没有合适的资源?快使用搜索试试~ 我知道了~
首页Packt C++ 17 STL Cookbook.2017
Packt C++ 17 STL Cookbook.2017
需积分: 13 13 下载量 141 浏览量
更新于2023-05-24
评论
收藏 11.26MB PDF 举报
The New C++17 Features In this chapter, we will cover thefollowing recipes: Using structured bindings to unpack bundled return values Limiting variable scopes to if and switch statements Profiting from the new bracket initializer rules Letting the constructor automatically deduce the resulting template class type Simplifying compile-time decisions with constexpr-if Enabling header-only libraries with inline variables Implementing handy helper functions with fold expressions
资源详情
资源评论
资源推荐
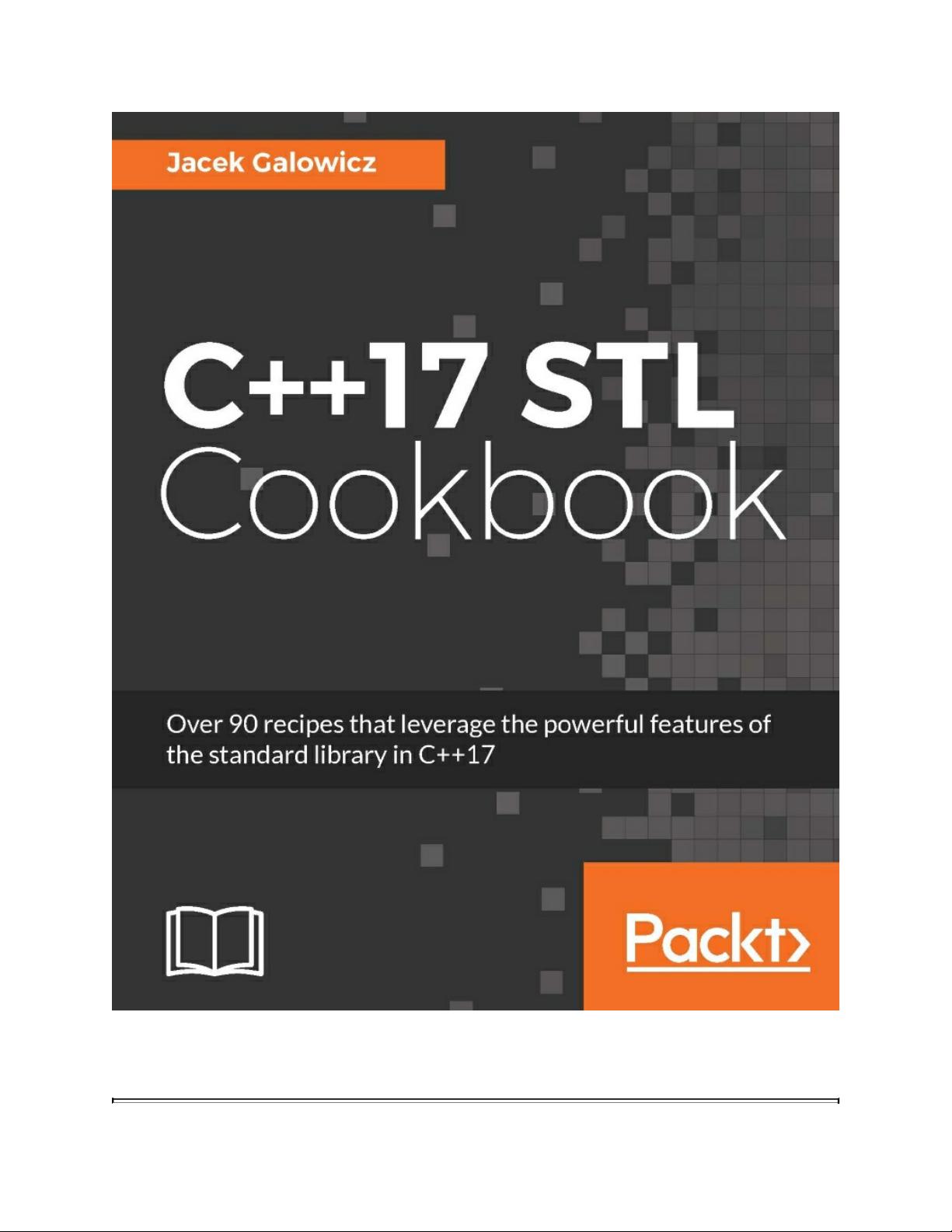
Contents
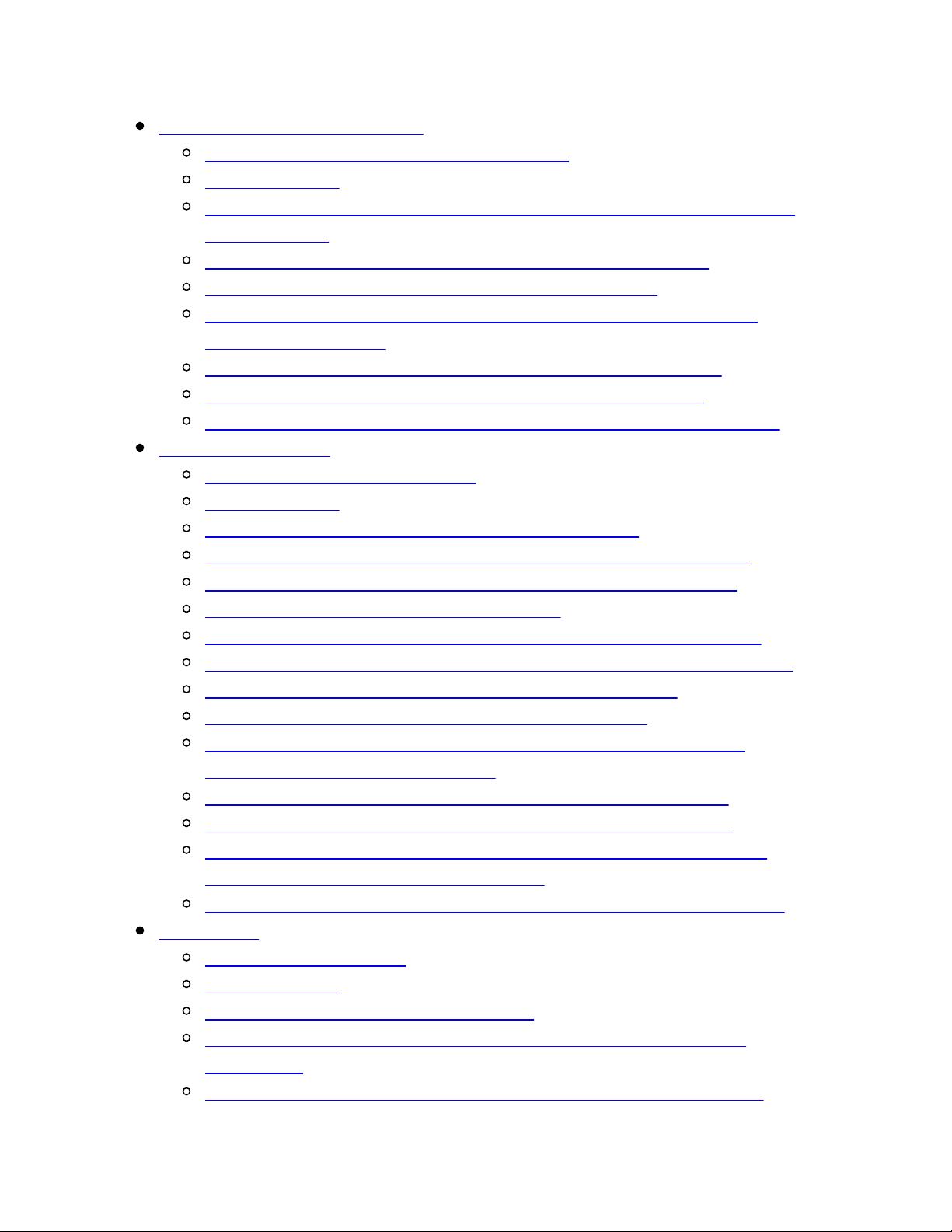
1: The New C++17 Features
b'Chapter 1: The New C++17 Features'
b'Introduction'
b'Using structured bindings to\xc3\x82\xc2\xa0unpack bundled
return values'
b'Limiting variable scopes to if and switch statements'
b'Profiting from the new bracket initializer rules'
b'Letting the constructor automatically deduce the resulting
template class type'
b'Simplifying compile time decisions with constexpr-if'
b'Enabling header-only libraries with inline variables'
b'Implementing handy helper functions with fold expressions'
2: STL Containers
b'Chapter 2: STL Containers'
b'Introduction'
b'Using the erase-remove idiom on std::vector'
b'Deleting items from an unsorted std::vector in O(1) time'
b'Accessing std::vector instances the fast or the safe way'
b'Keeping std::vector instances sorted'
b'Inserting items efficiently and conditionally into std::map'
b'Knowing the new insertion hint semantics of std::map::insert'
b'Efficiently modifying the keys of std::map items'
b'Using std::unordered_map with custom types'
b'Filtering duplicates from user input and printing them in
alphabetical order with std::set'
b'Implementing a simple RPN calculator with std::stack'
b'Implementing a word frequency counter with std::map'
b'Implement a writing style helper tool for finding very long
sentences in text with std::multimap'
b'Implementing a personal to-do list using std::priority_queue'
3: Iterators
b'Chapter 3: Iterators'
b'Introduction'
b'Building your own iterable range'
b'Making your own iterators compatible with STL iterator
categories'
b'Using iterator adapters\xc3\x82\xc2\xa0to fill generic data
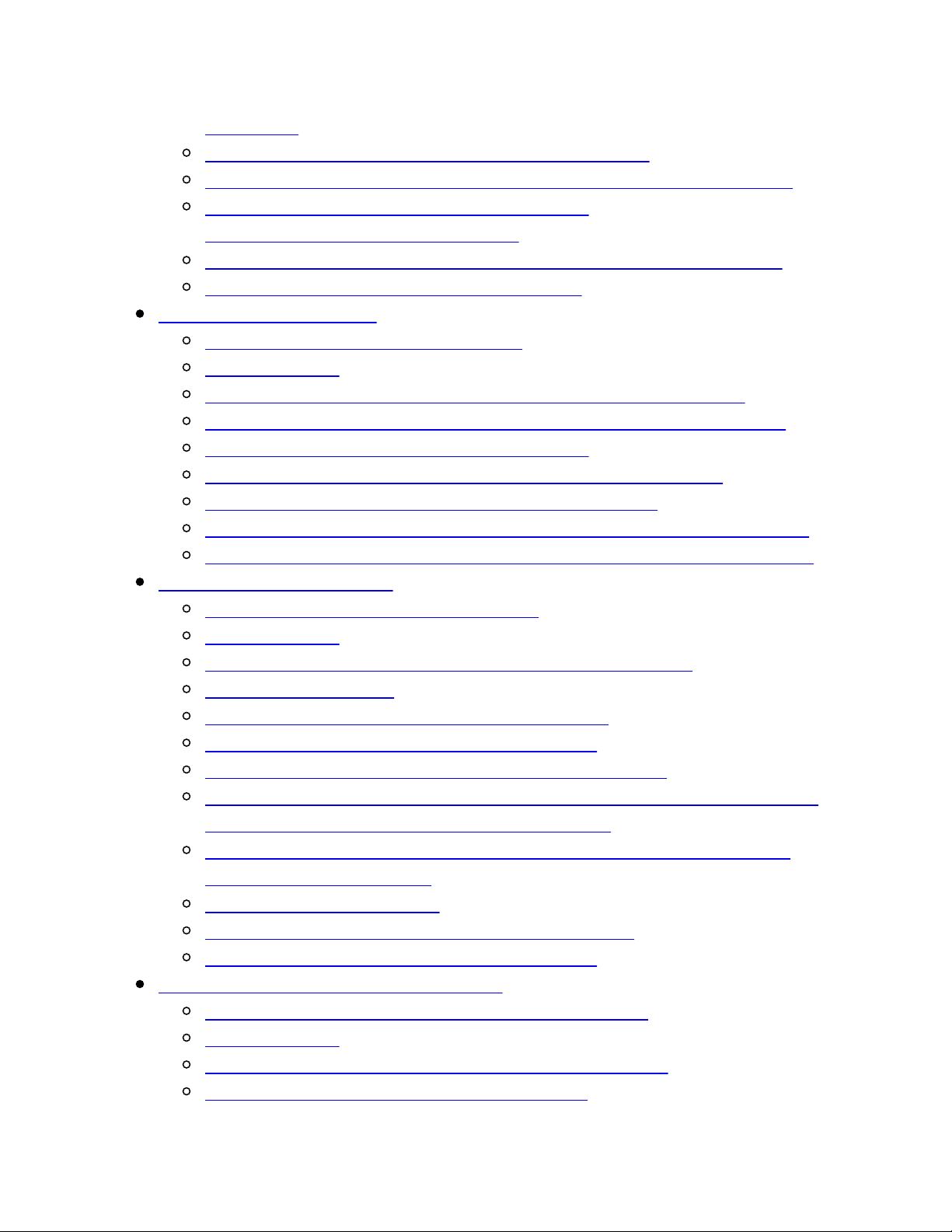
structures'
b'Implementing algorithms in terms of iterators'
b'Iterating the other way around using reverse iterator adapters'
b'Terminating iterations over ranges with
iterator\xc3\x82\xc2\xa0sentinels'
b'Automatically checking iterator code with checked iterators'
b'Building your own zip iterator adapter'
4: Lambda Expressions
b'Chapter 4: Lambda Expressions'
b'Introduction'
b'Defining functions on the run using lambda expressions'
b'Adding polymorphy by wrapping lambdas into std::function'
b'Composing functions by concatenation'
b'Creating complex predicates with logical conjunction'
b'Calling multiple functions with the same input'
b'Implementing transform_if using std::accumulate and lambdas'
b'Generating cartesian product pairs of any input at compile time'
5: STL Algorithm Basics
b'Chapter 5: STL Algorithm Basics'
b'Introduction'
b'Copying items from containers to other containers'
b'Sorting containers'
b'Removing specific items from containers'
b'Transforming the contents of containers'
b'Finding items in ordered and unordered vectors'
