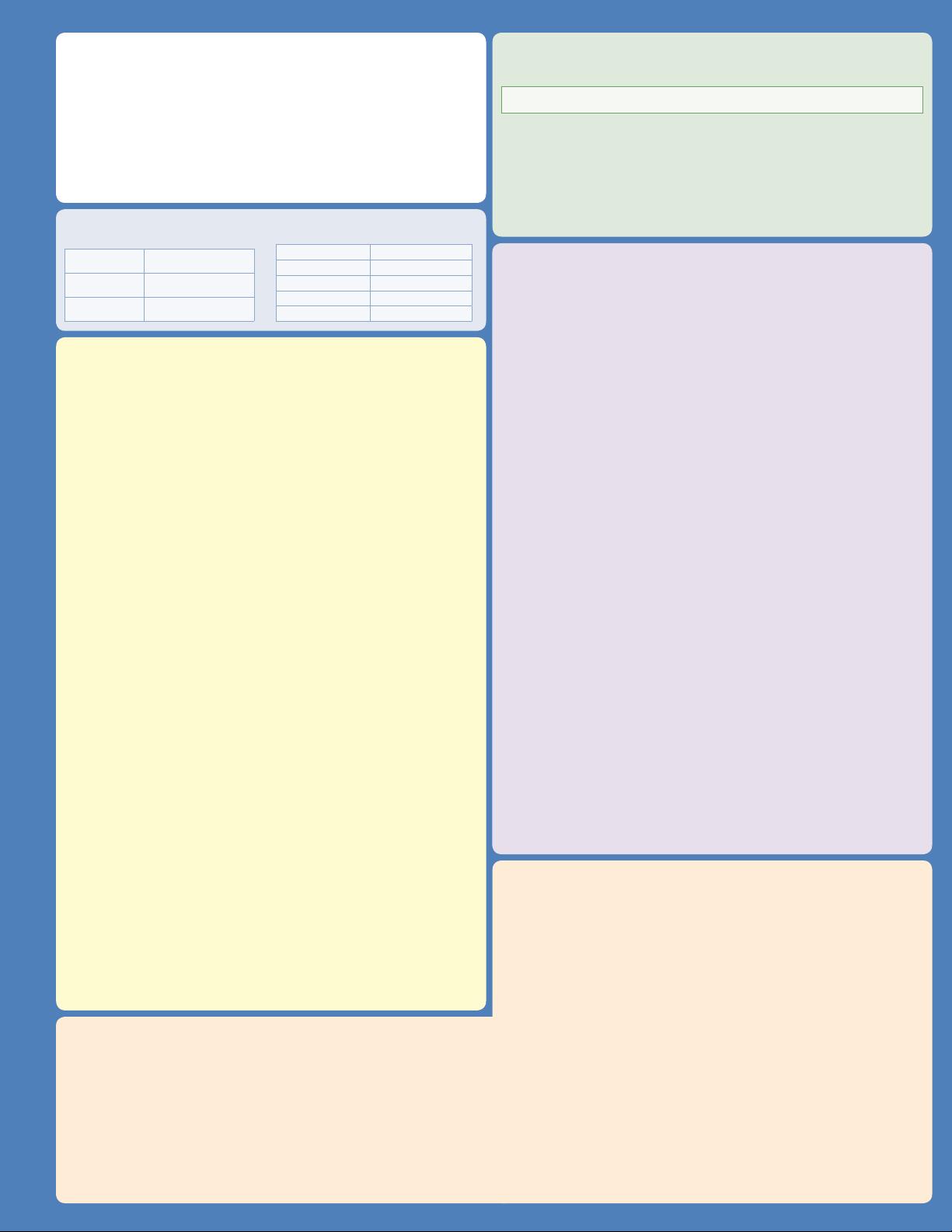
www.opengl.org/registry ©2010 Khronos Group - Rev. 0210
OpenGL 4.00 API Quick Reference Card
Vertex Arrays [2.8]
Vertex data may be placed into arrays stored in
the client address space or server address space.
void VertexPointer(int size, enum type,
sizei stride, void *pointer);
type: SHORT, INT, FLOAT, HALF_FLOAT, DOUBLE,
INT_2_10_10_10_REV,
UNSIGNED_INT_2_10_10_10_REV
void NormalPointer(enum type, sizei stride,
void *pointer);
type: see VertexPointer, plus BYTE
void ColorPointer(int size, enum type,
sizei stride, void *pointer);
type: see VertexPointer, plus BYTE, UBYTE, USHORT, UINT
void SecondaryColorPointer(int size,
enum type, sizei stride, void *pointer);
type: see ColorPointer
void IndexPointer(enum type, sizei stride,
void *pointer);
type: UBYTE, SHORT, INT, FLOAT, DOUBLE
void EdgeFlagPointer(sizei stride,
void *pointer);
void FogCoordPointer(enum type,
sizei stride, void *pointer);
type: FLOAT, HALF_FLOAT, DOUBLE
void TexCoordPointer(int size, enum type,
sizei stride, void *pointer);
type: see VertexPointer
void VertexAribPointer(uint index, int size,
enum type, boolean normalized,
sizei stride, const void *pointer);
type: see ColorPointer
void VertexAribIPointer(uint index,
int size, enum type, sizei stride,
const void *pointer);
type: BYTE, UBYTE, SHORT, USHORT, INT, UINT
index: [0, MAX_VERTEX_ATTRIBS - 1]
void EnableClientState(enum array);
void DisableClientState(enum array);
array: VERTEX_ARRAY, NORMAL_ARRAY,
COLOR_ARRAY, SECONDARY_COLOR_ARRAY,
INDEX_ARRAY, EDGE_FLAG_ARRAY,
FOG_COORD_ARRAY, TEXTURE_COORD_ARRAY
void EnableVertexAribArray(uint index);
void DisableVertexAribArray(uint index);
index: [0, MAX_VERTEX_ATTRIBS - 1]
void VertexAribDivisor(uint index,
uint divisor);
void ClientAcveTexture(enum texture);
index: TEXTUREi (where i is [0, MAX_TEXTURE_COORDS - 1])
void ArrayElement(int i);
Enable/Disable(PRIMITIVE_RESTART)
void PrimiveRestartIndex(uint index);
Drawing Commands [2.8.2] [2.8.3]
void DrawArrays(enum mode, int rst,
sizei count);
void DrawArraysInstanced(enum mode,
int rst, sizei count, sizei primcount};
void DrawArraysIndirect(enum mode,
const void *indirect);
void MulDrawArrays(enum mode,
int *rst, sizei *count, sizei primcount);
void DrawElements(enum mode,
sizei count, enum type, void *indices);
void DrawElementsInstanced(enum mode,
sizei count, enum type, const void *indices,
sizei primcount);
void MulDrawElements(enum mode,
sizei *count, enum type, void **indices,
sizei primcount);
void DrawRangeElements(enum mode,
uint start, uint end, sizei count,
enum type, void *indices);
void DrawElementsBaseVertex(enum mode,
sizei count, enum type, void *indices,
int basevertex);
void DrawRangeElementsBaseVertex(
enum mode, uint start, uint end,
sizei count, enum type, void *indices,
int basevertex);
void DrawElementsInstancedBaseVertex(
enum mode, sizei count, enum type,
const void *indices, sizei primcount,
int basevertex);
void DrawElementsIndirect(enum mode,
enum type, const void *indirect);
void MulDrawElementsBaseVertex(
enum mode, sizei *count, enum type,
void **indices, sizei primcount,
int *basevertex);
mode: POINTS,
LINE_STRIP, LINE_LOOP, LINES,
POLYGON, TRIANGLE_STRIP, TRIANGLE_FAN,
TRIANGLES, QUAD_STRIP, QUADS,
LINES_ADJACENCY, LINE_STRIP_ADJACENCY,
PATCHES, TRIANGLES_ADJACENCY,
TRIANGLE_STRIP_ADJACENCY
type: UNSIGNED_BYTE, UNSIGNED_SHORT,
UNSIGNED_INT
void InterleavedArrays(enum format,
sizei stride, void *pointer);
format: V2F, V3F, C4UB_V2F, C4UB_V3F, C3F_V3F,
N3F_V3F, C4F_N3F_V3F, T2F_V3F, T4F_V4F,
T2F_C4UB_V3F, T2F_C3F_V3F, T2F_N3F_V3F,
T2F_C4F_N3F_V3F, T4F_C4F_N3F_V4F
OpenGL
®
is the only cross-plaorm graphics API that enables developers of soware for
PC, workstaon, and supercompung hardware to create high-performance, visually-
compelling graphics soware applicaons, in markets such as CAD, content creaon,
energy, entertainment, game development, manufacturing, medical, and virtual reality.
