没有合适的资源?快使用搜索试试~ 我知道了~
首页Swift编程语言教程:革新iOS与OSX应用开发
Swift语言英文版是一本针对iOS和OS X应用程序开发的详尽教程,它是由苹果公司推出的新一代编程语言。Swift的设计旨在继承并改进C和Objective-C的优点,同时避免了C兼容性的限制。该语言强调安全编程模式,引入现代特性,如简洁的语法和更灵活的编程体验,旨在提升软件开发的效率和乐趣。
Swift的设计理念是重写编译器、调试器以及框架基础设施,以简化内存管理,通过自动引用计数(ARC)机制自动管理内存分配和释放,减轻了开发者的工作负担。其基础框架,建立在成熟的Foundation和Cocoa库之上,并且在整个框架栈中进行了现代化和标准化处理,使得Objective-C本身也得到了升级,支持了块(blocks)、集合字面量(collection literals)以及模块化(modules),这些新特性使框架能够无缝地接纳现代语言技术。
对于Objective-C开发者来说,Swift显得非常熟悉,它延续了Objective-C的可读性,并在此基础上加入了面向对象编程的现代特性。Swift的类、结构体、枚举、协议等概念易于理解,同时提供了更直观的错误处理和类型推断功能,降低了学习曲线。此外,Swift还引入了类型安全的泛型(Generics)和可选类型(Optional Types),帮助开发者编写更健壮的代码。
Swift的另一个亮点是交互式编程(Interactive Development),允许开发者在编码时即时看到结果,极大地提高了开发效率。Swift还支持函数式编程范式,提供了高阶函数(Higher-Order Functions)和闭包(Closure)等功能,为开发者提供了更多的编程风格选择。
本书将详细介绍Swift的关键特性,包括但不限于控制流、函数式编程、异常处理、并发编程、以及与Objective-C的互操作性。通过一系列实例和实战项目,读者将逐步掌握Swift编程的各个方面,并能够利用Swift构建高效、可维护的iOS和OS X应用。
Swift语言英文版是一本全面的指南,适合希望学习和转型到Swift编程的开发者,无论是初学者还是经验丰富的工程师,都能从中受益匪浅。通过学习Swift,开发者可以站在更高的层次上推动苹果软件开发的未来。
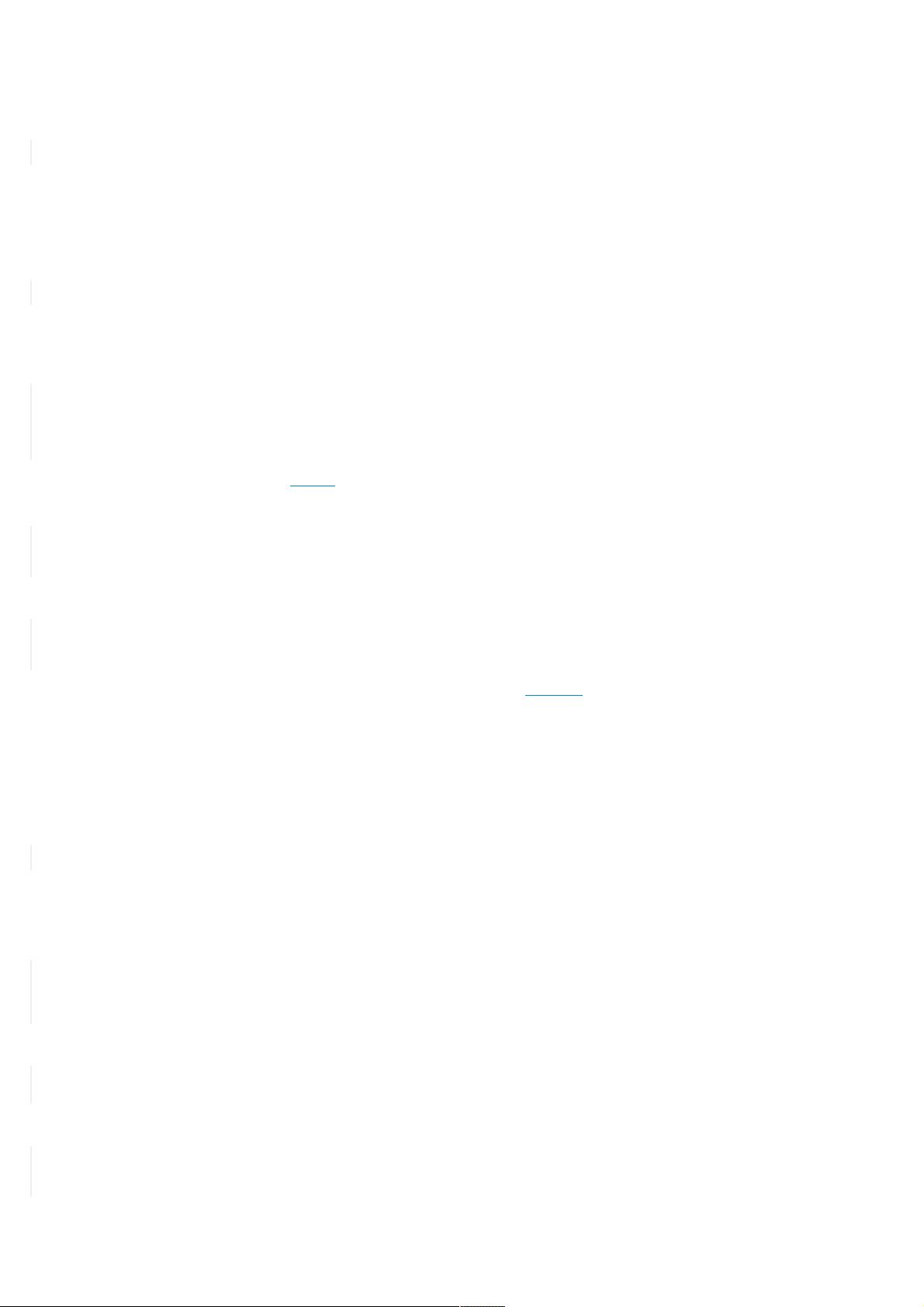
Type Aliases
Type aliases
define an alternative name for an existing type. You define type aliases with the typealias keyword.
