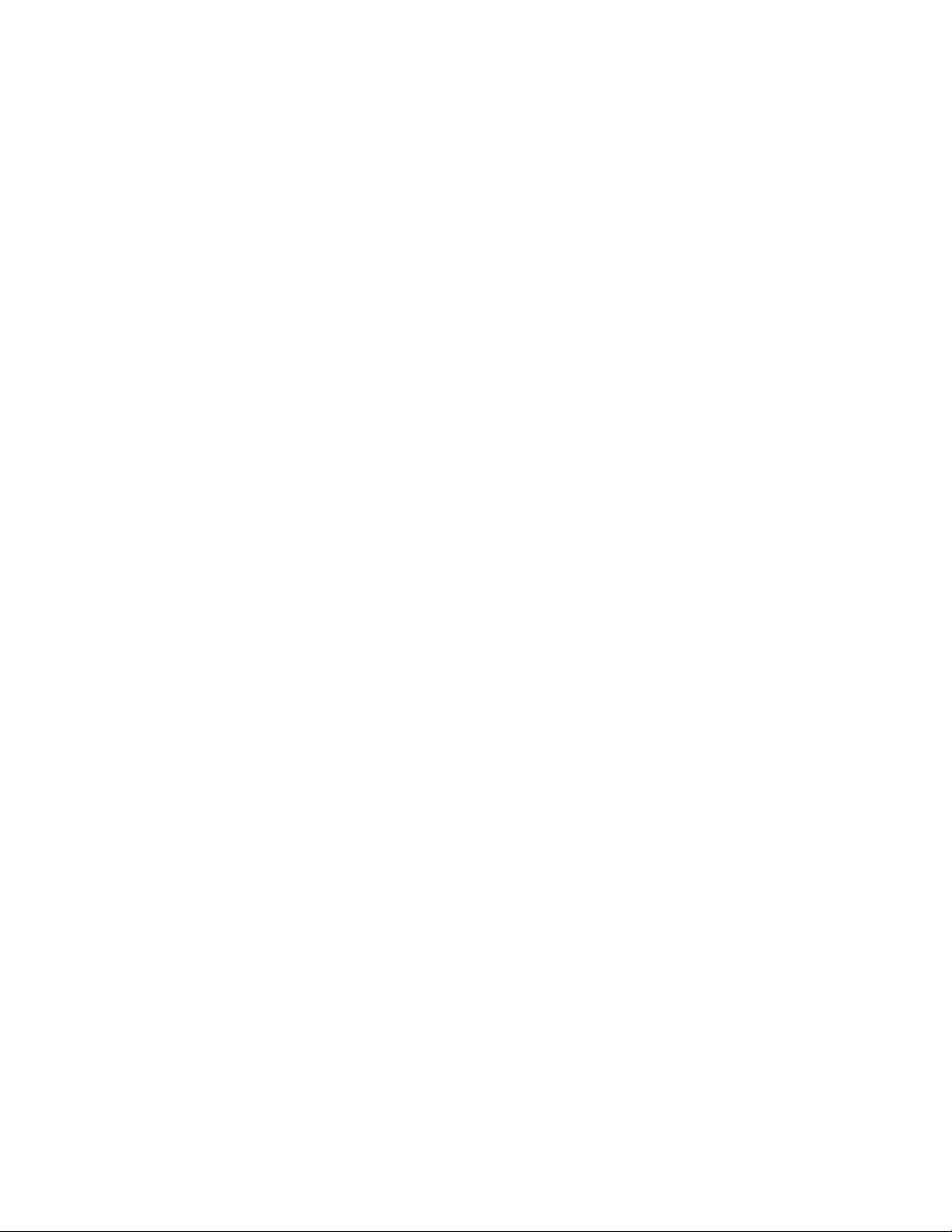
Dynamic, Recursive, Heterogeneous
Types in Statically-Typed Languages
A Presentation (20, 45 or 90 minute) for Intermediate to Advanced C++ Developers
Richard T. Saunders
Rincon Research Corporation
rts@rincon.com
Clint Jeffery
University of Idaho
jeffery@uidaho.edu
Abstract
Today’s modern software projects are written in many different
languages: Python, C++, Perl, Java, Javascript, Lua, Unicon, C,
all co-exist within a complex environment implementing different
components of a system. This proliferation of languages causes
dynamically-typed language concepts to infiltrate statically-typed
languages. One i mportant idea that seems ubiquitous across dy-
namic languages is t he dynamic, recursive, heterogeneous dictio-
nary: the Python dict, Perl hash, Javascript object, Lua table, and
Icon/Unicon table are all realizations of this abstraction—JSON
and, to a lesser extent, XML are language-agnostic versions of the
dynamic dictionary. Statically typed languages (like C++ and Java)
historically have trouble expressing and dealing with dynamic dic-
tionaries without cl umsy libraries. This paper explores how to rep-
resent dictionaries in C++ by introducing a simple, novel solution
for dealing with dynamic constructs by embracing unique static-
typing features of the C++ language: type-inference, user-defined
conversions, type selection, and overloading. Taken all together,
these static features paradoxically make dynamic dictionaries much
easier t o manipulate from C++, approaching the ease of dynamic
dictionary manipulation in dynamic languages. Note that these
techniques do not require special reflection facilities/libraries.
