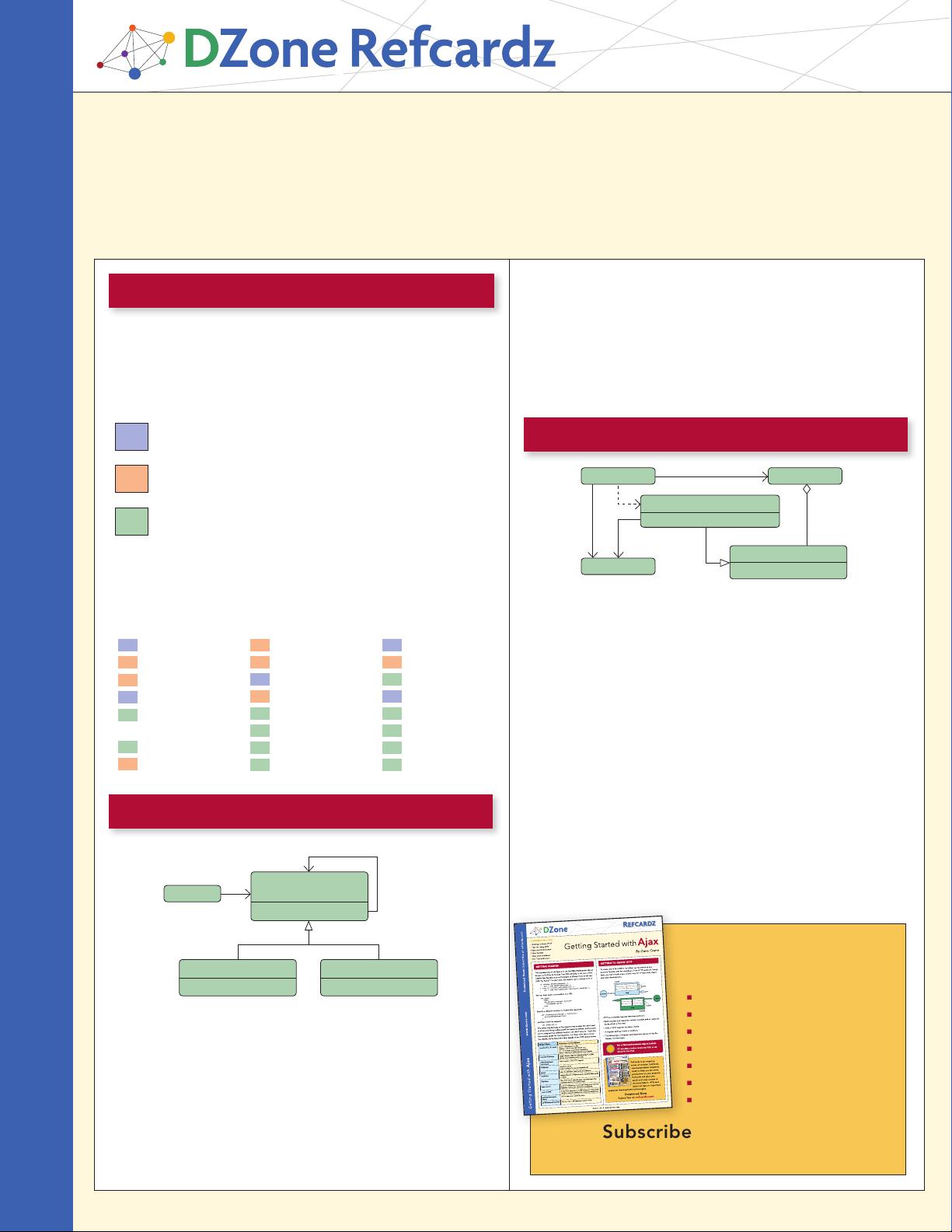
Design Patterns
By Jason McDonald
CONTENTS INCLUDE:
n
Chain of Responsibility
n
Command
n
Interpreter
n
Iterator
n
Mediator
n
Observer
n
Template Method and more...