b'Limiting\xc3\x82\xc2\xa0the values\xc3\x82\xc2\xa0of a vector
to a specific numeric range with std::clamp'
b'Locating patterns in strings with std::search and choosing the
optimal implementation'
b'Sampling large vectors'
b'Generating permutations of input sequences'
b'Implementing a dictionary merging tool'
6: Advanced Use of STL Algorithms
b'Chapter 6: Advanced Use of STL Algorithms'
b'Introduction'
b'Implementing a trie class using STL algorithms'
b'Implementing\xc3\x82\xc2\xa0a search
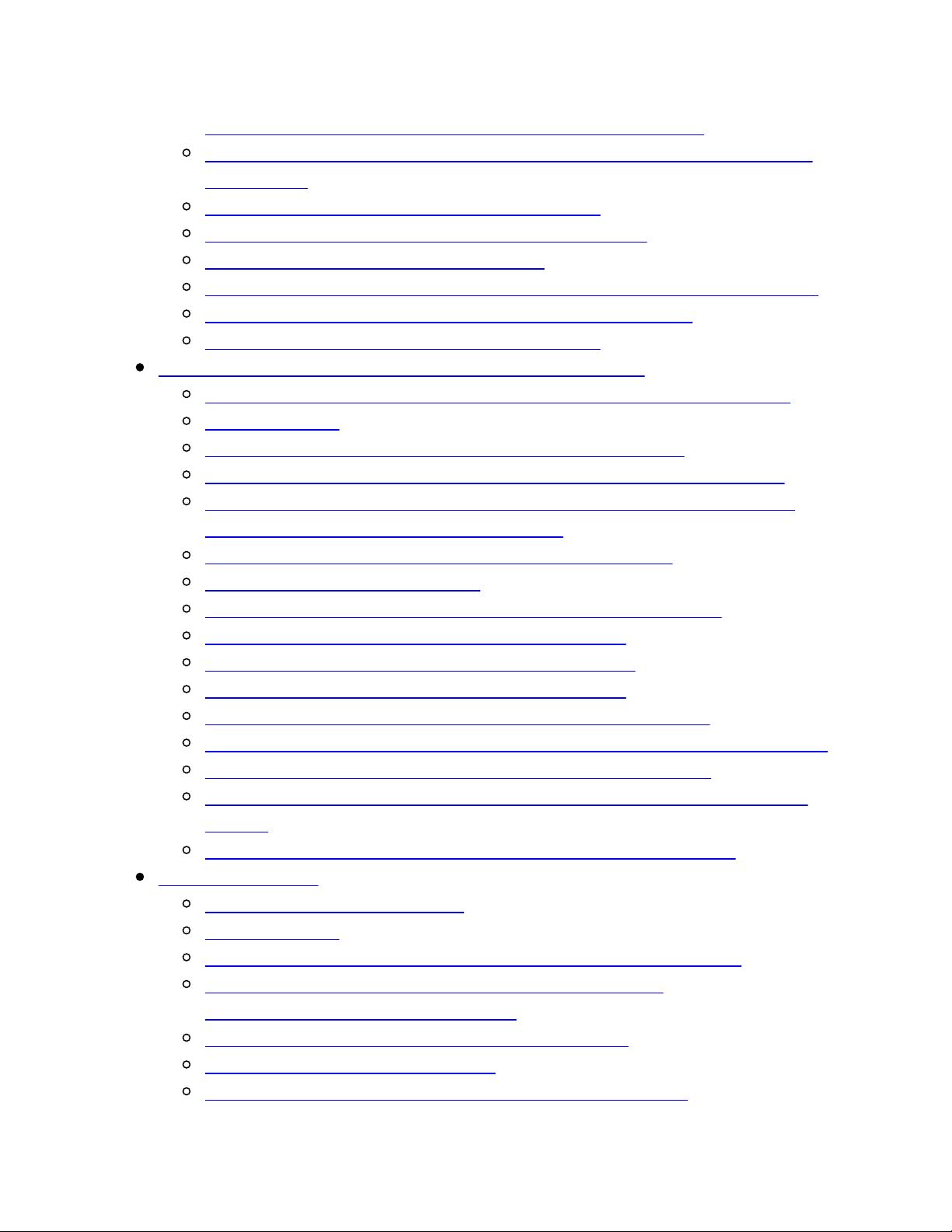
input\xc3\x82\xc2\xa0suggestion generator with tries'
b'Implementing the Fourier transform formula with STL numeric
algorithms'
b'Calculating the error sum of two vectors'
b'Implementing an ASCII Mandelbrot renderer'
b'Building our own algorithm - split'
b'Composing useful algorithms from standard algorithms - gather'
b'Removing consecutive whitespace between words'
b'Compressing and decompressing strings'
7: Strings, Stream Classes, and Regular Expressions
b'Chapter 7: Strings, Stream Classes, and Regular Expressions'
b'Introduction'
b'Creating, concatenating, and transforming strings'