Specicaons are available at www.opengl.org/registry
• see FunconName refers to funcons on this reference card.
• Content shown in blue is removed from the OpenGL 4.00 core prole and present only in the
OpenGL 4.00 compability prole. Prole selecon is made at context creaon.
• [n.n.n] and [Table n.n] refer to secons and tables in the OpenGL 4.00 core specicaon.
• [n.n.n] and [Table n.n] refer to secons and tables in the OpenGL 4.00 compability prole
specicaon, and are shown only when they dier from the core prole.
• [n.n.n] refers to secons in the OpenGL Shading Language 4.00 specicaon.
GL Command Syntax [2.3]
GL commands are formed from a return type, a name, and oponally up to 4 characters
(or character pairs) from the Command Leers table (above), as shown by the prototype below:
return-type Name{1234}{b s i i64 f d ub us ui ui64}{v} ([args ,] T arg1 , . . . , T argN [, args]);
The arguments enclosed in brackets ([args ,] and [, args]) may or may not be present.
The argument type T and the number N of arguments may be indicated by the command name
suxes. N is 1, 2, 3, or 4 if present, or else corresponds to the type leers from the Command
Table (above). If “v” is present, an array of N items are passed by a pointer.
For brevity, the OpenGL documentaon and this reference may omit the standard prexes.
The actual names are of the forms:
glFunconName(), GL_CONSTANT, GLtype
OpenGL Operaon
Floang-Point Numbers [2.1.1 - 2.1.4]
16-Bit 1-bit sign, 5-bit exponent,
10-bit manssa
Unsigned 11-Bit no sign bit, 5-bit exponent,
6-bit manssa
Unsigned 10-Bit no sign bit, 5-bit exponent,
5-bit manssa
Command Leers [Table 2.1]
Leers are used in commands to denote types.
b - byte (8 bits) ub - ubyte (8 bits)
s - short (16 bits) us - ushort (16 bits)
i - int (32 bits) ui - uint (32 bits)
i64 - int64 (64 bits) ui64 - uint64 (64 bits)
f - oat (32 bits) d - double (64 bits)
Buer Objects
[2.9]
void GenBuers(sizei n, uint *buers);
void DeleteBuers(sizei n, const uint *buers);
Creang and Binding Buer Objects[2.9.1]
void BindBuer(enum target, uint buer);
target: ARRAY_BUFFER, COPY_{READ, WRITE}_BUFFER,
DRAW_INDIRECT_BUFFER,
ELEMENT_ARRAY_BUFFER,
PIXEL_PACK_BUFFER, PIXEL_UNPACK_BUFFER,
TEXTURE_BUFFER, TRANSFORM_FEEDBACK_BUFFER,
UNIFORM_BUFFER
void BindBuerRange(enum target, uint index,
uint buer, intptr oset, sizeiptr size);
target: TRANSFORM_FEEDBACK_BUFFER,
UNIFORM_BUFFER
void BindBuerBase(enum target,
uint index, uint buer);
target: see BindBuerRange
Creang Buer Object Data Stores[2.9.
2]
void BuerData(enum target, sizeiptr size,
const void *data, enum usage);
usage: STREAM_{DRAW, READ, COPY},
STATIC_{DRAW, READ, COPY},
DYNAMIC_ {DRAW, READ, COPY}
target: see BindBuer
void BuerSubData(enum target,
intptr oset, sizeiptr size,
const void *data);
target: see BindBuer
Mapping/Unmapping Buer Data[2.9.3]
void *MapBuerRange(enum target,
intptr oset, sizeiptr length,
biield access);
access: The logical OR of MAP_{READ, WRITE}_BIT,
MAP_INVALIDATE_{BUFFER, RANGE}_BIT,
MAP_FLUSH_EXPLICIT_BIT,
MAP_UNSYNCHRONIZED_BIT
target: see BindBuer
void *MapBuer(enum target, enum access);
access: READ_ONLY, WRITE_ONLY, READ_WRITE
void FlushMappedBuerRange(
enum target, intptr oset, sizeiptr length);
target: see BindBuer
boolean UnmapBuer(enum target);
target: see BindBuer
Copying Between Buers [2.9.5]
void *CopyBuerSubData(enum readtarget,
enum writetarget, intptr readoset,
intptr writeoset, sizeiptr size);
readtarget and writetarget: see BindBuer
Vertex Array Objects [2.10]
All states related to denion of data used by
vertex processor is in a vertex array object.