Type aliases are useful when you want to refer to an existing type by a name that is contextually more appropriate, such as when working with data of a specific size from an external source:
typealias AudioSample = UInt16
Once you define a type alias, you can use the alias anywhere you might use the original name:
1 v ar maxAmplitudeFound = AudioSample.min
2 // maxAmplitudeFound is now 0
Here, AudioSample is defined as an alias for UInt16. Because it is an alias, the call to AudioSample.min actually calls UInt16.min, which provides an initial value of 0 for the maxAmplitudeFound variable.
Booleans
Swift has a basic
Boolean
type, called Bool. Boolean values are referred to as
logical
, because they can only ever be true or false. Swift provides two Boolean constant values, true and false:
1 let orangesAreOrange = true
2 let turnipsAreDelicious = false
The types of orangesAreO range and turnipsAreDelicious have been inferred as Bool from the fact that they were initialized with Boolean literal values. As with Int and Double above, you don’t need to declare constants or variables as Bool if you set
them to true or false as soon as you create them. Type inference helps make Swift code more concise and readable when it initializes constants or variables with other values whose type is already known.
Boolean values are particularly useful when you work with conditional statements such as the if statement:
1 if turnipsAreDelicious {
2 println("Mmm, tasty turnips!")
3 } else {
4 println("Eww, turnips are horrible.")
5 }
6 // prints "Eww, turnips are horrible."
Conditional statements such as the if statement are covered in more detail in Control Flow.
Swift’s type safety prevents non-Boolean values from being be substituted for Bool. The following example reports a compile-time error:
1 let i = 1
2 if i {
3 // this example will not compile, and will report an error
4 }
However, the alternative example below is valid:
1 let i = 1
2 if i == 1 {
3 // this example will compile successfully
4 }
The result of the i == 1 comparison is of type Bool, and so this second example passes the type-check. Comparisons like i == 1 are discussed in Basic Operators.
As with other examples of type safety in Swift, this approach avoids accidental errors and ensures that the intention of a particular section of code is always clear.
Tuples
Tuples
group multiple values into a single compound value. The values within a tuple can be of any type and do not have to be of the same type as each other.
In this example, (404, "Not Found") is a tuple that describes an
HTTP status code
. An HTTP status code is a special value returned by a web server whenever you request a web page. A status code of 404 Not Found is returned if you request a
webpage that doesn’t exist.
1 let http404Error = (404, "Not Found")
2 // http404Error is of type (Int, String), and equals (404, "Not Found")
The (404, "Not Found") tuple groups together an Int and a String to give the HTTP status code two separate values: a number and a human-readable description. It can be described as “a tuple of type (Int, String)”.
You can create tuples from any permutation of types, and they can contain as many different types as you like. There’s nothing stopping you from having a tuple of type (Int, Int, Int), or (String, Bool), or indeed any other permutation you require.
You can
decompose
a tuple’s contents into separate constants or variables, which you then access as usual:
1 let (statusCode, statusMessage) = http404Error
2 println("The status code is \(statusCode)")
3 // prints "The status code is 404"
4 println("The status message is \(statusMessage)")
5 // prints "The status message is Not Found"
If you only need some of the tuple’s values, ignore parts of the tuple with an underscore (_) when you decompose the tuple:
1 let (justTheStatusCode, _) = http404Error
2 println("The status code is \(justTheStatusCode)")
3 // prints "The status code is 404"
Alternatively, access the individual element values in a tuple using index numbers starting at zero:
1 println("The status code is \(http404Error.0)")
2 // prints "The status code is 404"
3 println("The status message is \(http404Error.1)")
4 // prints "The status message is Not Found"
You can name the individual elements in a tuple when the tuple is defined:
let http200Status = (statusCode: 200, description: "O K")
If you name the elements in a tuple, you can use the element names to access the values of those elements:
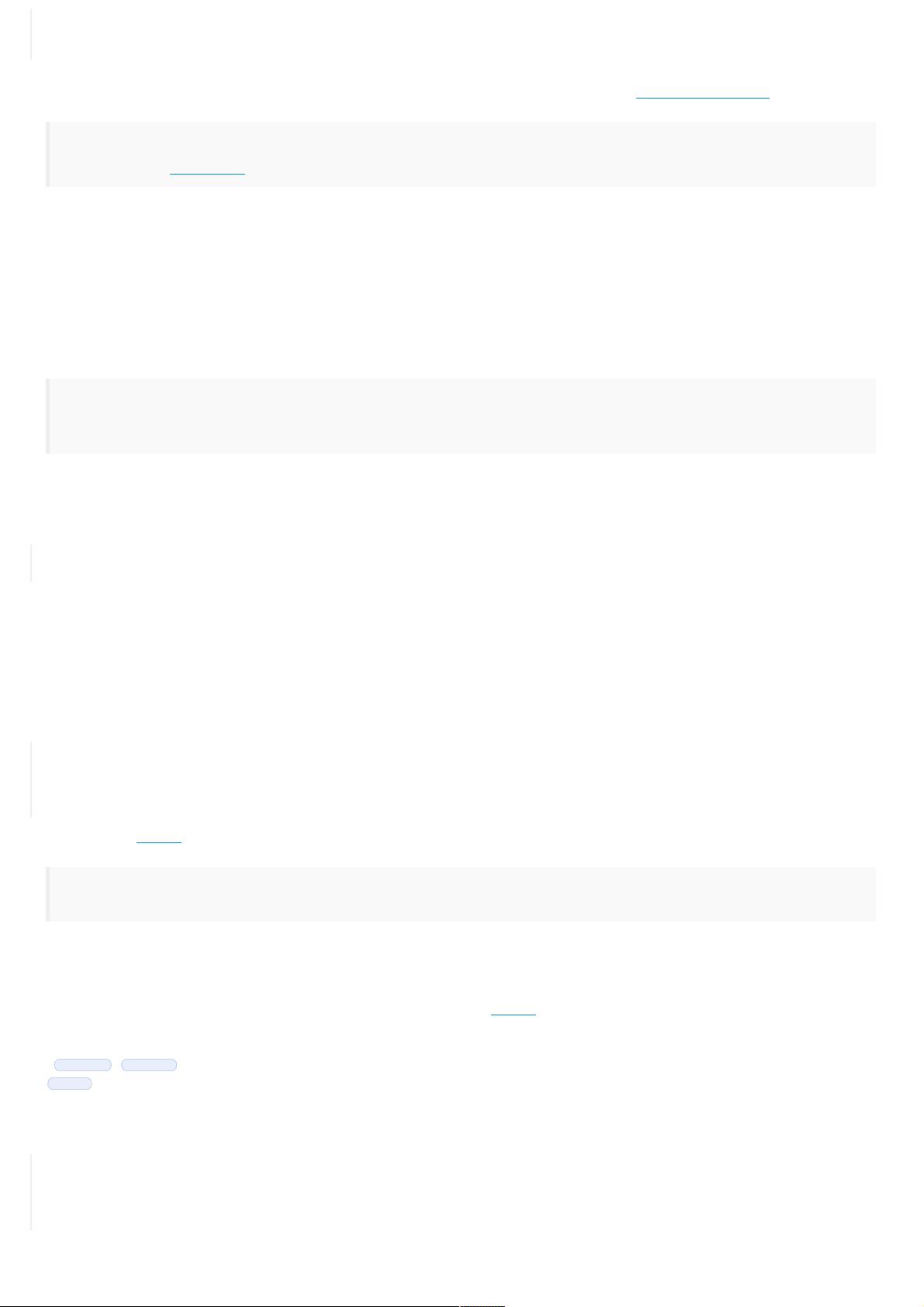
1 println("The status code is \(http200Status.statusCode)")
2 // prints "The status code is 200"
3 println("The status message is \(http200Status.description)")
4 // prints "The status message is OK"
Tuples are particularly useful as the return values of functions. A function that tries to retrieve a web page might return the (Int, String) tuple type to describe the success or failure of the page retrieval. By returning a tuple with two distinct values,
each of a different type, the function provides more useful information about its outcome than if it could only return a single value of a single type. For more information, see Functions with Multiple Return Values.
N O T E
Tuples are useful for temporary groups of related v alues. They are not suited to the creation of complex data structures. If your data structure is likely to persist beyond a temporary scope, model it as a class or structure, rather than as a
tuple. For more information, see Classes and Structures.
Optionals
You use
optionals
in situations where a value may be absent. An optional says:
or
N O T E
The concept of optionals doesn’t exist in C or O bjective-C. The nearest thing in O bjective-C is the ability to return nil from a method that would otherwise return an object, with nil meaning “the absence of a valid object.” However, this only
works for objects—it doesn’t work for structs, basic C types, or enumeration v alues. For these types, Objective-C methods typically return a special value (such as NSNotF ound) to indicate the absence of a value. This approach assumes that
the method’s caller knows there is a special value to test against and remembers to check for it. Swift’s optionals let you indicate the absence of a value for
any type at all
, without the need for special constants.
