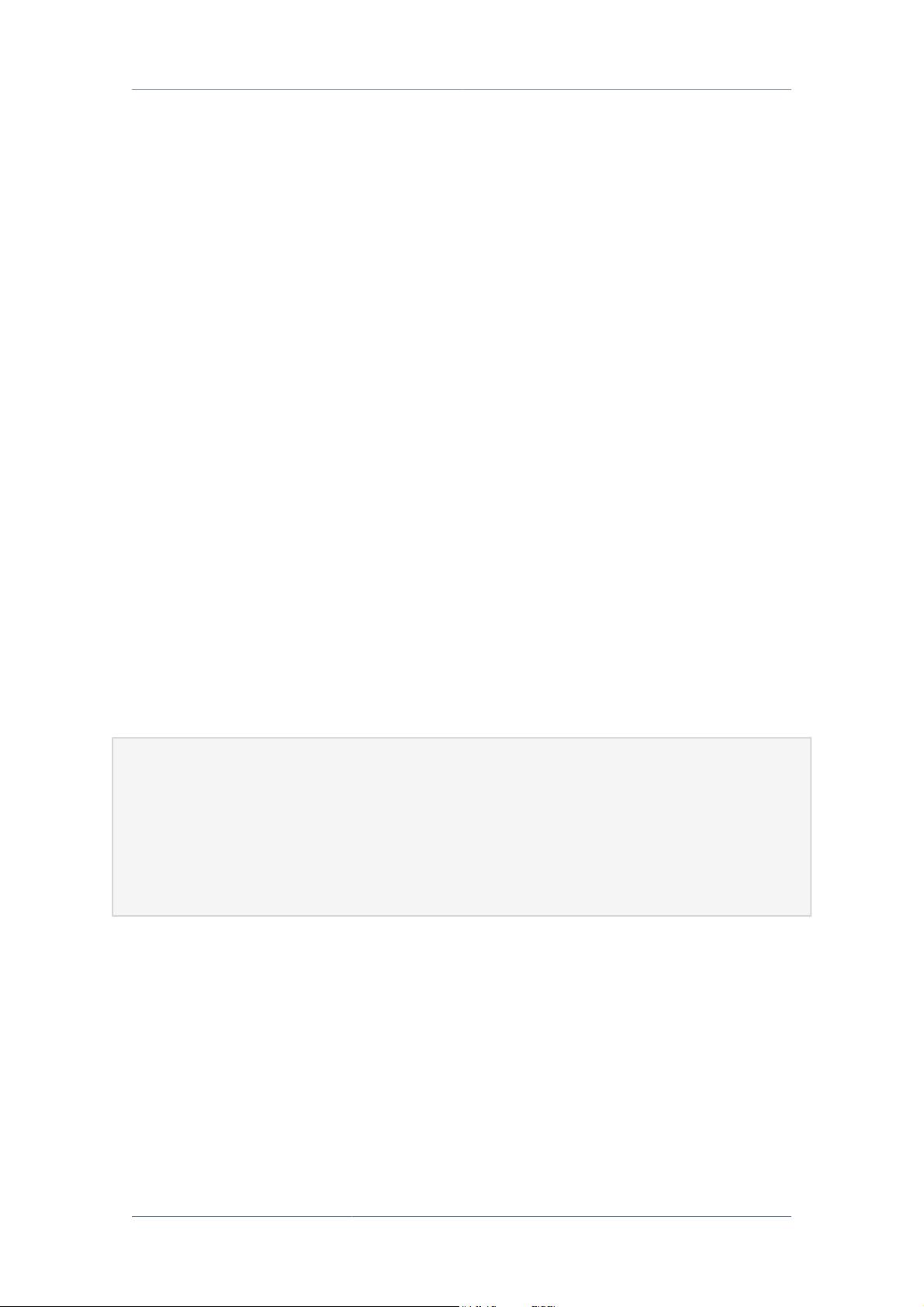
Chapter 1. Tutorial
4
required. Hibernate can also access fields directly, the benefit of accessor methods is robustness
for refactoring.
The id property holds a unique identifier value for a particular event. All persistent entity classes
(there are less important dependent classes as well) will need such an identifier property if we want
to use the full feature set of Hibernate. In fact, most applications, especially web applications, need
to distinguish objects by identifier, so you should consider this a feature rather than a limitation.
However, we usually do not manipulate the identity of an object, hence the setter method should
be private. Only Hibernate will assign identifiers when an object is saved. Hibernate can access
public, private, and protected accessor methods, as well as public, private and protected fields
directly. The choice is up to you and you can match it to fit your application design.
The no-argument constructor is a requirement for all persistent classes; Hibernate has to create
objects for you, using Java Reflection. The constructor can be private, however package or public
visibility is required for runtime proxy generation and efficient data retrieval without bytecode
instrumentation.
Save this file to the src/main/java/org/hibernate/tutorial/domain directory.
1.1.3. The mapping file
Hibernate needs to know how to load and store objects of the persistent class. This is where
the Hibernate mapping file comes into play. The mapping file tells Hibernate what table in the
database it has to access, and what columns in that table it should use.
The basic structure of a mapping file looks like this:
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping package="org.hibernate.tutorial.domain">
[...]
</hibernate-mapping>
Hibernate DTD is sophisticated. You can use it for auto-completion of XML mapping elements
and attributes in your editor or IDE. Opening up the DTD file in your text editor is the easiest
way to get an overview of all elements and attributes, and to view the defaults, as well as some
comments. Hibernate will not load the DTD file from the web, but first look it up from the classpath
of the application. The DTD file is included in hibernate-core.jar (it is also included in the
hibernate3.jar, if using the distribution bundle).