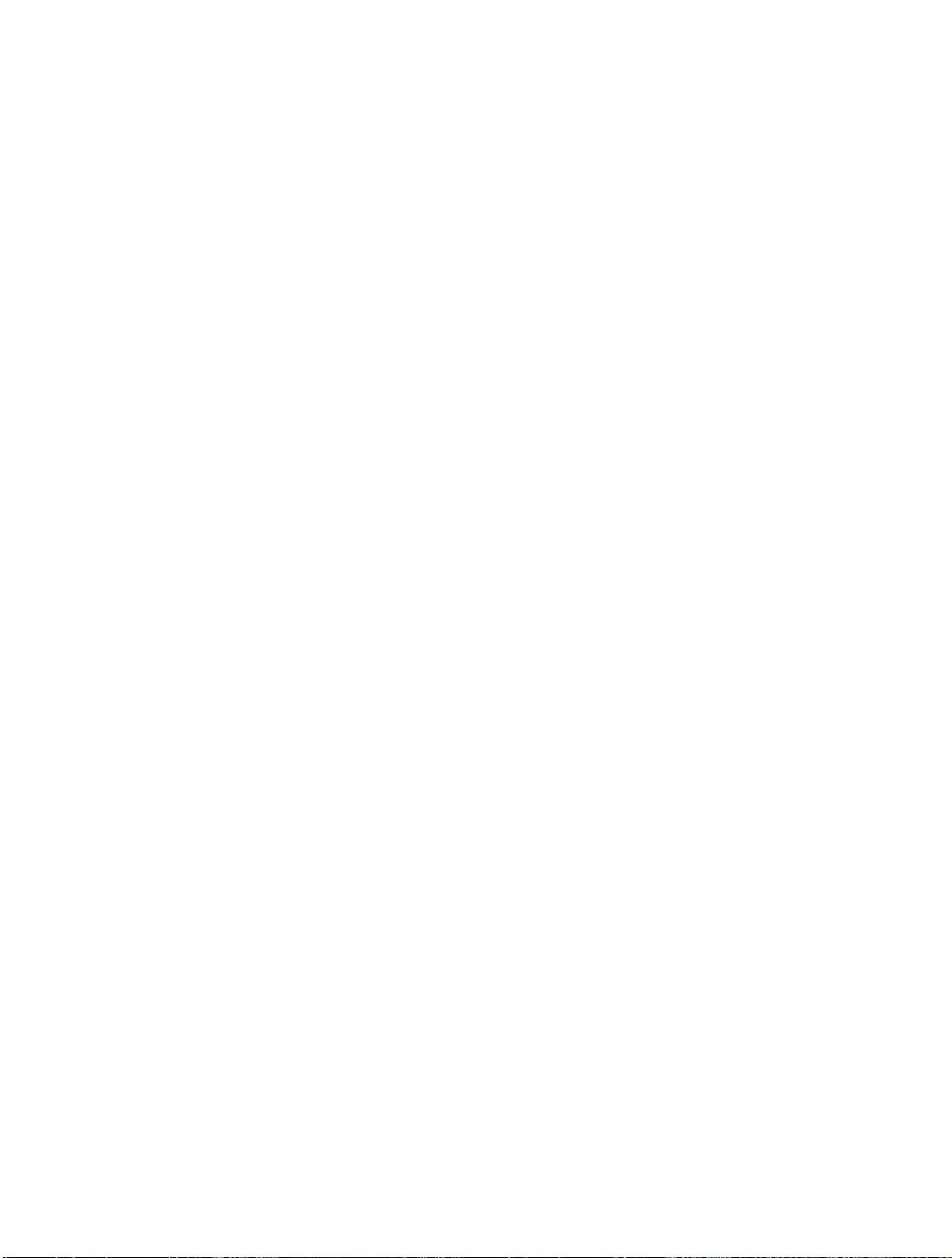
xiv Contents in Detail
Global Promise Rejection Handling .................................... 224
Node.js Rejection Handling ................................... 225
Browser Rejection Handling ................................... 227
Chaining Promises ................................................ 228
Catching Errors............................................ 229
Returning Values in Promise Chains.............................. 230
Returning Promises in Promise Chains ............................ 231
Responding to Multiple Promises ...................................... 233
The Promise.all() Method ..................................... 234
The Promise.race() Method.................................... 235
Inheriting from Promises ............................................ 236
Promise-Based Asynchronous Task Running ............................... 237
Summary ...................................................... 241
12
PROXIES AND THE REFLECTION API 243
The Array Problem ................................................ 244
Introducing Proxies and Reflection ..................................... 244
Creating a Simple Proxy ............................................ 245
Validating Properties Using the set Trap ................................. 246
Object Shape Validation Using the get Trap .............................. 247
Hiding Property Existence Using the has Trap ............................. 249
Preventing Property Deletion with the deleteProperty Trap ..................... 250
Prototype Proxy Traps .............................................. 252
How Prototype Proxy Traps Work ............................... 252
Why Two Sets of Methods? ................................... 254
Object Extensibility Traps ........................................... 255
Two Basic Examples ........................................ 255
Duplicate Extensibility Methods................................. 256
Property Descriptor Traps ........................................... 257
Blocking Object.defineProperty()................................ 258
Descriptor Object Restrictions .................................. 259
Duplicate Descriptor Methods .................................. 260
The ownKeys Trap ................................................ 261
Function Proxies with the apply and construct Traps ......................... 262
Validating Function Parameters................................. 264
Calling Constructors Without new ............................... 265
Overriding Abstract Base Class Constructors ....................... 266
Callable Class Constructors ................................... 267
Revocable Proxies ................................................ 268
Solving the Array Problem........................................... 269
Detecting Array Indexes...................................... 270
Increasing length When Adding New Elements...................... 270
Deleting Elements When Reducing length.......................... 272
Implementing the MyArray Class................................ 273
Using a Proxy as a Prototype......................................... 275
Using the get Trap on a Prototype ............................... 276
Using the set Trap on a Prototype ............................... 277
Using the has Trap on a Prototype............................... 278
Proxies as Prototypes on Classes................................ 279
Summary ...................................................... 282