1. Introduction
Large software projects today are made up of many languages[20].
Performance critical code is typically written in statically-typed
languages like C/ C++ and possibly Java. Scripting and front-
end code is typically written in dynamically-typed languages like
Python, Ruby, Lua, Icon/Unicon and Javascript. This proliferation
of languages in r eal software projects has made it necessary for
information to propagate from one language to another: front-end
dynamic languages need to communicate with back-end static lan-
guages and vice-versa[4][9].
PicklingTools is a library meant to bridge the gap between static
and dynamic languages. The original goal of the PicklingTools
library was to make it easier to use Python and C++ together:
see http://www.picklingtools.com. At first, this involved getting
serialization libraries working; Python has a built-in serialization
Permission to make digital or hard copies of all or part of this work for personal or
classroom use is granted without fee provided that copies are not made or distributed
for profit or commercial advantage and that copies bear this notice and the full citation
on the first page. To copy otherwise, to republish, to post on servers or to redistribute
to lists, requires prior specific permission and/or a fee.
C++ Now Conference 2013
May 13-17, 2013.
Copyright
c
2013 ACM [to be supplied]. . . $10.00
format, called the pickle format[21], which is the de-facto format
for Python serializati on. To talk to Python, the PicklingTools library
implemented the pickling serialization in C++. Once this was done,
serialized data could then be transported between Python and C++
via files or sockets. The information that flowed between C++
and Python was the Python dictionary; it was the currency of the
system.
Over time, it became obvious that manipulating Python dictio-
naries in C++ is non-trivial. C++ is a statically-typed language and
Python is a dynamically-typed language: their approach to handling
hash tables and arrays is very different. If Python dictionaries are
the currency of choice, the C++ side has to be able to manipulate
these dictionaries as easily as Python for the library to be success-
ful. Originally a secondary goal, making dynamic dictionaries easy
to manipulate from C++ became a primary goal of the Pickling-
Tools library.
In Python, the dictionary (a.k.a., dict) is one of the fundamental
building blocks of the language: namespaces, modules, classes, are
all implemented using the dict; t his in t urn enables dynamic intro-
spection. Because of the ubiquity of these dictionaries in Python, or
perhaps because they are so important, dictionaries are fundamen-
tally easy to manipulate, create and read.
The Python dictionary has three main properties of interest.
1. Heterogeneous: values in dictionaries can be any type, and keys
can be almost any type (as long as they are hashable).
2. Recursive: dictionaries can contain dictionaries of dictionaries,
ad infinitum. This is closely related to heterogeneity, as t he dic-
tionary is just another type, but the recursive nature is well-
supported in Python. For example, cascading lookups and in-
sertions are easily expressible ( see below) so that nested dicts
are accessible.
3. Dynamic: the values can change at run-time. This is also closely
related to heterogeneity: values can be any type, but that type
can also vary at run-time. A C struct, in contrast, can contain
heterogeneous types, but can’t change after compile time.
Manipulating dictionaries is natural in the Python language.
# Python
>>> v = "abc"
>>> v = 1 # dynamic values
# heterogenous types in dict
>>> d = { ’a’: v, ’nest’: { ’b’: 3.14 }}
# recursive, supports cascading lookup
>>> print d[’nest’][’b’]