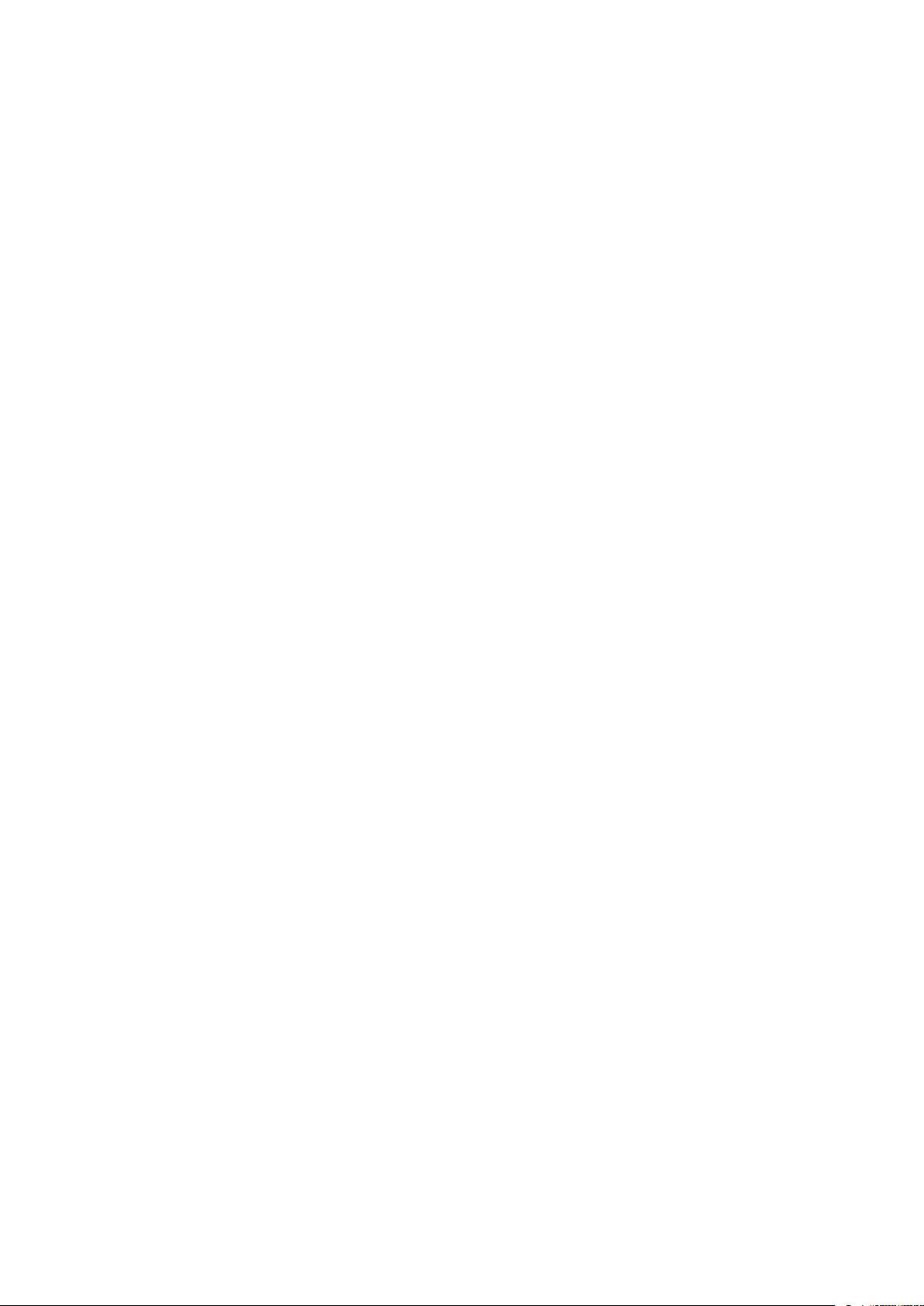
Contents
1 On C++ and programming. 4
1.1 Compiling and linking . . . . . . . . . . . . . . . . 4
1.2 The structure of a C++ program . . . . . . . . . . 4
1.2.1 Types . . . . . . . . . . . . . . . . . . . . . 4
1.2.2 Operations . . . . . . . . . . . . . . . . . . . 5
1.2.3 Functions and libraries . . . . . . . . . . . . 5
1.2.4 Templates and libraries . . . . . . . . . . . . 6
1.2.5 Flow control . . . . . . . . . . . . . . . . . . 6
1.2.6 Input Output . . . . . . . . . . . . . . . . . 7
1.2.7 Splitting up a program . . . . . . . . . . . . 7
1.2.8 Namespaces . . . . . . . . . . . . . . . . . . 8
1.3 Extending the language, the class concept. . . . . . 8
1.3.1 date, an example class . . . . . . . . . . . . 8
1.4 Const references . . . . . . . . . . . . . . . . . . . . 10
2 The value of time 11
2.1 Present value. . . . . . . . . . . . . . . . . . . . . . 11
2.2 Internal rate of return. . . . . . . . . . . . . . . . . 12
2.2.1 Check for unique IRR . . . . . . . . . . . . . 15
2.3 Bonds . . . . . . . . . . . . . . . . . . . . . . . . . 16
2.3.1 Bond Price . . . . . . . . . . . . . . . . . . . 16
2.3.2 Yield to maturity. . . . . . . . . . . . . . . . 17
2.3.3 Duration. . . . . . . . . . . . . . . . . . . . 19
3 The term structure of interest rates and an object
lesson 21
3.1 Term structure calculations . . . . . . . . . . . . . . 21
3.2 Using the currently observed term structure. . . . . 22
3.2.1 Linear Interpolation. . . . . . . . . . . . . . 22
3.3 The term structure as an object . . . . . . . . . . . 22
3.4 Implementing a term structure class . . . . . . . . . 23
3.4.1 Base class . . . . . . . . . . . . . . . . . . . 23
3.4.2 Flat term structure. . . . . . . . . . . . . . . 25
3.4.3 Interpolated term structure. . . . . . . . . . 26
3.5 Bond calculations using the term structure class . . 28
4 Futures algoritms. 29
4.1 Pricing of futures contract. . . . . . . . . . . . . . . 29
5 Binomial option pricing 30
5.1 Multiperiod binomial pricing . . . . . . . . . . . . . 32
6 Basic Option Pricing, the Black Scholes formula 37
6.1 The formula . . . . . . . . . . . . . . . . . . . . . . 37
6.2 Understanding the why’s of the formula . . . . . . . 40
6.2.1 The original Black Scholes analysis . . . . . 40
6.2.2 The limit of a binomial case . . . . . . . . . 40
6.2.3 The representative agent framework . . . . . 41
6.3 Partial derivatives. . . . . . . . . . . . . . . . . . . 41
6.3.1 Delta . . . . . . . . . . . . . . . . . . . . . . 41
6.3.2 Other Derivatives . . . . . . . . . . . . . . . 41
6.3.3 Implied Volatility. . . . . . . . . . . . . . . . 44
7 Warrants 47
7.1 Warrant value in terms of assets . . . . . . . . . . . 47
7.2 Valuing warrants when observing the stock value . . 48
7.3 Readings . . . . . . . . . . . . . . . . . . . . . . . . 48
8 Extending the Black Scholes formula 50
8.1 Adjusting for payouts of the underlying. . . . . . . . 50
8.1.1 Continous Payouts from underlying. . . . . . 50