b'Trimming whitespace from the beginning and end of strings'
b'Getting the comfort of std::string\xc3\x82\xc2\xa0without the
cost of constructing std::string objects'
b'Reading values\xc3\x82\xc2\xa0from user input'
b'Counting all words in a file'
b'Formatting your output with I/O stream manipulators'
b'Initializing complex objects from file input'
b'Filling containers from std::istream iterators'
b'Generic printing with std::ostream iterators'
b'Redirecting output to files for specific code sections'
b'Creating custom string classes by inheriting from std::char_traits'
b'Tokenizing input with the regular expression library'
b'Comfortably pretty printing numbers differently per context on
the fly'
b'Catching readable exceptions from std::iostream errors'
8: Utility Classes
b'Chapter 8: Utility Classes'
b'Introduction'
b'Converting between different time units using std::ratio'
b'Converting between absolute and relative times
with\xc3\x82\xc2\xa0std::chrono'
b'Safely signalizing failure with std::optional'
b'Applying functions on tuples'
b'Quickly composing data structures with std::tuple'
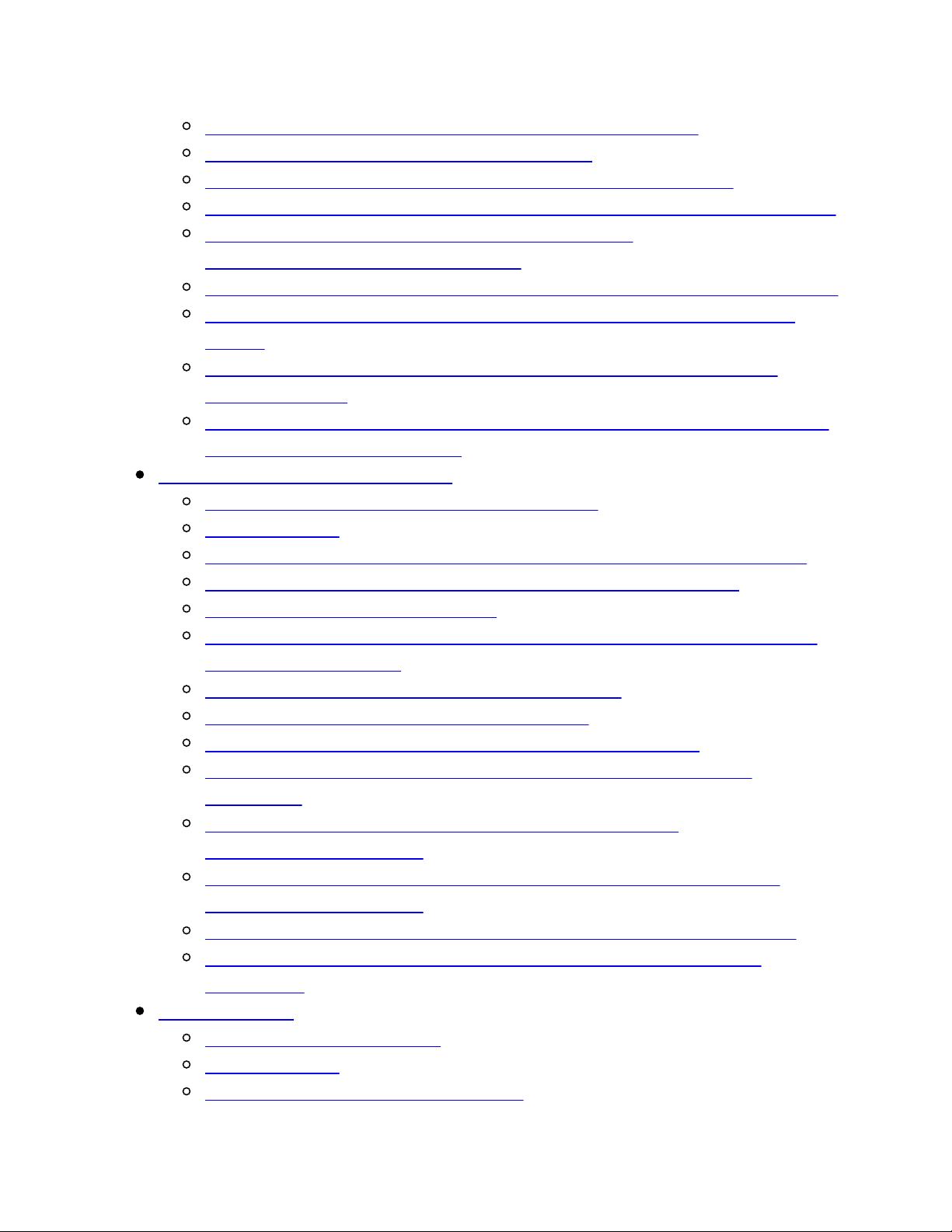
b'Replacing void* with std::any for more type safety'
b'Storing different types with std::variant'
b'Automatically handling resources with std::unique_ptr'
b'Automatically handling shared heap memory with std::shared_ptr'
b'Dealing with\xc3\x82\xc2\xa0weak pointers
to\xc3\x82\xc2\xa0shared objects'
b'Simplifying resource handling of legacy APIs with smart pointers'
b'Sharing different member values\xc3\x82\xc2\xa0of the same
object'
b'Generating random numbers and choosing the right random
number engine'
b'Generating\xc3\x82\xc2\xa0random numbers and letting the STL
shape specific distributions'
9: Parallelism and Concurrency
b'Chapter 9: Parallelism and Concurrency'
b'Introduction'
b'Automatically parallelizing code that uses standard algorithms'
b'Putting a program to sleep for specific amounts of time'
b'Starting and stopping threads'
b'Performing exception safe shared locking with std::unique_lock
and std::shared_lock'
b'Avoiding deadlocks with std::scoped_lock'
b'Synchronizing concurrent std::cout use'
b'Safely postponing initialization with std::call_once'
b'Pushing the execution of tasks into the background using
std::async'
b'Implementing the producer/consumer idiom with
std::condition_variable'
b'Implementing the multiple producers/consumers idiom with
std::condition_variable'
b'Parallelizing the ASCII Mandelbrot renderer using std::async'
b'Implementing a tiny automatic parallelization library with
std::future'
10: Filesystem
b'Chapter 10: Filesystem'
b'Introduction'
b'Implementing a path normalizer'
剩余588页未读,继续阅读
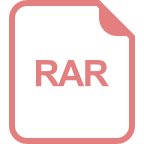
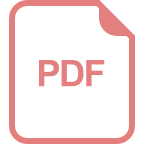
















fuller1234
- 粉丝: 0
- 资源: 7
上传资源 快速赚钱
我的内容管理 收起
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

会员权益专享
最新资源
- RTL8188FU-Linux-v5.7.4.2-36687.20200602.tar(20765).gz
- c++校园超市商品信息管理系统课程设计说明书(含源代码) (2).pdf
- 建筑供配电系统相关课件.pptx
- 企业管理规章制度及管理模式.doc
- vb打开摄像头.doc
- 云计算-可信计算中认证协议改进方案.pdf
- [详细完整版]单片机编程4.ppt
- c语言常用算法.pdf
- c++经典程序代码大全.pdf
- 单片机数字时钟资料.doc
- 11项目管理前沿1.0.pptx
- 基于ssm的“魅力”繁峙宣传网站的设计与实现论文.doc
- 智慧交通综合解决方案.pptx
- 建筑防潮设计-PowerPointPresentati.pptx
- SPC统计过程控制程序.pptx
- SPC统计方法基础知识.pptx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


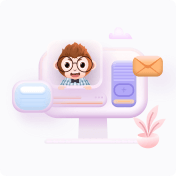
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0