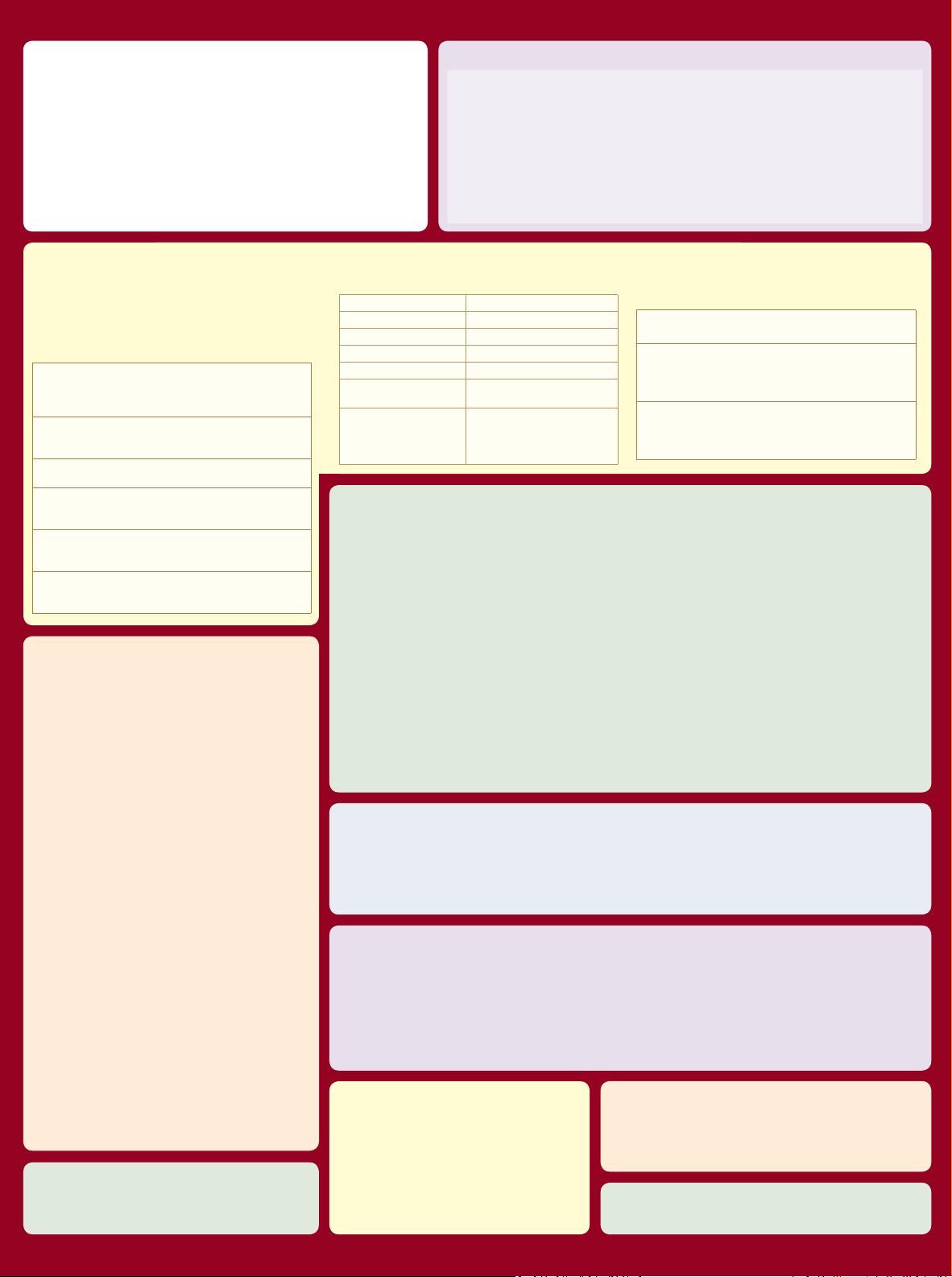
www.khronos.org/webgl©2011 Khronos Group - Rev. 0211
WebGL 1.0 API Quick Reference Card - Page 1
Whole Framebuer Operaons
[5.13.3]
void clear(ulong mask)
[5.13.11]
mask:
Bitwise OR of {COLOR, DEPTH, STENCIL}_BUFFER_BIT
void clearColor(
oat
red,
oat
green,
oat
blue,
oat
alpha)
void clearDepth(
oat
depth)
depth:
Clamped to the range 0 to 1.
void clearStencil(int s)
void colorMask(bool red, bool green, bool blue, bool alpha)
void depthMask(bool ag)
void stencilMask(uint mask)
void stencilMaskSeparate(enum face, uint mask)
face:
FRONT, BACK, FRONT_AND_BACK
WebGL
®
is a soware interface for accessing graphics hardware
from within a web browser. Based on OpenGL ES 2.0, WebGL allows a
programmer to specify the objects and operaons involved in producing
high-quality graphical images, specically color images of 3D objects.