8.1.2 Dividends. . . . . . . . . . . . . . . . . . . . 51
8.2 American options. . . . . . . . . . . . . . . . . . . . 52
8.2.1 Exact american call formula when stock is
paying one dividend. . . . . . . . . . . . . . 52
8.3 Options on futures . . . . . . . . . . . . . . . . . . 56
8.3.1 Black’s model . . . . . . . . . . . . . . . . . 56
8.4 Foreign Currency Options . . . . . . . . . . . . . . 58
8.5 Perpetual puts and calls . . . . . . . . . . . . . . . 60
8.6 Readings . . . . . . . . . . . . . . . . . . . . . . . . 61
9 Option pricing with binomial approximations 62
9.1 Introduction . . . . . . . . . . . . . . . . . . . . . . 62
9.2 Pricing of options in the Black Scholes setting . . . 63
9.2.1 European Options . . . . . . . . . . . . . . . 63
9.2.2 American Options . . . . . . . . . . . . . . . 63
9.2.3 Estimating partials. . . . . . . . . . . . . . . 66
9.3 Adjusting for payouts for the underlying . . . . . . 69
9.4 Pricing options on stocks paying dividends using a
binomial approximation . . . . . . . . . . . . . . . . 70
9.4.1 Checking for early exercise in the binomial
model. . . . . . . . . . . . . . . . . . . . . . 70
9.4.2 Proportional dividends. . . . . . . . . . . . . 70
9.4.3 Discrete dividends . . . . . . . . . . . . . . . 70
9.5 Option on futures . . . . . . . . . . . . . . . . . . . 74
9.6 Foreign Currency options . . . . . . . . . . . . . . . 75
9.7 References . . . . . . . . . . . . . . . . . . . . . . . 76
10 Finite Differences 77
10.1 Explicit Finite differences . . . . . . . . . . . . . . . 77
10.2 European Options. . . . . . . . . . . . . . . . . . . 77
10.3 American Options. . . . . . . . . . . . . . . . . . . 79
11 Option pricing by simulation 81
11.1 Simulating lognormally distributed random variables 81
11.2 Pricing of European Call options . . . . . . . . . . . 82
11.3 Hedge parameters . . . . . . . . . . . . . . . . . . . 83
11.4 More general payoffs. Function prototypes . . . . . 83
11.5 Improving the efficiency in simulation . . . . . . . . 85
11.5.1 Control variates. . . . . . . . . . . . . . . . 85
11.5.2 Antithetic variates. . . . . . . . . . . . . . . 86
11.5.3 Example . . . . . . . . . . . . . . . . . . . . 88
11.6 More exotic options . . . . . . . . . . . . . . . . . . 90
11.7 Exercises . . . . . . . . . . . . . . . . . . . . . . . . 90
12 Approximations 91
12.1 A quadratic approximation to American prices due
to Barone–Adesi and Whaley. . . . . . . . . . . . . 91
13 Average, lookback and other exotic options 95
13.1 Bermudan options . . . . . . . . . . . . . . . . . . . 95
13.2 Asian options . . . . . . . . . . . . . . . . . . . . . 98
13.3 Lookback options . . . . . . . . . . . . . . . . . . . 99
13.4 Monte Carlo Pricing of options whose payoff depend
on the whole price path . . . . . . . . . . . . . . . . 100
13.4.1 Generating a series of lognormally dis-
tributed variables . . . . . . . . . . . . . . . 100
13.5 Control variate . . . . . . . . . . . . . . . . . . . . 103
1