Here’s an example. Swift’s String type has a method called toInt, which tries to convert a String value into an Int value. However, not every string can be converted into an integer. The string "123" can be converted into the numeric value 123, but the
string "hello, world" does not have an obvious numeric value to convert to.
The example below uses the toInt method to try to convert a String into an Int:
1 let possibleNumber = "123"
2 let convertedNumber = possibleNumber.toInt()
3 // convertedNumber is inferred to be of type "Int?", or "optional Int"
Because the toInt method might fail, it returns an
optional
Int, rather than an Int. An optional Int is written as Int?, not Int. The question mark indicates that the value it contains is optional, meaning that it might contain
some
Int value, or it might
contain
no value at all
. (It can’t contain anything else, such as a Bool value or a String value. It’s either an Int, or it’s nothing at all.)
If Statements and Forced Unwrapping
You can use an if statement to find out whether an optional contains a value. If an optional does have a value, it evaluates to true; if it has no value at all, it evaluates to false.
Once you’re sure that the optional
does
contain a value, you can access its underlying value by adding an exclamation mark (!) to the end of the optional’s name. The exclamation mark effectively says, “I know that this optional definitely has a value;
please use it.” This is known as
forced unwrapping
of the optional’s value:
1 if conv ertedNumber {
2 println("\(possibleNumber) has an integer value of \(conv ertedNumber!)")
3 } else {
4 println("\(possibleNumber) could not be conv erted to an integer")
5 }
6 // prints "123 has an integer v alue of 123"
For more on the if statement, see Control Flow.
N O T E
Trying to use ! to access a non-existent optional value triggers a runtime error. Alway s make sure that an optional contains a non-nil value before using ! to force-unwrap its value.
Opt ional Binding
You use
optional binding
to find out whether an optional contains a value, and if so, to make that value available as a temporary constant or variable. Optional binding can be used with if and while statements to check for a value inside an optional,
and to extract that value into a constant or variable, as part of a single action. if and while statements are described in more detail in Control Flow.
Write optional bindings for the if statement as follows:
if let constantName = someOptional {
statements
}
You can rewrite the possibleNumber example from above to use optional binding rather than forced unwrapping:
1 if let actualNumber = possibleNumber.toInt() {
2 println("\(possibleNumber) has an integer value of \(actualNumber)")
3 } else {
4 println("\(possibleNumber) could not be conv erted to an integer")
5 }
6 // prints "123 has an integer v alue of 123"
This can be read as:
“If the optional Int returned by possibleNumber.toInt contains a value, set a new constant called actualNumber to the value contained in the optional.”
There
is
a value, and it equals
x
•
There
isn’t
a value at all•
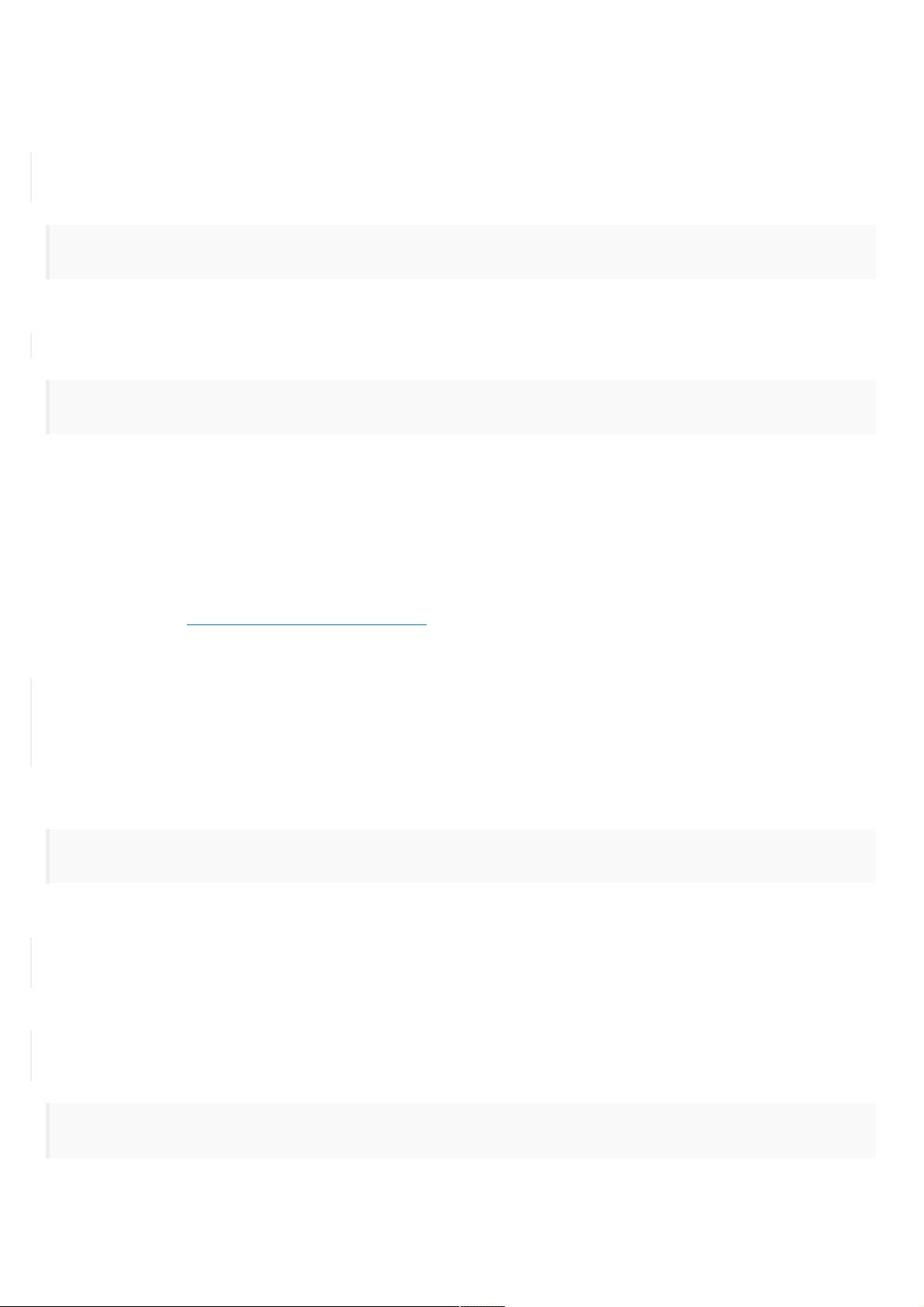
If the conversion is successful, the actualNumber constant becomes available for use within the first branch of the if statement. It has already been initialized with the value contained
within
the optional, and so there is no need to use the ! suffix to
access its value. In this example, actualNumber is simply used to print the result of the conversion.
You can use both constants and variables with optional binding. If you wanted to manipulate the value of actualNumber within the first branch of the if statement, you could write if var actualNumber instead, and the value contained within the optional
would be made available as a variable rather than a constant.
nil
You set an optional variable to a valueless state by assigning it the special value nil:
1 v ar serv erResponseCode: Int? = 404
2 // serverResponseCode contains an actual Int value of 404
3 serv erResponseCode = nil
4 // serverResponseCode now contains no v alue
N O T E
nil cannot be used with non-optional constants and v ariables. If a constant or v ariable in your code needs to be able to cope with the absence of a value under certain conditions, alway s declare it as an optional value of the appropriate type.
If you define an optional constant or variable without providing a default value, the constant or variable is automatically set to nil for you:
1 v ar surv eyA nswer: String?
2 // surveyA nswer is automatically set to nil
N O T E
Swift’s nil is not the same as nil in Objective-C . In O bjective-C, nil is a pointer to a non-existent object. In Swift, nil is not a pointer—it is the absence of a value of a certain type. Optionals of
any
type can be set to nil, not just object types.
Implicitly Unwrapped Opt ionals
As described above, optionals indicate that a constant or variable is allowed to have “no value”. Optionals can be checked with an if statement to see if a value exists, and can be conditionally unwrapped with optional binding to access the optional’s
value if it does exist.
Sometimes it is clear from a program’s structure that an optional will
always
have a value, after that value is first set. In these cases, it is useful to remove the need to check and unwrap the optional’s value every time it is accessed, because it can be
safely assumed to have a value all of the time.
These kinds of optionals are defined as
implicitly unwrapped optionals
. You write an implicitly unwrapped optional by placing an exclamation mark (String!) rather than a question mark (String?) after the type that you want to make optional.
Implicitly unwrapped optionals are useful when an optional’s value is confirmed to exist immediately after the optional is first defined and can definitely be assumed to exist at every point thereafter. The primary use of implicitly unwrapped optionals in
Swift is during class initialization, as described in Unowned References and Implicitly Unwrapped Optional Properties.
An implicitly unwrapped optional is a normal optional behind the scenes, but can also be used like a nonoptional value, without the need to unwrap the optional value each time it is accessed. The following example shows the difference in behavior
between an optional String and an implicitly unwrapped optional String:
1 let possibleString: String? = "An optional string."
2 println(possibleString!) // requires an exclamation mark to access its value
3 // prints "An optional string."
4
5 let assumedString: String! = "An implicitly unwrapped optional string."
6 println(assumedString) // no exclamation mark is needed to access its value
7 // prints "An implicitly unwrapped optional string."
You can think of an implicitly unwrapped optional as giving permission for the optional to be unwrapped automatically whenever it is used. Rather than placing an exclamation mark after the optional’s name each time you use it, you place an
exclamation mark after the optional’s type when you declare it.
N O T E
If you try to access an implicitly unwrapped optional when it does not contain a value, you will trigger a runtime error. The result is exactly the same as if you place an exclamation mark after a normal optional that does not contain a value.
You can still treat an implicitly unwrapped optional like a normal optional, to check if it contains a value:
1 if assumedString {
2 println(assumedString)
3 }
4 // prints "An implicitly unwrapped optional string."
You can also use an implicitly unwrapped optional with optional binding, to check and unwrap its value in a single statement:
1 if let definiteString = assumedString {
2 println(definiteString)
3 }
4 // prints "An implicitly unwrapped optional string."