• [n.n.n] refers to secons in the WebGL 1.0 specicaon, available at
www.khronos.org/webgl
• Content marked in purple does not have a corresponding funcon in
OpenGL ES. The OpenGL ES 2.0 specicaon is available at
www.khronos.org/registry/gles
WebGL funcon calls behave idencally to their OpenGL ES counterparts
unless otherwise noted.
ArrayBuer and Typed Arrays
[5.12]
Data is transferred to WebGL using ArrayBuer and views.
Buers represent unstructured binary data, which can be
modied using one or more typed array views.
Buers
ArrayBuer(ulong byteLength)
ulong byteLength: read-only, length of view in bytes.
Creates a new buer. To modify the data, create one or more
views referencing it.
Views
In the following, ViewType may be Int8Array, Int16Array,
Int32Array, Uint8Array, Uint16Array, Uint32Array, Float32Array.
ViewType(ulong length)
Creates a view and a new underlying buer.
ulong length: Read-only, number of elements in this view.
ViewType(ViewType other)
Creates new underlying buer and copies ‘other’ array.
ViewType(type[] other)
Creates new underlying buer and copies ‘other’ array.
ViewType(ArrayBuer buer, [oponal] ulong byteOset,
[oponal] ulong length)
Create a new view of given buer, starng at oponal byte
oset, extending for oponal length elements.
ArrayBuer buer: Read-only, buer backing this view
ulong byteOset: Read-only, byte oset of view start in buer
ulong length: Read-only, number of elements in this view
Other Properes
ulong byteLength: Read-only, length of view in bytes.
const ulong BYTES_PER_ELEMENT: element size in bytes.
Methods
view[i] = get/set element i
s
et(ViewType other, [oponal] ulong oset)
se
t(type[] other, [oponal] ulong oset)
Replace elements in this view with those from other, starng
at oponal oset.
ViewType subset(long begin, [oponal] long end)
Return a subset of this view, referencing the same underlying
buer.
The WebGL Context and getContext() [2.5]
This object manages OpenGL state
and renders to the a drawing buer,
which must is also be created at the
same me of as the context creaon.
Create the
WebGLRenderingContext
object and drawing buer by calling
the getContext method of a given
HTMLCanvasElement
object with the
exact string ‘webgl’. The drawing buer
is also created by getContext.
For example:
<!DOCTYPE html>
<html><body>
<canvas id=”c”></canvas>
<script type=”text/javascript”>
var canvas = document.getElementById(“c”);
var gl = canvas.getContext(“webgl”);
gl.clearColor(1.0, 0.0, 0.0, 1.0);
gl.clear(gl.COLOR_BUFFER_BIT);
</script>
</body></html>
WebGLObject [5.3]
This is the parent interface for all WebGL resource objects.
Resource interface objects:
WebGLBuer [5.4] OpenGL Buer Object.
WebGLProgram [5.6] OpenGL Program Object.
WebGLRenderbuer [5.7] OpenGL Renderbuer Object.
WebGLShader [5.8] OpenGL Shader Object.
WebGLTexture [5.9] OpenGL Texture Object.
WebGLUniformLocaon [5.10] Locaon of a uniform variable in a
shader program.
WebGLAcveInfo [5.11] Informaon returned from calls to
getAcveArib and getAcveUniform.
Has the following read-only
properes: name, locaon, size, type.
WebGLRenderingContext
[5.13]
This is the prinicpal interface in WebGL. The funcons listed on
this reference card are available within this interface.
Aributes:
canvas Type: HTMLCanvasElement
A reference to the canvas element which created this context.
drawingBuerWidth Type: GLsizei
The actual width of the drawing buer, which may dier from the
width aribute of the HTMLCanvasElement if the implementaon is
unable to sasfy the requested width or height.
drawingBuerHeight Type: GLsizei
The actual height of the drawing buer, which may dier from the
height aribute of the HTMLCanvasElement if the implementaon is
unable to sasfy the requested width or height
Interfaces
Interfaces are oponal requests and may be ignored by an
implementaon. See getContextAributes for actual values.
WebGLContextAributes
[5.2]
This interface contains requested drawing surface aributes
and is passed as the second parameter to getContext.
