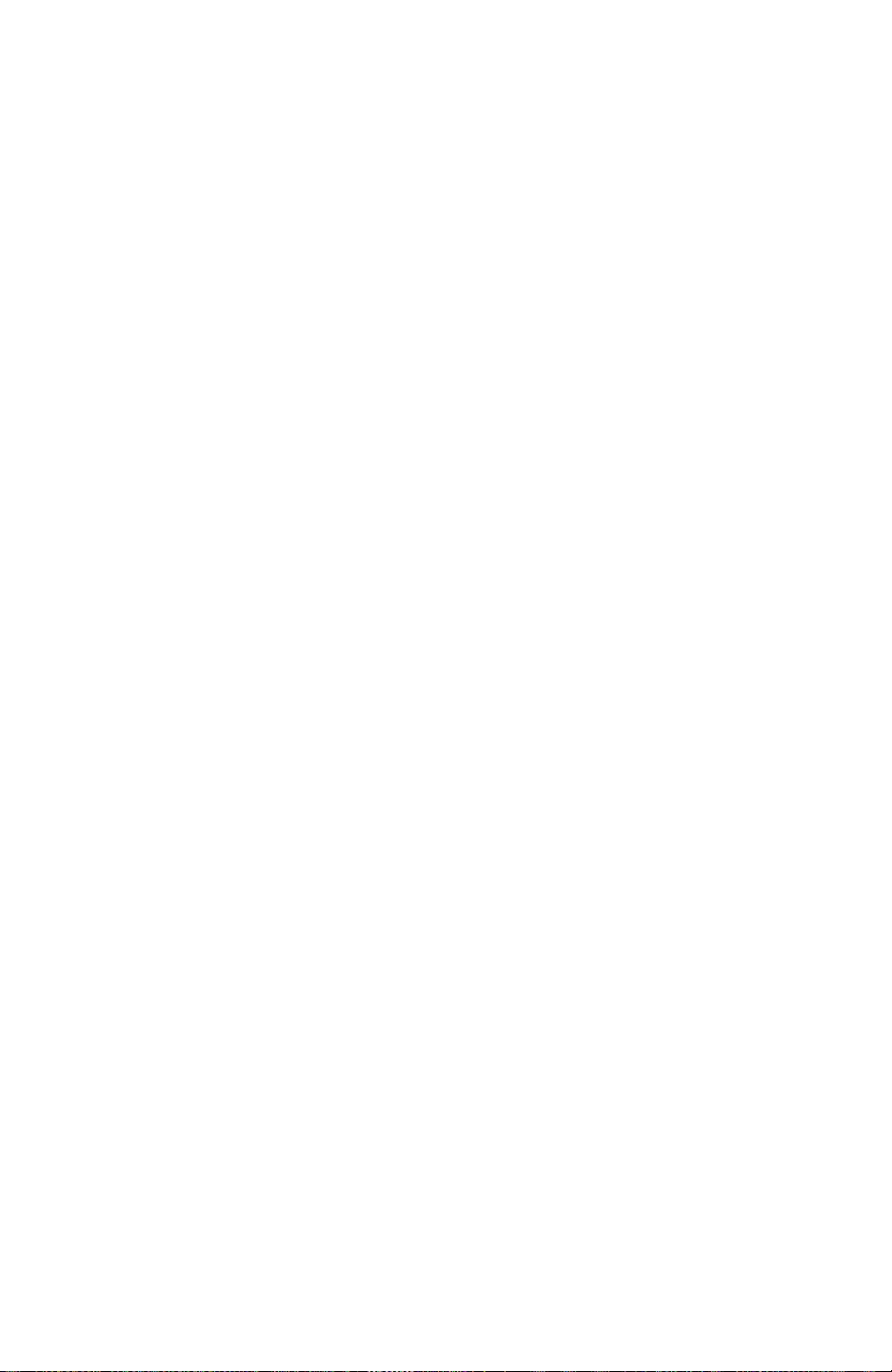
xviii
Preface
Assembly languages also have input/output (I/O) instructions to access I/O
devices on the computer. Input/output instructions are usually not available for high-
level languages. Also, assembly languages can access the stack, general-purpose
registers, base pointer registers, segment registers, and execute PUSH and POP oper-
ations.
The book presents the binary, octal, decimal, and hexadecimal number systems,
as well as the basic X86 processor architecture. The architecture includes the gen-
eral-purpose registers, the segment registers, the flags register, the instruction
pointer, and the floating-point registers. The following topics are also presented: dif-
ferent addressing modes, data transfer instructions, branching and looping opera-
tions, stack operations, logic, shift, and rotate instructions. Computer arithmetic
topics are presented in detail, including fixed-point, binary-coded decimal, and float-
ing-point instructions. There are additional chapters on procedures, string opera-
tions, arrays, macros, and input/output operations. The fundamentals of C
programming are covered in a separate chapter.
The book is intended to be tutorial, and as such, is comprehensive and self con-
tained. All program examples are carried through to completion — nothing is left
unfinished or partially designed. Also, all programs provide the outputs that result
from program execution. Each chapter includes numerous problems of varying com-
plexity to be designed by the reader.
Chapter
1
covers the number systems of different radices, such as binary, octal,
binary-coded octal, decimal, binary-coded decimal, hexadecimal, and binary-coded
hexadecimal. The chapter also presents the number representations of sign magni-
tude, diminished-radix complement, and radix complement.
Chapter
2
presents the generic architecture of processors and how the architec-
ture corresponds more appropriately to the X86 architecture execution environment,
including the different sets of registers. The chapter also covers the arithmetic and
logic unit (ALU), the control unit, and memory, including main memory and cache
memory. Error detection and correction is also discussed using the Hamming code
developed by Richard W. Hamming. A brief introduction to tape drives and disk
drives is also presented. The X86 register set is covered, which includes the general-
purpose registers (GPRs), the segment registers, the EFLAGS register containing
status flags, system flags, and a control flag. Other registers include the instruction
pointer and the floating-point registers. The translation lookaside buffer (TLB) and
the assembler are also briefly discussed.
Chapter
3
presents the various addressing modes of the X86 assembly language.
The instruction set provides various methods to address operands. The main meth-
ods are: register, immediate, direct, register indirect, base, index, and base combined
with index. A displacement may also be present. These and other addressing meth-
ods are presented in this chapter together with examples. The processor selects the
applicable default segment as a function of the instruction: instruction fetching
assumes the code segment; accessing data in main memory references the data seg-
ment; and instructions that pertain to the stack reference the stack segment. How-
ever, a
segment override prefix
can be used to change the default data segment to
another segment; that is, to explicitly specify any segment register to be used as the
current segment.