N O T E
Implicitly unwrapped optionals should not be used when there is a possibility of a variable becoming nil at a later point. Always use a normal optional type if you need to check for a nil value during the lifetime of a variable.
Assertions
Optionals enable you to check for values that may or may not exist, and to write code that copes gracefully with the absence of a value. In some cases, however, it is simply not possible for your code to continue execution if a value does not exist,
or if a provided value does not satisfy certain conditions. In these situations, you can trigger an
assertion
in your code to end code execution and to provide an opportunity to debug the cause of the absent or invalid value.
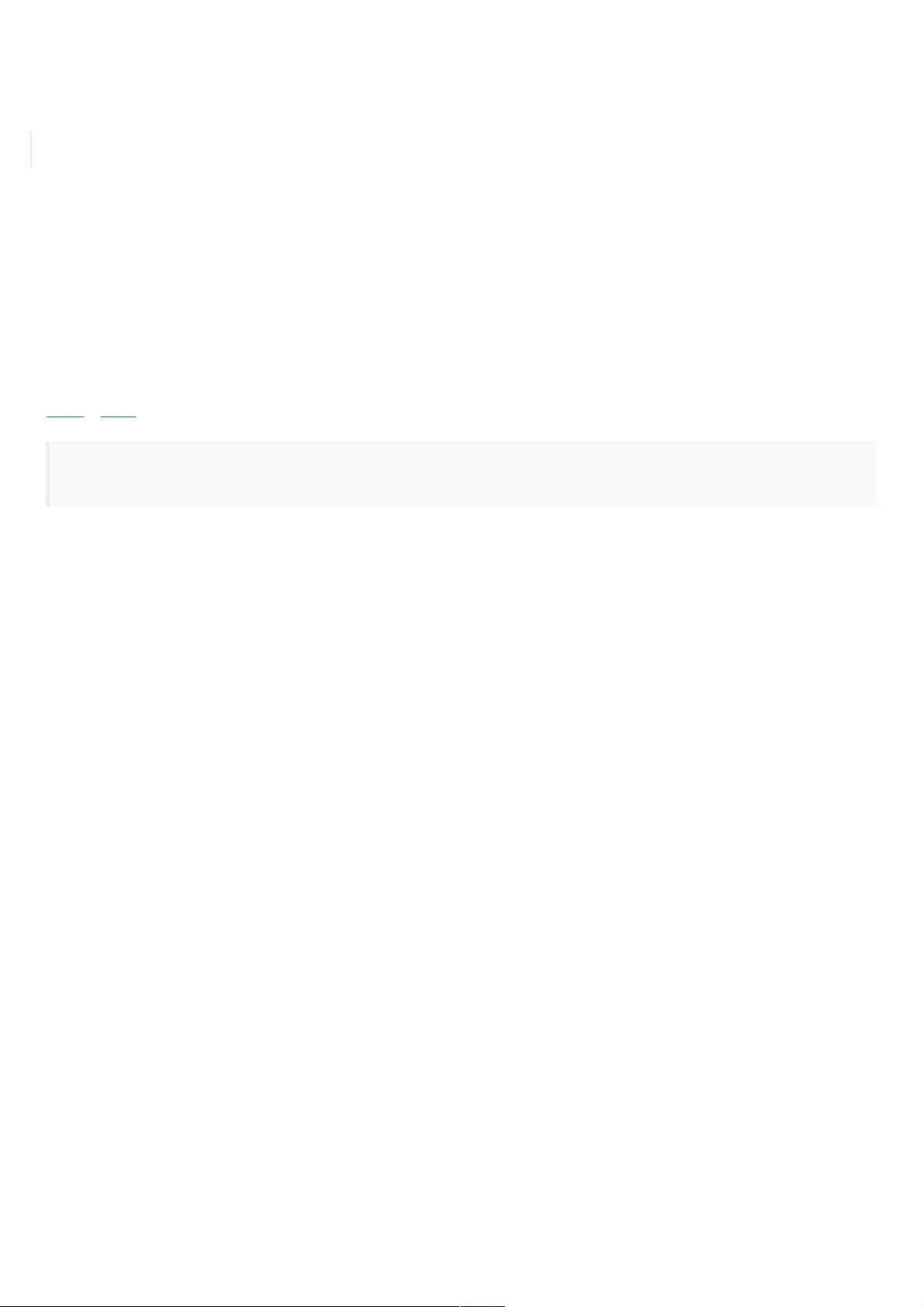
Debugging with A ssertions
An assertion is a runtime check that a logical condition definitely evaluates to true. Literally put, an assertion “asserts” that a condition is true. You use an assertion to make sure that an essential condition is satisfied before executing any further
code. If the condition evaluates to true, code execution continues as usual; if the condition evaluates to false, code execution ends, and your app is terminated.
If your code triggers an assertion while running in a debug environment, such as when you build and run an app in Xcode, you can see exactly where the invalid state occurred and query the state of your app at the time that the assertion was
triggered. An assertion also lets you provide a suitable debug message as to the nature of the assert.
You write an assertion by calling the global assert function. You pass the assert function an expression that evaluates to true or false and a message that should be displayed if the result of the condition is false:
1 let age = -3
2 assert(age >= 0, "A person's age cannot be less than zero")
3 // this causes the assertion to trigger, because age is not >= 0
In this example, code execution will continue only if age >= 0 evaluates to true, that is, if the value of age is non-negative. If the value of age
is
negative, as in the code above, then age >= 0 evaluates to false, and the assertion is triggered,
terminating the application.
Assertion messages cannot use string interpolation. The assertion message can be omitted if desired, as in the following example:
assert(age >= 0)
When t o Use A ssertions
Use an assertion whenever a condition has the potential to be false, but must
definitely
be true in order for your code to continue execution. Suitable scenarios for an assertion check include:
See also Subscripts and Functions.
N O T E
Assertions cause your app to terminate and are not a substitute for designing y our code in such a way that inv alid conditions are unlikely to arise. Nonetheless, in situations where inv alid conditions are possible, an assertion is an effective way
to ensure that such conditions are highlighted and noticed during development, before y our app is published.
An integer subscript index is passed to a custom subscript implementation, but the subscript index value could be too low or too high.•
A value is passed to a function, but an invalid value means that the function cannot fulfill its task.•
An optional value is currently nil, but a non-nil value is essential for subsequent code to execute successfully.•
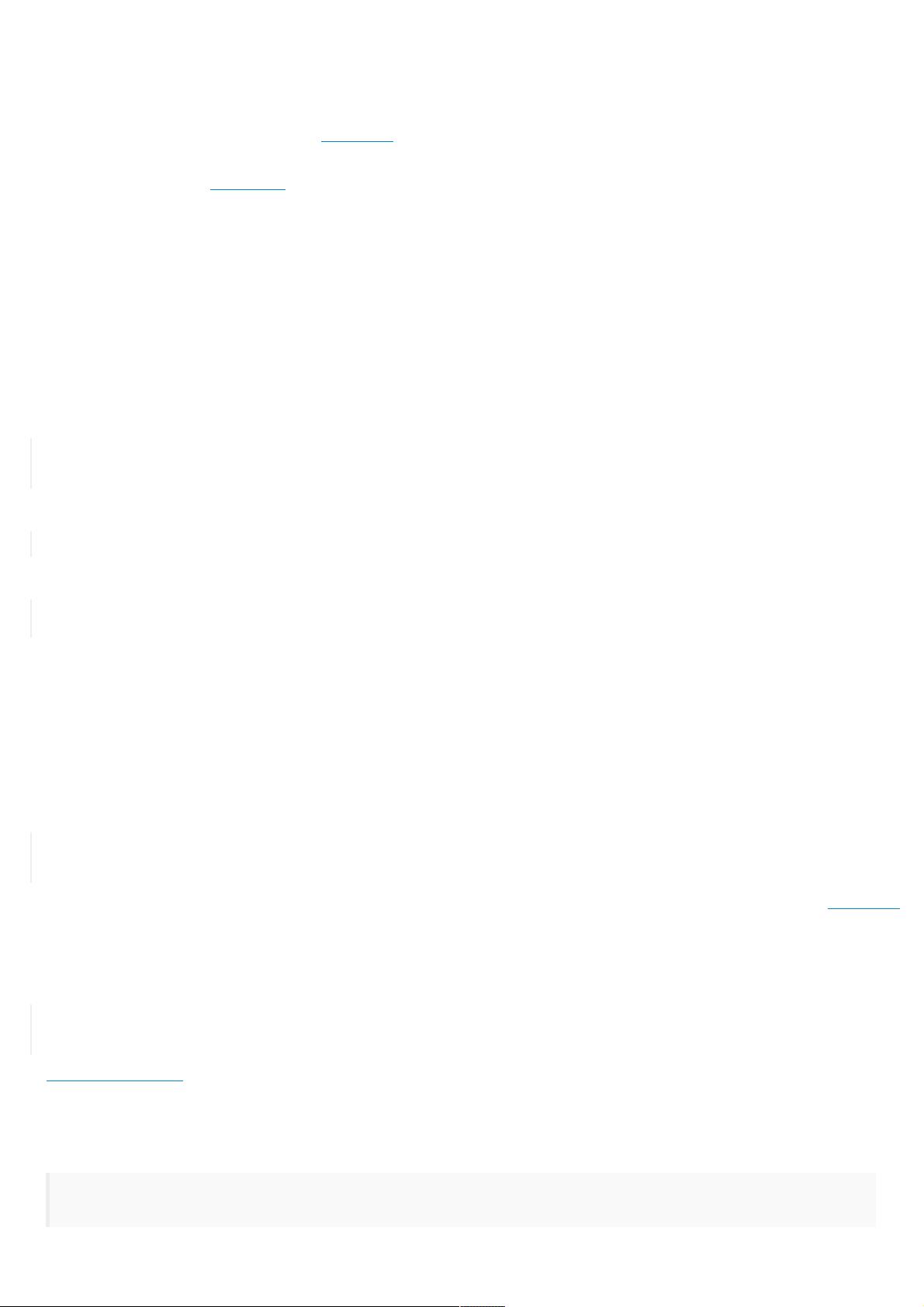
Basic Operators
An
operator
is a special symbol or phrase that you use to check, change, or combine values. For example, the addition operator (+) adds two numbers together (as in let i = 1 + 2). More complex examples include the logical AND operator && (as in if
enteredDoorC ode && passedRetinaScan) and the increment operator ++i, which is a shortcut to increase the value of i by 1.
