本资源是一份详尽的Python 2.7语法参考指南,特别针对初学者设计,旨在提供清晰的教程和实用的指导。它分为多个部分,包括但不限于: 1. **前言**:介绍了版本2.7的更新内容,以及用户可以访问官方更新信息的网址,并鼓励报告任何发现的问题、不准确之处和改进建议,以便与作者Richard Gruet保持联系。 2. **调用选项**:这部分可能涉及如何正确地启动和配置Python解释器,包括环境变量设置。 3. **词法实体**:详细解释了Python的关键字、标识符、字符串常量、布尔值、数字类型、序列(如列表)、字典、集合、运算符等基础概念。 4. **基本类型和操作**:涵盖了Python的None、布尔值、数值类型(整型、浮点型等)、序列处理、文件操作、集合、命名元组、日期/时间等核心概念及操作方法。 5. **高级类型**:介绍了迭代器、生成器、描述符、装饰器等进阶主题,这些都是实现更复杂程序结构的重要工具。 6. **语句与控制流程**:涵盖赋值、条件表达式、控制流(if-else、循环等)、异常处理以及函数和类定义的语法和用法。 7. **内置函数和异常**:列出了Python内置的函数库,如sys、os、time等,以及如何处理常见的错误和异常。 8. **用户自定义类中的标准方法与重写**:讲解如何在用户创建的类中覆盖或扩展内置操作。 9. **特殊信息状态属性**:介绍了一些数据类型特有的状态属性,有助于深入理解Python内部机制。 10. **重要模块**:列举了标准库中的一些关键模块,如re(正则表达式)、math(数学计算)、os(操作系统接口)等,以及它们在实际开发中的应用。 11. **工作区探索与编程习惯提示**:提供了如何有效利用工作空间资源以及遵循Python编程的最佳实践建议。 12. **Emacs下的Python模式**:如果读者使用Emacs编辑器,这部分将介绍如何设置Python特定的环境和快捷键。 最后,整个文档遵循Creative Commons许可协议,允许用户在规定范围内自由分享和使用。这份参考文档是学习和掌握Python 2.7语法的良好起点,无论是初学者还是进阶开发者都能从中获益良多。
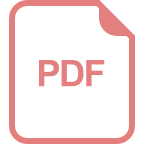
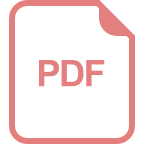

















- 粉丝: 0
- 资源: 9
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- 解决Eclipse配置与导入Java工程常见问题
- 真空发生器:工作原理与抽吸性能分析
- 爱立信RBS6201开站流程详解
- 电脑开机声音解析:故障诊断指南
- JAVA实现贪吃蛇游戏
- 模糊神经网络实现与自学习能力探索
- PID型模糊神经网络控制器设计与学习算法
- 模糊神经网络在自适应PID控制器中的应用
- C++实现的学生成绩管理系统设计
- 802.1D STP 实现与优化:二层交换机中的生成树协议
- 解决Windows无法完成SD卡格式化的九种方法
- 软件测试方法:Beta与Alpha测试详解
- 软件测试周期详解:从需求分析到维护测试
- CMMI模型详解:软件企业能力提升的关键
- 移动Web开发框架选择:jQueryMobile、jQTouch、SenchaTouch对比
- Java程序设计试题与复习指南

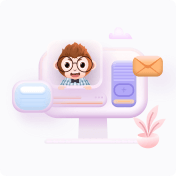
