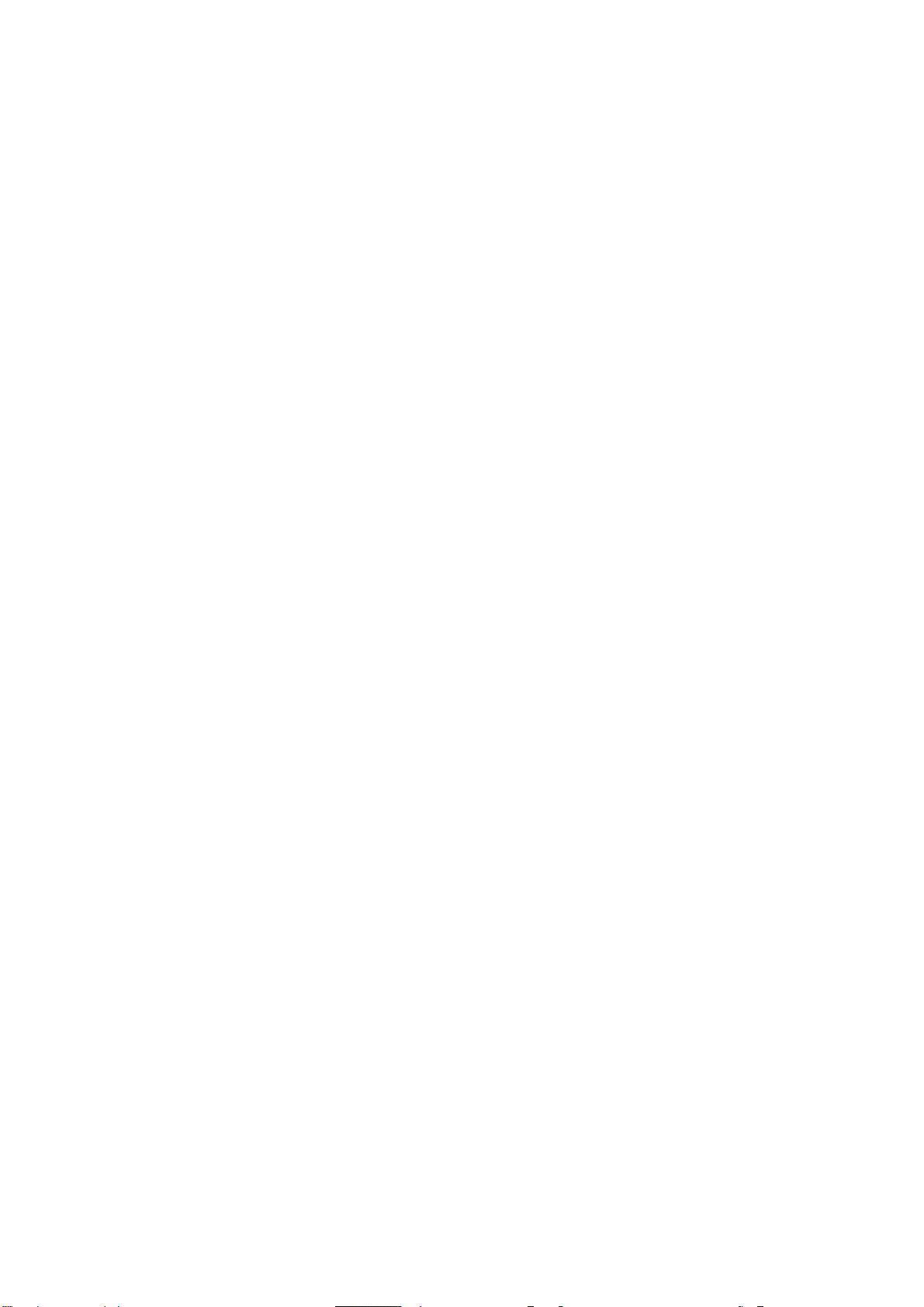
7
A class is a programmer-defined data type. C++ also provides a set of built-in data types: Boolean, integer,
floating point, and character. A keyword is associated with each one to allow us to specify the data type.
For example, to store the value entered by the user, we define an integer data object:
int usr_val;
int
is a language keyword identifying usr_val as a data object of integer type. Both the number of
guesses a user makes and the number of correct guesses are also integer objects. The difference here is that
we wish to set both of them to an initial value of 0. We can define each on a separate line:
int num_tries = 0;
int num_right = 0;
Or we can define them in a single comma-separated declaration statement:
int num_tries = 0, num_right = 0;
In general, it is a good idea to initialize data objects even if the value simply indicates that the object has
no useful value as yet. I didn't initialize
usr_val because its value is set directly from the user's input
before the program makes any use of the object.
An alternative initialization syntax, called a constructor syntax, is
int num_tries(0);
I know. Why are there two initialization syntaxes? And, worse, why am I telling you this now? Well, let's
see whether the following explanation satisfies either or both questions.
The use of the assignment operator (
=) for initialization is inherited from the C language. It works well
with the data objects of the built-in types and for class objects that can be initialized with a single value,
such as the string class:
string sequence_name = "Fibonacci";
It does not work well with class objects that require multiple initial values, such as the standard library
complex number class, which can take two initial values: one for its real component and one for its
imaginary component. The alternative constructor initialization syntax was introduced to handle multiple
value initialization:
#include <complex>
complex<double> purei(0, 7);
The strange bracket notation following complex indicates that the complex class is a template class. We'll
see a great deal more of template classes throughout the book. A template class allows us to define a class
without having to specify the data type of one or all of its members.
The complex number class, for example, contains two member data objects. One member represents the
real component of the number. The second member represents the imaginary component of the number.
These members need to be floating point data types, but which ones? C++ generously supports three
floating point size types: single precision, represented by the keyword
float; double precision,
represented by the keyword
double; and extended precision, represented by the two keywords long
double.