Swift supports most standard C operators and improves several capabilities to eliminate common coding errors. The assignment operator (=) does not return a value, to prevent it from being mistakenly used when the equal to operator (==) is
intended. Arithmetic operators (+, -, *, /, % and so forth) detect and disallow value overflow, to avoid unexpected results when working with numbers that become larger or smaller than the allowed value range of the type that stores them. You can
opt in to value overflow behavior by using Swift’s overflow operators, as described in Overflow Operators.
Unlike C, Swift lets you perform remainder (%) calculations on floating-point numbers. Swift also provides two range operators (a..b and a...b) not found in C, as a shortcut for expressing a range of values.
This chapter describes the common operators in Swift. Advanced Operators covers Swift’s advanced operators, and describes how to define your own custom operators and implement the standard operators for your own custom types.
Terminology
Operators are unary, binary, or ternary:
The values that operators affect are
operands
. In the expression 1 + 2, the + symbol is a binary operator and its two operands are the values 1 and 2.
Assignment Operator
The
assignment operator
(a = b) initializes or updates the value of a with the value of b:
1 let b = 10
2 v ar a = 5
3 a = b
4 // a is now equal to 10
If the right side of the assignment is a tuple with multiple values, its elements can be decomposed into multiple constants or variables at once:
1 let (x, y) = (1, 2)
2 // x is equal to 1, and y is equal to 2
Unlike the assignment operator in C and Objective-C, the assignment operator in Swift does not itself return a value. The following statement is not valid:
1 if x = y {
2 // this is not valid, because x = y does not return a value
3 }
This feature prevents the assignment operator (=) from being used by accident when the equal to operator (==) is actually intended. By making if x = y invalid, Swift helps you to avoid these kinds of errors in your code.
Arithmetic Operators
Swift supports the four standard
arithmetic operators
for all number types:
1 1 + 2 // equals 3
2 5 - 3 // equals 2
3 2 * 3 // equals 6
4 10.0 / 2.5 // equals 4.0
Unlike the arithmetic operators in C and Objective-C, the Swift arithmetic operators do not allow values to overflow by default. You can opt in to value overflow behavior by using Swift’s overflow operators (such as a &+ b). See Overflow Operators.
The addition operator is also supported for String concatenation:
"hello, " + "world" // equals "hello, world"
Two Character values, or one Character value and one String value, can be added together to make a new String value:
1 let dog: Character = ""
2 let cow: Character = ""
3 let dogCow = dog + cow
4 // dogCow is equal to ""
See also Concatenating Strings and Characters.
Remainder Operator
The
remainder operator
(a % b) works out how many multiples of b will fit inside a and returns the value that is left over (known as the
remainder
).
N O T E
The remainder operator (% ) is also known as a
modulo operator
in other languages. However, its behavior in Swift for negative numbers means that it is, strictly speaking, a remainder rather than a modulo operation.
Here’s how the remainder operator works. To calculate 9 % 4, you first work out how many 4s will fit inside 9:
Unary
operators operate on a single target (such as -a). Unary
prefix
operators appear immediately before their target (such as !b), and unary
postfix
operators appear immediately after their target (such as i++).•
Binary
operators operate on two targets (such as 2 + 3) and are
infix
because they appear in between their two targets.•
Ternary
operators operate on three targets. Like C, Swift has only one ternary operator, the ternary conditional operator (a ? b : c).•
Addition (+)•
Subtraction (-)•
Multiplication (*)•
Division (/)•
剩余168页未读,继续阅读
点击了解资源详情
206 浏览量
点击了解资源详情
2014-06-06 上传
2014-06-13 上传
123 浏览量
231 浏览量
376 浏览量
2024-05-07 上传

龙孩儿
- 粉丝: 9
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 微波网络分析仪详解:概念、参数与测量
- 从Windows到Linux:一个UNIX爱好者的心路历程
- 经典Bash shell教程:深入学习与实践
- .NET平台入门教程:C#编程精髓
- 深入解析Linux 0.11内核源代码详解
- MyEclipse + Struts + Hibernate:初学者快速配置指南
- 探索WPF/E:跨平台富互联网应用开发入门
- Java基础:递归、过滤器与I/O流详解
- LoadRunner入门教程:自动化压力测试实践
- Java程序员挑战指南:BITSCorporation课程
- 粒子群优化在自适应均衡算法中的应用
- 改进LMS算法在OFDM系统中的信道均衡应用
- Ajax技术解析:开启Web设计新篇章
- Oracle10gR2在AIX5L上的安装教程
- SD卡工作原理与驱动详解
- 基于IIS总线的嵌入式音频系统详解与Linux驱动开发
安全验证
文档复制为VIP权益,开通VIP直接复制