void GenVertexArrays(sizei n, uint *arrays);
void DeleteVertexArrays(sizei n,
const uint *arrays);
void BindVertexArray(uint array);
Buer Object Queries [6.1.9] [6.1.15]
boolean IsBuer(uint buer);
void GetBuerParameteriv(enum target,
enum pname, int *data);
target: see BindBuer
pname: BUFFER_SIZE, BUFFER_USAGE,
BUFFER_ACCESS{_FLAGS}, BUFFER_MAPPED,
BUFFER_MAP_{OFFSET, LENGTH}
void GetBuerParameteri64v(enum target,
enum pname, int64 *data);
target: see BindBuer
pname: see GetBuerParameteriv,
void GetBuerSubData(enum target,
intptr oset, sizeiptr size, void *data);
target: see BindBuer
void GetBuerPointerv(enum target,
enum pname, void **params);
target: see BindBuer
pname: BUFFER_MAP_POINTER
Vertex Array Object Queries
[6.1.10] [6.1.16]
boolean IsVertexArray(uint array);
Vertex Specicaon
Begin and End [2.6]
Enclose coordinate sets between Begin/End
pairs to construct geometric objects.
void Begin(enum mode);
void End(void);
mode: see MulDrawElementsBaseVertex
Separate Patches
void PatchParameteri(enum pname, int value);
pname: PATCH_VERTICES
Polygon Edges [2.6.2]
Flag each edge of polygon primives as either
boundary or non-boundary.
void EdgeFlag(boolean ag);
void EdgeFlagv(boolean *ag);
Vertex Specicaon [2.7]
Verces have two, three, or four coordinates,
and oponally a current normal, mulple
current texture coordinate sets, mulple current
generic vertex aributes, current color, current
secondary color, and current fog coordinates.
void Vertex{234}{sifd}(T coords);
void Vertex{234}{sifd}v(T coords);
void VertexP{234}ui(enum type, uint coords)
void VertexP{234}uiv(enum type, uint *coords)
type: INT_2_10_10_10_REV,
UNSIGNED_INT_2_10_10_10_REV
void TexCoord{1234}{sifd}(T coords);
void TexCoord{1234}{sifd}v(T coords);
void TexCoordP{1234}ui(enum type,
uint coords)
void TexCoordP{1234}uiv(enum type,
uint *coords)
type: see VertexP{234}uiv
void MulTexCoord{1234}{sifd}(
enum texture, T coords)
void MulTexCoord{1234}{sifd}v(
enum texture, T coords)
texture: TEXTUREi (where i is
[0, MAX_TEXTURE_COORDS - 1])
void MulTexCoordP{1234}ui(enum texture,
enum type, uint coords)
void MulTexCoordP{1234}uiv(
enum texture, enum type, uint *coords)
void Normal3{bsifd}(T coords);
void Normal3{bsifd}v(T coords);
void NormalP3ui(enum type, uint normal)
void NormalP3uiv(enum type, uint *normal)
void FogCoord{fd}(T coord);
void FogCoord{fd}v(T coord);
void Color{34}{bsifd ubusui}(T components);
void Color{34}{bsifd ubusui}v(
T components);
void ColorP{34}ui(enum type, uint coords)
void ColorP{34}uiv(enum type, uint *coords)
void SecondaryColor3{bsifd ubusui}(
T components);
void SecondaryColor3{bsifd ubusui}v(
T components);
void SecondaryColorP3ui(enum type,
uint coords)
void SecondaryColorP3uiv(enum type,
uint *coords)
void Index{sifd ub}(T index);
void Index{sifd ub}v(T index);
void VertexArib{1234}{sfd}(uint index,
T values);
void VertexArib{123}{sfd}v(uint index,
T values);
void VertexArib4{bsifd ub us ui}v(
uint index, T values);
void VertexArib4Nub(uint index, T values);
void VertexArib4N{bsi ub us ui}v(
uint index, T values);
void VertexAribI{1234}{i ui}(uint index,
T values);
void VertexAribI{1234}{i ui}v(uint index,
T values);
void VertexAribI4{bs ub us}v(uint index,
T values);
void VertexAribP{1234}ui(
uint index, enum type, boolean normalized,
uint value)
void VertexAribP{1234}uiv(uint index,
enum type, boolean normalized, uint *value)
type: see VertexP{234}uiv