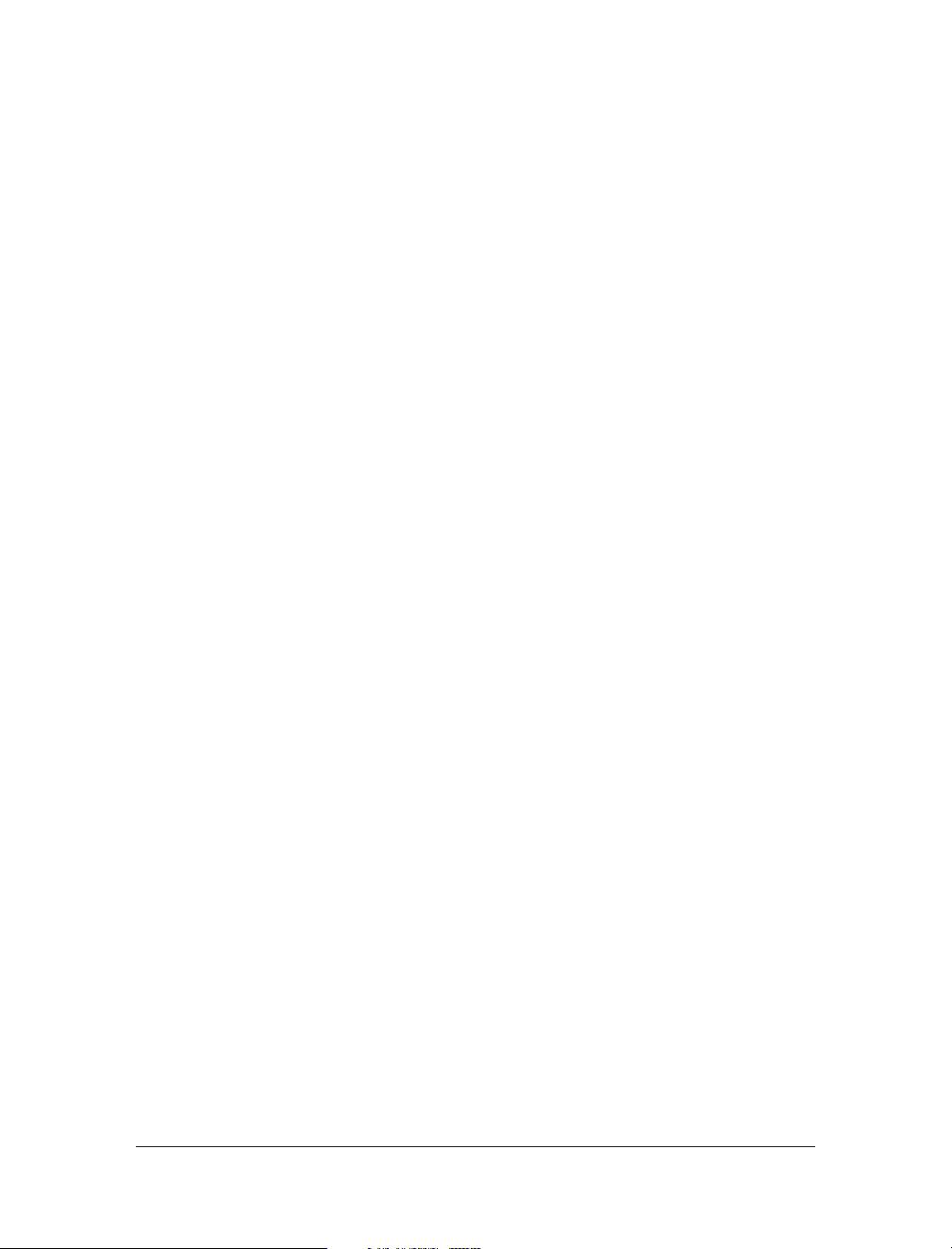
The next two chapters throw some light on two parts of Java programming that are
critical to almost all network programs but are often misunderstood and misused: I/O
and threading. Chapter 2, Streams, explores Java’s classic I/O which—despite the new
I/O APIs—isn’t going away any time soon and is still the preferred means of handling
input and output in most client applications. Understanding how Java handles I/O in
the general case is a prerequisite for understanding the special case of how Java handles
network I/O. Chapter 3, Threads, explores multithreading and synchronization, with a
special emphasis on how they can be used for asynchronous I/O and network servers.
Experienced Java programmers may be able to skim or skip these two chapters. However,
Chapter 4, Internet Addresses, is essential reading for everyone. It shows how Java pro‐
grams interact with the Domain Name System through the InetAddress class, the one
class that’s needed by essentially all network programs. Once you’ve finished this chap‐
ter, it’s possible to jump around in the book as your interests and needs dictate.
Chapter 5, URLs and URIs, explores Java’s URL class, a powerful abstraction for down‐
loading information and files from network servers of many kinds. The URL class enables
you to connect to and download files and documents from a network server without
concerning yourself with the details of the protocol the server speaks. It lets you connect
to an FTP server using the same code you use to talk to an HTTP server or to read a file
on the local hard disk. You’ll also learn about the newer URI class, a more standards-
conformant alternative for identifying but not retrieving resources.
Chapter 6, HTTP, delves deeper into the HTTP protocol specifically. Topics covered
include REST, HTTP headers, and cookies. Chapter 7, URLConnections, shows you how
to use the URLConnection and HttpURLConnection classes not just to download data
from web servers, but to upload documents and configure connections.
Chapter 8 through Chapter 10 discuss Java’s low-level socket classes for network access.
Chapter 8, Sockets for Clients, introduces the Java sockets API and the Socket class in
particular. It shows you how to write network clients that interact with TCP servers of
all kinds including whois, dict, and HTTP. Chapter 9, Sockets for Servers, shows you
how to use the ServerSocket class to write servers for these and other protocols. Finally,
Chapter 10, Secure Sockets, shows you how to protect your client-server communica‐
tions using the Secure Sockets Layer (SSL) and the Java Secure Sockets Extension (JSSE).
Chapter 11, Nonblocking I/O, introduces the new I/O APIs specifically designed for
network servers. These APIs enable a program to figure out whether a connection is
ready before it tries to read from or write to the socket. This allows a single thread to
manage many different connections simultaneously, thereby placing much less load on
the virtual machine. The new I/O APIs don’t help much for small servers or clients that
don’t open many simultaneous connections, but they may provide performance boosts
for high-volume servers that want to transmit as much data as the network can handle
as fast as the network can deliver it.
Preface | xv