Android EditText禁用特定字符并显示提示
在Android编程中,有时我们需要对用户在EditText中的输入进行限制,比如禁止输入特定字符或格式错误的文本。本文将详细讲解如何实现这一功能,让用户在输入不符合规则的字符时能够实时看到相关的提示信息。 首先,我们需要创建一个布局文件,包含一个EditText和一个TextView用于显示提示信息。在提供的代码片段中,我们看到了一个简单的布局结构,包含两个TextView(用于显示提示信息)和一个EditText。布局文件中设置了适当的内边距以便于用户体验。 ```xml <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:text="@string/text_num" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_below="@+id/num" android:text="@string/text_error" /> <EditText android:id="@+id/editText1" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@+id/textView1" android:inputType="numberDecimal" /> </RelativeLayout> ``` 在这个例子中,EditText的`inputType`属性设置为`numberDecimal`,这意味着用户只能输入数字和小数点,这是基本的输入限制。然而,如果我们要阻止用户输入特定字符(例如除数字和小数点之外的其他字符),我们需要在Java代码中添加监听器。 在Activity的Java代码中,我们可以添加一个TextWatcher来监听EditText的文本变化。TextWatcher有三个方法:`beforeTextChanged()`, `onTextChanged()`, 和 `afterTextChanged()`。在这里,我们主要关注`afterTextChanged()`,它会在每次用户输入完成时调用。 ```java public class MainActivity extends AppCompatActivity { private EditText editText; private TextView errorTextView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); editText = findViewById(R.id.editText1); errorTextView = findViewById(R.id.textView2); // 添加TextWatcher editText.addTextChangedListener(new TextWatcher() { @Override public void beforeTextChanged(CharSequence s, int start, int count, int after) {} @Override public void onTextChanged(CharSequence s, int start, int before, int count) {} @Override