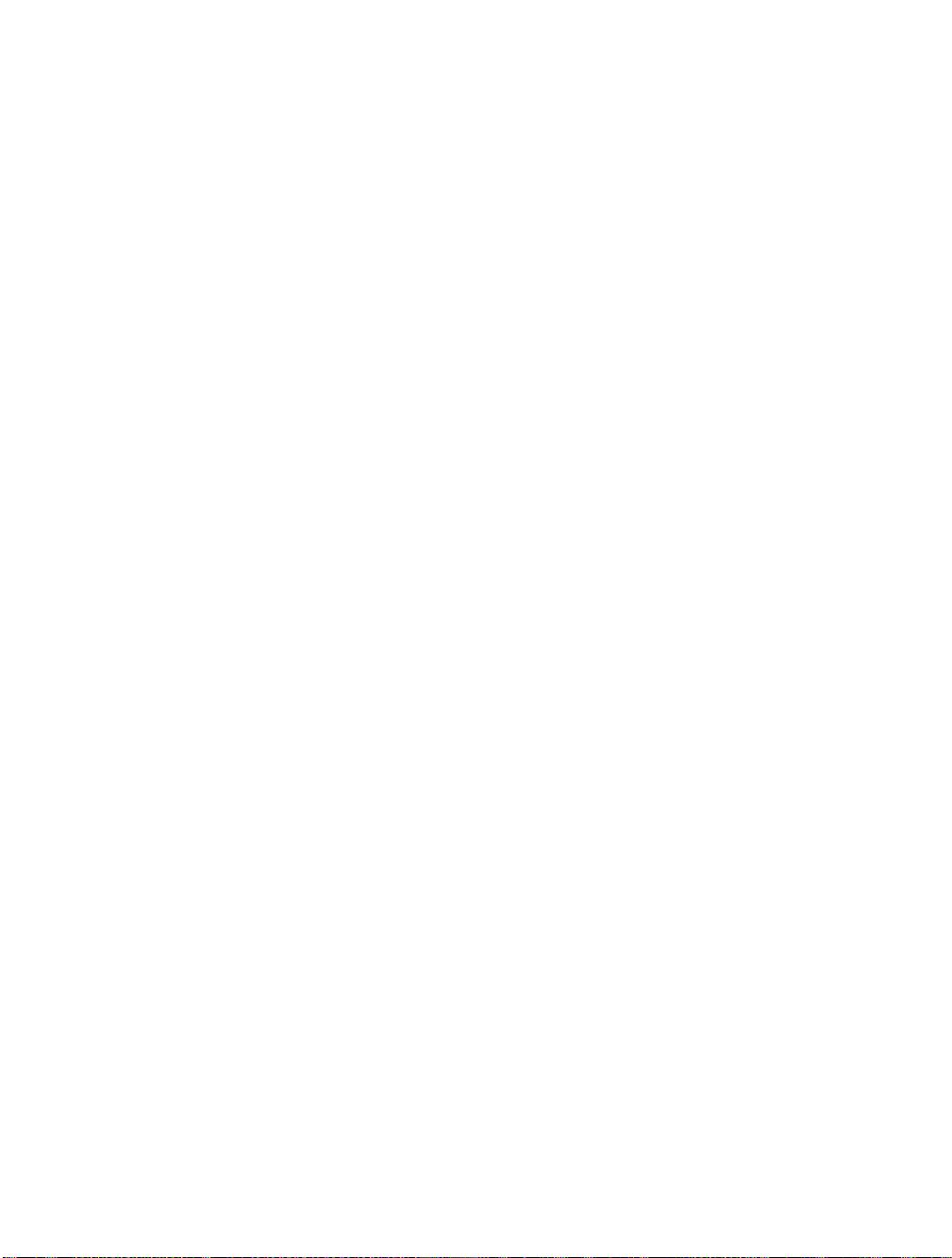
xviii Contents in Detail
Large Files: One Million Digits ................................. 189
Is Your Birthday Contained in Pi? ............................... 190
Exercise 10-1: Learning Python ................................. 191
Exercise 10-2: Learning C .................................... 191
Writing to a File.................................................. 191
Writing to an Empty File ..................................... 191
Writing Multiple Lines ....................................... 192
Appending to a File......................................... 193
Exercise 10-3: Guest ........................................ 193
Exercise 10-4: Guest Book .................................... 193
Exercise 10-5: Programming Poll................................ 193
Exceptions...................................................... 194
Handling the ZeroDivisionError Exception ......................... 194
Using try-except Blocks ...................................... 194
Using Exceptions to Prevent Crashes ............................. 195
The else Block............................................. 196
Handling the FileNotFoundError Exception ......................... 197
Analyzing Text ............................................ 198
Working with Multiple Files ................................... 199
Failing Silently ............................................ 200
Deciding Which Errors to Report................................ 201
Exercise 10-6: Addition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 201
Exercise 10-7: Addition Calculator .............................. 202
Exercise 10-8: Cats and Dogs ................................. 202
Exercise 10-9: Silent Cats and Dogs ............................. 202
Exercise 10-10: Common Words ............................... 202
Storing Data .................................................... 202
Using json.dump() and json.load()............................... 203
Saving and Reading User-Generated Data ......................... 204
Refactoring............................................... 206
Exercise 10-11: Favorite Number ............................... 208
Exercise 10-12: Favorite Number Remembered ..................... 208
Exercise 10-13: Verify User ................................... 208
Summary ...................................................... 208
11
TESTING YOUR CODE 209
Testing a Function ................................................ 210
Unit Tests and Test Cases ..................................... 211
A Passing Test ............................................ 211
A Failing Test . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 212
Responding to a Failed Test ................................... 213
Adding New Tests.......................................... 214
Exercise 11-1: City, Country ................................... 215
Exercise 11-2: Population..................................... 216
Testing a Class .................................................. 216
A Variety of Assert Methods ................................... 216
A Class to Test ............................................ 217
Testing the AnonymousSurvey Class ............................. 218
The setUp() Method ......................................... 220
Exercise 11-3: Employee ..................................... 221
Summary ...................................................... 222