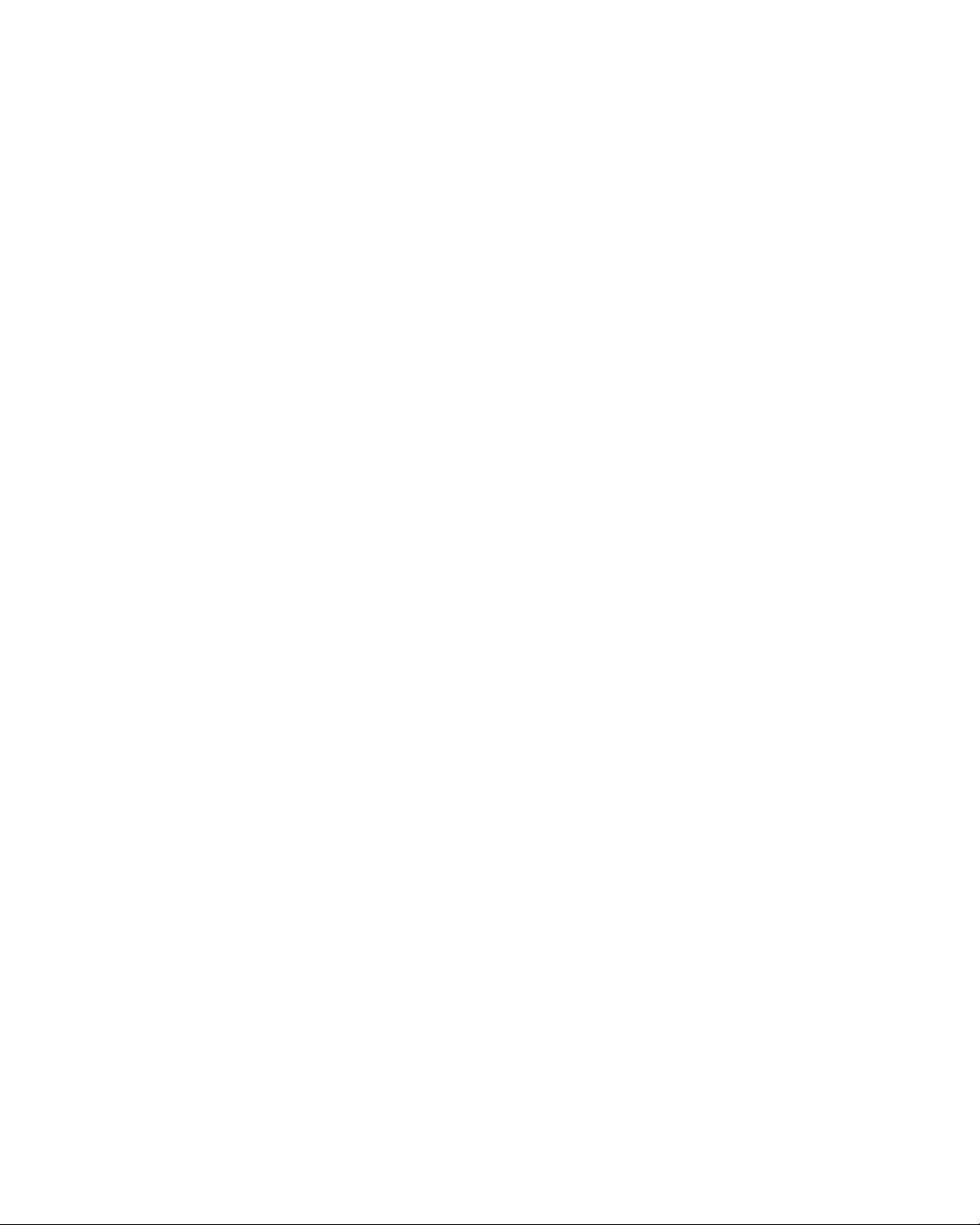
xvi Contents
Writing an Event Listener for the
KiloConverter
Class . . . . . . . . . . . . . .779
Background and Foreground Colors . . . . . . . . . . . . . . . . . . . . . . . . . . . .784
The
ActionEvent
Object . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .788
12.3 Layout Managers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 793
Adding a Layout Manager to a Container . . . . . . . . . . . . . . . . . . . . . . .794
The
FlowLayout
Manager . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .794
The
BorderLayout
Manager . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .797
The
GridLayout
Manager . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .804
12.4 Radio Buttons and Check Boxes . . . . . . . . . . . . . . . . . . . . . . . . . . . . 810
Radio Buttons . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .810
Check Boxes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .816
12.5 Borders . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 821
12.6 Focus on Problem Solving: Extending Classes from
JPanel
. . . . . . . . 824
The Brandi’s Bagel House Application. . . . . . . . . . . . . . . . . . . . . . . . . . .824
The
GreetingPanel
Class. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .825
The
BagelPanel
Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .826
The
ToppingPanel
Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .828
The
CoffeePanel
Class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .830
Putting It All Together . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .832
12.7 Splash Screens . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 836
12.8 Using Console Output to Debug a GUI Application . . . . . . . . . . . . . 837
12.9 Common Errors to Avoid . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 842
Review Questions and Exercises 842
Programming Challenges 845
Chapter 13 Advanced GUI Applications 849
13.1 The Swing and AWT Class Hierarchy . . . . . . . . . . . . . . . . . . . . . . . . 849
13.2 Read-Only Text Fields. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 850
13.3 Lists . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 852
Selection Modes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .852
Responding to List Events. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .853
Retrieving the Selected Item. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .854
Placing a Border around a List . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .858
Adding a Scroll Bar to a List . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .858
Adding Items to an Existing
JList
Component. . . . . . . . . . . . . . . . . . . .863
Multiple Selection Lists. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .863
13.4 Combo Boxes. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 868
Retrieving the Selected Item. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .869
13.5 Displaying Images in Labels and Buttons . . . . . . . . . . . . . . . . . . . . . 874
13.6 Mnemonics and Tool Tips . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 880
Mnemonics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .880
Tool Tips . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .882
13.7 File Choosers and Color Choosers . . . . . . . . . . . . . . . . . . . . . . . . . . 882
File Choosers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .883
Color Choosers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .885