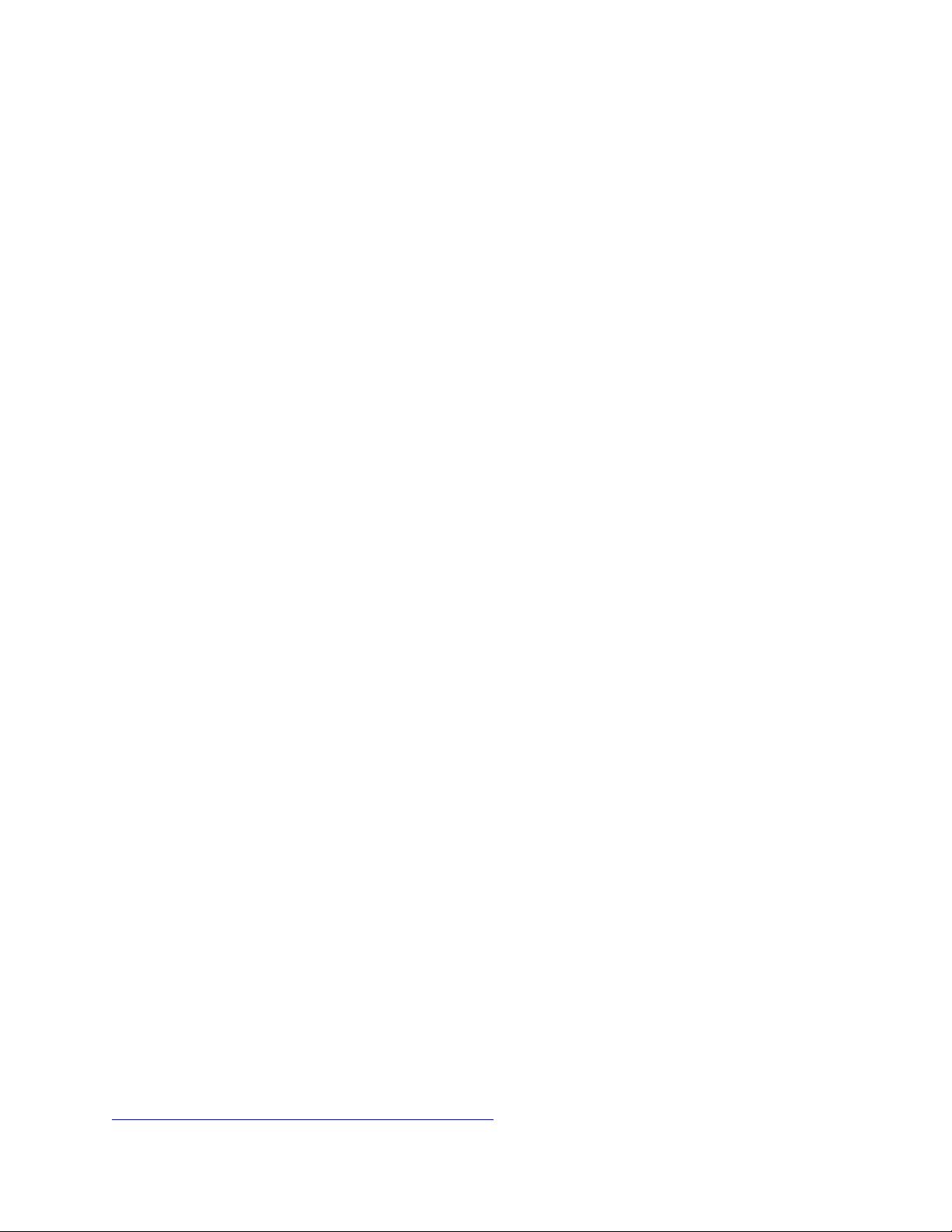
Please post comments or corrections to the Author online forum at
http://www.manning-sandbox.com/forum.jspa?forumID=295
NHibernate support a feature called lazy loading to solve this problem. Basically, when loading the user,
you can decide between loading the items or not. If you choose not to do so, this collection will be
transparently initialized when you need it.
There are many implications in using these features; we will progressively cover them in this book.
Caching
Tracking entities implies keeping their references in some place. NHibernate uses what is called a cache. This
cache is indispensable to implement the Unit of Work pattern. However, it can also make applications more
efficient. We deeply cover the concept of caching in chapter 5, section 5.3 “Caching theory and practice”.
A cache is used by the identity map of NHibernate to avoid loading an entity many times. And this cache
can be shared by transactions and even applications.
Let’s say that we build a website for our auction application. Our visitors may be interested by some items.
Without a cache, these items will be loaded from the database each time a visitor wants to see it. With few
lines, we can ask NHibernate to cache these items and enjoy the performance gain.
1.3.3 Complex queries and ADO.NET Entity Framework
This is the last but not the least feature related to persistence. In section 1.2.5, we talked about CRUD
operations. We have discovered some features related to Create, Update and Delete (all related to the Unit of
Work pattern). Now, we are going to talk about Retrieve operations; that is searching and loading information.
We know that we can easily generate code to load an entity using its identifier (primary key in the context
of relational database). But in real-world applications, users rarely deal with identifiers; instead they use some
criteria to run a search and then pick the information they want.
Implementing a query engine
If you are familiar with SQL, you know that you can write very complex queries using the SELECT ...
FROM ... WHERE ...
construct. But if you work with business objects, you would then have to transform
the results of your SQL queries into entities. We already advertised the benefits of working with entities,
therefore it might make more sense to take advantage of those benefits even when querying the database .
Based on the fact that NHibernate can load and save entities, we can deduce that it knows how each entity
is mapped to the database. When we ask for an entity by it’s identifier, NHibernate knows how to find it. So,
we should be able to express a query using entity names and properties, and then NHibernate should be able to
convert that into a corresponding SQL query understood by the relational database.
NHibernate provides two query APIs: The first is called the Hibernate Query Language. HQL is similar to
SQL in many ways, but also has some useful object-oriented features. Note that you can query NHibernate
using plain old SQL, but as you will learn, using HQL offers several advantages.
The second query API is called the Query by Criteria API (QBC). It provides a set of type-safe classes to
build queries in your chosen .NET language. This means that if you’re using Visual Studio, you’ll benefit from
the inline error reporting and Intellisense™!
To give you a foretaste of the power of these APIs, we are going to build three simple queries. Firstly, here
is some HQL. It finds all bids for items, where the sellers name starts with the letter K:
from Bid bid
where bid.Item.Seller.Name like 'K%'
As you can see, it’s very easy to understand. If you want to write some SQL to do the same thing, you would
need something a little more verbose, along the lines of:
Licensed to Jason Wilden <jasonwilden@internode.on.net>