DZone, Inc.
|
www.dzone.com
This Design Patterns refcard provides a quick reference to
the original 23 Gang of Four design patterns, as listed in the
book Design Patterns: Elements of Reusable Object-Oriented
Software. Each pattern includes class diagrams, explanation,
usage information, and a real world example.
Object Scope: Deals with object relationships that can be
changed at runtime.
Class Scope: Deals with class relationships that can be changed
at compile time.
C
Abstract Factory
S
Adapter
S
Bridge
C
Builder
B
Chain of
Responsibility
B
Command
S
Composite
S
Decorator
S
Facade
C
Factory Method
S
Flyweight
B
Interpreter
B
Iterator
B
Mediator
B
Memento
C
Prototype
S
Proxy
B
Observer
C
Singleton
B
State
B
Strategy
B
Template Method
B
Visitor
ABOUT DESIGN PATTERNS
n
Authoritative content
n
Designed for developers
n
Written by top experts
n
Latest tools & technologies
n
Hot tips & examples
n
Bonus content online
n
New issue every 1-2 weeks
Subscribe Now for FREE!
Refcardz.com
Get More Refcardz
(
They’re free!
)
tech facts at your fingertips
Method Name Parameters and Descriptions
open(method, url, async) open a connection to a URL
method = HTTP verb (GET, POST, etc.)
url = url to open, may include querystring
async = whether to make asynchronous request
onreadystatechange assign a function object as callback (similar to onclick,
onload, etc. in browser event model)
setRequestHeader
(namevalue)
add a header to the HTTP request
send(body) send the request
body = string to be used as request body
abort() stop the XHR from listening for the response
readyState stage in lifecycle of response (only populated after send()
is called)
httpStatus The HTTP return code (integer, only populated after
response reaches the loaded state)
responseText body of response as a JavaS cript string (only set aft er
response reaches the interact ive readyState)
responseXML body of the response as a XML document object (only
set after response reaches the interactive readyState)
getResponseHeader
(name)
read a response header by nam e
getAllResponseHeaders() Get an array of all response header names
Hot
Tip
tech facts at your fingert ips
Design Patterns www.dzone.com Get More Refcarz! Visit refcardz.com
Creational Patterns: Used to construct objects such that
they can be decoupled from their implementing system.
Structural Patterns: Used to form large object structures
between many disparate objects.
Behavioral Patterns: Used to manage algorithms,
relationships, and responsibilities between objects.
CHAIN OF RESPONSIBILITY
Object Behavioral
Purpose
Gives more than one object an opportunity to handle a request
by linking receiving objects together.
Use When
n
Multiple objects may handle a request and the handler
doesn’t have to be a specific object.
n
A set of objects should be able to handle a request with the
handler determined at runtime.
n
A request not being handled is an acceptable potential
outcome.
Example
Exception handling in some languages implements this pattern.
When an exception is thrown in a method the runtime checks to
see if the method has a mechanism to handle the exception or
if it should be passed up the call stack. When passed up the call
stack the process repeats until code to handle the exception is
encountered or until there are no more parent objects to hand
the request to.
COMMAND
Object Behavioral
Purpose
Encapsulates a request allowing it to be treated as an object.
This allows the request to be handled in traditionally object
based relationships such as queuing and callbacks.
Use When
n
You need callback functionality.
n
Requests need to be handled at variant times or in variant orders.
n
A history of requests is needed.
n
The invoker should be decoupled from the object handling the
invocation.
Example
Job queues are widely used to facilitate the asynchronous
processing of algorithms. By utilizing the command pattern the
functionality to be executed can be given to a job queue for
processing without any need for the queue to have knowledge
of the actual implementation it is invoking. The command object
that is enqueued implements its particular algorithm within the
confines of the interface the queue is expecting.
Receiver
Invoker
Command
+execute( )
Client
ConcreteCommand
+execute( )
successor
Client
<<interface>>
Handler
+handlerequest()
ConcreteHandler 1
+handlerequest()
ConcreteHandler 2
+handlerequest()
#8