Aributes:
alpha Default: true
If true, requests a drawing buer with an alpha channel for the
purposes of performing OpenGL desnaon alpha operaons
and composing with the page.
depth
De
fault: true
If true, requests drawing buer with a depth buer of at least
16 bits.
stencil Default: false
If true, requests a stencil buer of at least 8 bits.
analias
De
fault: true
If true, requests drawing buer with analiasing using its choice
of technique (mulsample/supersample) and quality.
premulpliedAlpha Default: true
If true, requests drawing buer which contains colors with
premulplied alpha. (Ignored if Alpha is false.)
preserveDrawingBuer
De
fault: false
If true, requests that contents of the drawing buer remain in
between frames, at potenal performance cost.
View and Clip
[5.13.3 - 5.13.4]
The viewport species the ane transformaon of x
and y from normalized device coordinates to window
coordinates. Drawing buer size is determined by the
HTMLCanvasElement.
void depthRange(oat zNear, oat zFar)
zNear: Clamped to the range 0 to 1 Must be <= zFar
zFar: Clamped to the range 0 to 1.
void scissor(int x, int y, long width, long height)
void viewport(int x, int y, long width, long height)
Per-Fragment Operaons
[5.13.3]
void
blendColor(oat red, oat green, oat blue, oat alpha)
void blendEquaon(enum mode)
mode: See modeRGB for blendEquaonSeparate
void blendEquaonSeparate(enum modeRGB,
enum modeAlpha)
modeRGB, and modeAlpha: FUNC_ADD, FUNC_SUBTRACT,
FUNC_REVERSE_SUBTRACT
void blendFunc(enum sfactor, enum dfactor)
sfactor: Same as for dfactor, plus SRC_ALPHA_SATURATE
dfactor: ZERO, ONE, [ONE_MINUS_]SRC_COLOR,
[ONE_MINUS_]DST_COLOR, [ONE_MINUS_]SRC_ALPHA,
[ONE_MINUS_]DST_ALPHA, [ONE_MINUS_]CONSTANT_COLOR,
[ONE_MINUS_]CONSTANT_ALPHA
Note: Src and dst factors may not both reference constant color
void blendFuncSeparate(enum srcRGB, enum dstRGB,
enum srcAlpha, enum dstAlpha)
srcRGB, srcAlpha: See sfactor for blendFunc
dstRGB, dstAlpha: See dfactor for blendFunc
Note: Src and dst factors may not both reference constant color
void depthFunc(enum func)
func: NEVER, ALWAYS, LESS, EQUAL, LEQUAL, GREATER,
GEQUAL, NOTEQUAL
void sampleCoverage(oat value, bool invert)
void stencilFunc(enum func, int ref, uint mask)
func: NEVER, ALWAYS, LESS, LEQUAL, [NOT]EQUAL, GREATER,
GEQUAL
void stencilFuncSeparate(enum face, enum func, int ref,
uint mask)
face: FRONT, BACK, FRONT_AND_BACK
func: NEVER, ALWAYS, LESS, LEQUAL, [NOT]EQUAL, GREATER,
GEQUAL
void stencilOp(enum fail, enum zfail, enum zpass)
fail, zfail, and zpass: KEEP, ZERO, REPLACE, INCR, DECR, INVERT,
INCR_WRAP, DECR_WRAP
void
stencilOpSeparate(enum face, enum fail, enum zfail,
enum zpass)
face: FRONT, BACK, FRONT_AND_BACK
fail, zfail, and zpass: See fail, zfail, and zpass for stencilOp
Buer Objects
[5.13.5]
Once bound, buers may not be rebound with a dierent Target.
void bindBuer(enum target, Object buer)
target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER
void buerData(enum target, long size, enum usage)
target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER
usage: STATIC_DRAW, STREAM_DRAW, DYNAMIC_DRAW
void buerData(enum target, Object data, enum usage)
target and usage: Same as for buerData above
void buerSubData(enum target, long oset, Object data)
target: ARRAY_BUFFER, ELEMENT_ARRAY_BUFFER
Object createBuer()
Note: Corresponding OpenGL ES funcon is GenBuers
void deleteBuer(Object buer)
any getBuerParameter(enum target, enum pname)
target: ARRAY_BUFFER, ELEMENT_ ARRAY_BUFFER
pname: BUFFER_SIZE, BUFFER_USAGE
bool isBuer(Object buer)
Detect and Enable Extensions [5.13.14]
string[ ] getSupportedExtensions()
object getExtension(string name)
Rasterizaon
[5.13.3]
void cullFace(enum mode)
mode: BACK, FRONT_AND_BACK,
FRONT
void frontFace(enum mode)
mode: CCW, CW
void lineWidth(oat width)
void polygonOset(oat factor,
oat units)
Detect context lost events [5.13.13]
bool isContextLost()