Unix shells.
使用 Python 解释器的时候,我们可能需要在每次解释器启动时执行一些命令。 你可以在一个文件中包含
你想要执行的命令,设定一个名为 PYTHONSTARTUP 的 环境变量来指定这个文件。这类似于 Unix shell的
.profile 文件。
This file is only read in interactive sessions, not when Python reads commands from a script,
and not when /dev/tty is given as the explicit source of commands (which otherwise behaves like
an interactive session). It is executed in the same namespace where interactive commands are
executed, so that objects that it defines or imports can be used without qualification in the
interactive session. You can also change the prompts sys.ps1 and sys.ps2 in this file.
2.2. The Interpreter and Its Environment 解释器及其环境 11