public void afterTextChanged(Editable s) { String input = s.toString(); if (!isValidInput(input)) { errorTextView.setText("输入包含非法字符,请检查!"); } else { errorTextView.setText(""); } } }); } // 验证输入是否合法 private boolean isValidInput(String input) { // 在这里定义你想要允许的字符集合 String allowedChars = "0123456789."; for (char c : input.toCharArray()) { if (!allowedChars.contains(String.valueOf(c))) { return false; } } return true; } } ``` 在`afterTextChanged()`方法中,我们获取当前的输入文本,然后通过`isValidInput()`方法检查输入是否包含不允许的字符。如果输入包含非法字符,我们就更新错误提示TextView,显示错误信息;否则,清除错误提示。 `isValidInput()`函数遍历输入字符串的每个字符,与预定义的允许字符集合进行比较。如果输入的字符不在允许的集合中,就返回false,表示输入不合法。 通过这种方式,我们可以在Android应用中实现对EditText输入的自定义验证,确保用户只能输入我们允许的字符,并在输入不合规时提供实时反馈。这个方法适用于各种场景,如限制输入的长度、格式或者特定字符等。
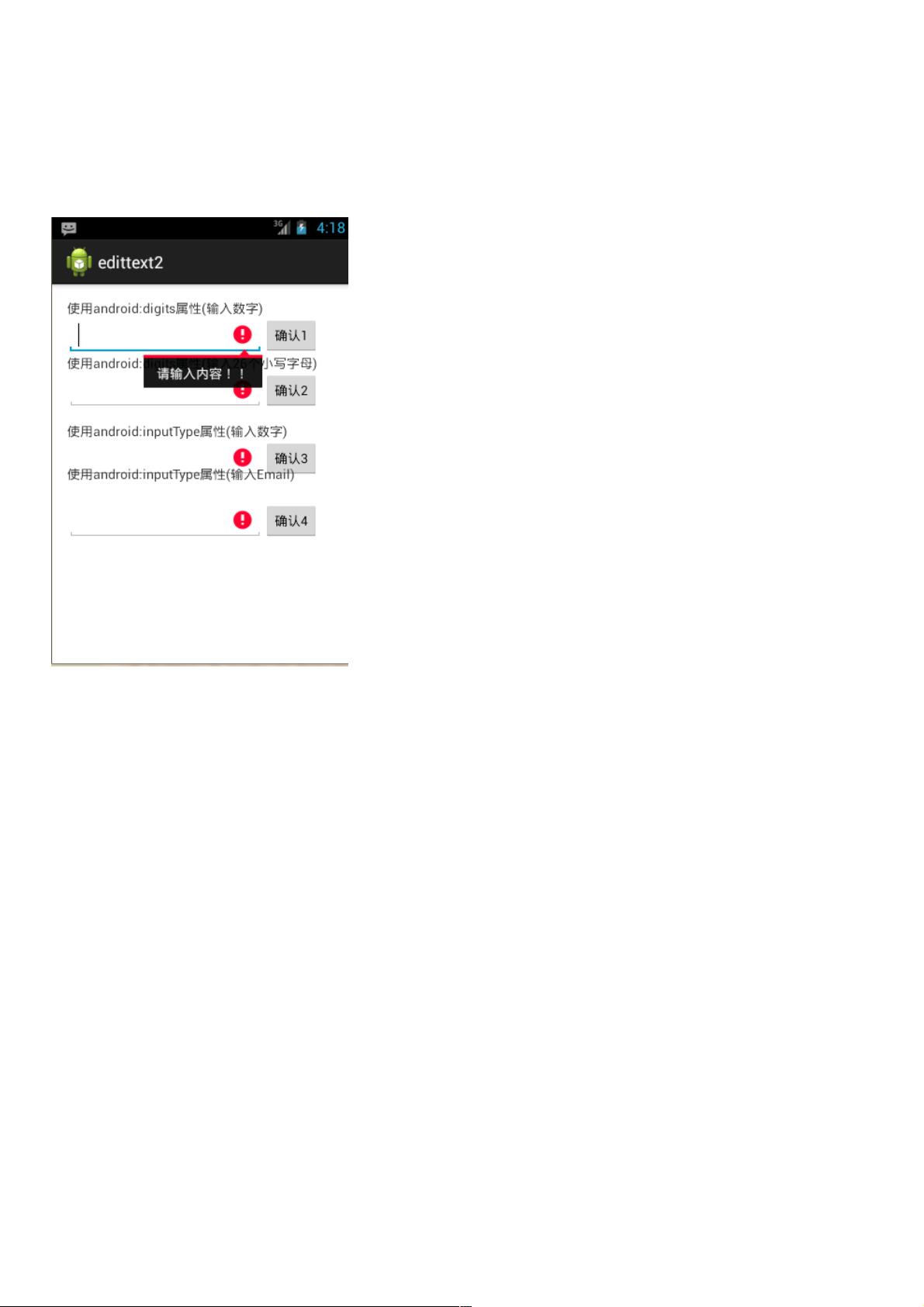
下载后可阅读完整内容,剩余3页未读,立即下载
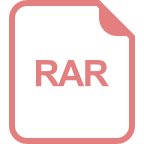
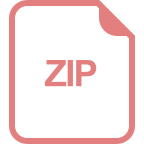
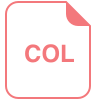
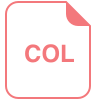
















- 粉丝: 4
- 资源: 898
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 十种常见电感线圈电感量计算公式详解
- 军用车辆:CAN总线的集成与优势
- CAN总线在汽车智能换档系统中的作用与实现
- CAN总线数据超载问题及解决策略
- 汽车车身系统CAN总线设计与应用
- SAP企业需求深度剖析:财务会计与供应链的关键流程与改进策略
- CAN总线在发动机电控系统中的通信设计实践
- Spring与iBATIS整合:快速开发与比较分析
- CAN总线驱动的整车管理系统硬件设计详解
- CAN总线通讯智能节点设计与实现
- DSP实现电动汽车CAN总线通讯技术
- CAN协议网关设计:自动位速率检测与互连
- Xcode免证书调试iPad程序开发指南
- 分布式数据库查询优化算法探讨
- Win7安装VC++6.0完全指南:解决兼容性与Office冲突
- MFC实现学生信息管理系统:登录与数据库操作

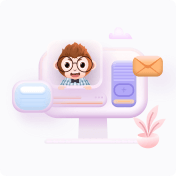
