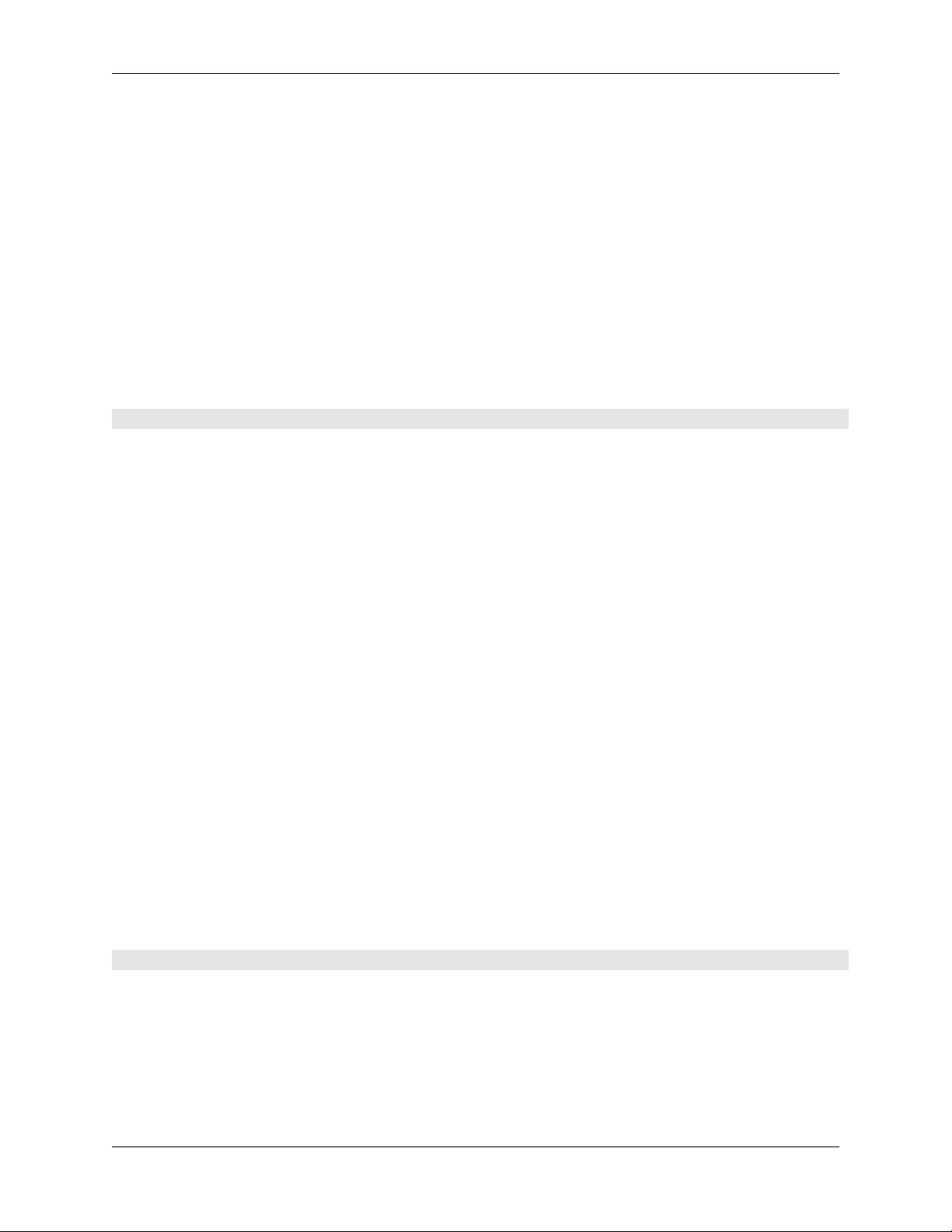
Django Documentation, Release 1.11.8.dev20171115160334
The philosophy here is that your site is edited by a staff, or a client, or maybe just you – and you don’t want to have to
deal with creating backend interfaces just to manage content.
One typical workflow in creating Django apps is to create models and get the admin sites up and running as fast as
possible, so your staff (or clients) can start populating data. Then, develop the way data is presented to the public.
2.1.5 Design your URLs
A clean, elegant URL scheme is an important detail in a high-quality Web application. Django encourages beautiful
URL design and doesn’t put any cruft in URLs, like .php or .asp.
To design URLs for an app, you create a Python module called a URLconf . A table of contents for your app, it contains
a simple mapping between URL patterns and Python callback functions. URLconfs also serve to decouple URLs from
Python code.
Here’s what a URLconf might look like for the Reporter/Article example above:
mysite/news/urls.py
from django.conf.urls import url
from . import views
urlpatterns = [
url(r'^articles/([0-9]{4})/$', views.year_archive),
url(r'^articles/([0-9]{4})/([0-9]{2})/$', views.month_archive),
url(r'^articles/([0-9]{4})/([0-9]{2})/([0-9]+)/$', views.article_detail),
]
The code above maps URLs, as simple regular expressions, to the location of Python callback functions (“views”).
The regular expressions use parenthesis to “capture” values from the URLs. When a user requests a page, Django runs
through each pattern, in order, and stops at the first one that matches the requested URL. (If none of them matches,
Django calls a special-case 404 view.) This is blazingly fast, because the regular expressions are compiled at load
time.
Once one of the regexes matches, Django calls the given view, which is a Python function. Each view gets passed a
request object – which contains request metadata – and the values captured in the regex.
For example, if a user requested the URL “/articles/2005/05/39323/”, Django would call the function news.views.
article_detail(request, '2005', '05', '39323').
2.1.6 Write your views
Each view is responsible for doing one of two things: Returning an HttpResponse object containing the content
for the requested page, or raising an exception such as Http404. The rest is up to you.
Generally, a view retrieves data according to the parameters, loads a template and renders the template with the
retrieved data. Here’s an example view for year_archive from above:
mysite/news/views.py
from django.shortcuts import render
from .models import Article
def year_archive(request, year):
a_list = Article.objects.filter(pub_date__year=year)
context = {'year': year, 'article_list': a_list}
return render(request, 'news/year_archive.html', context)
10 Chapter 2. Getting started