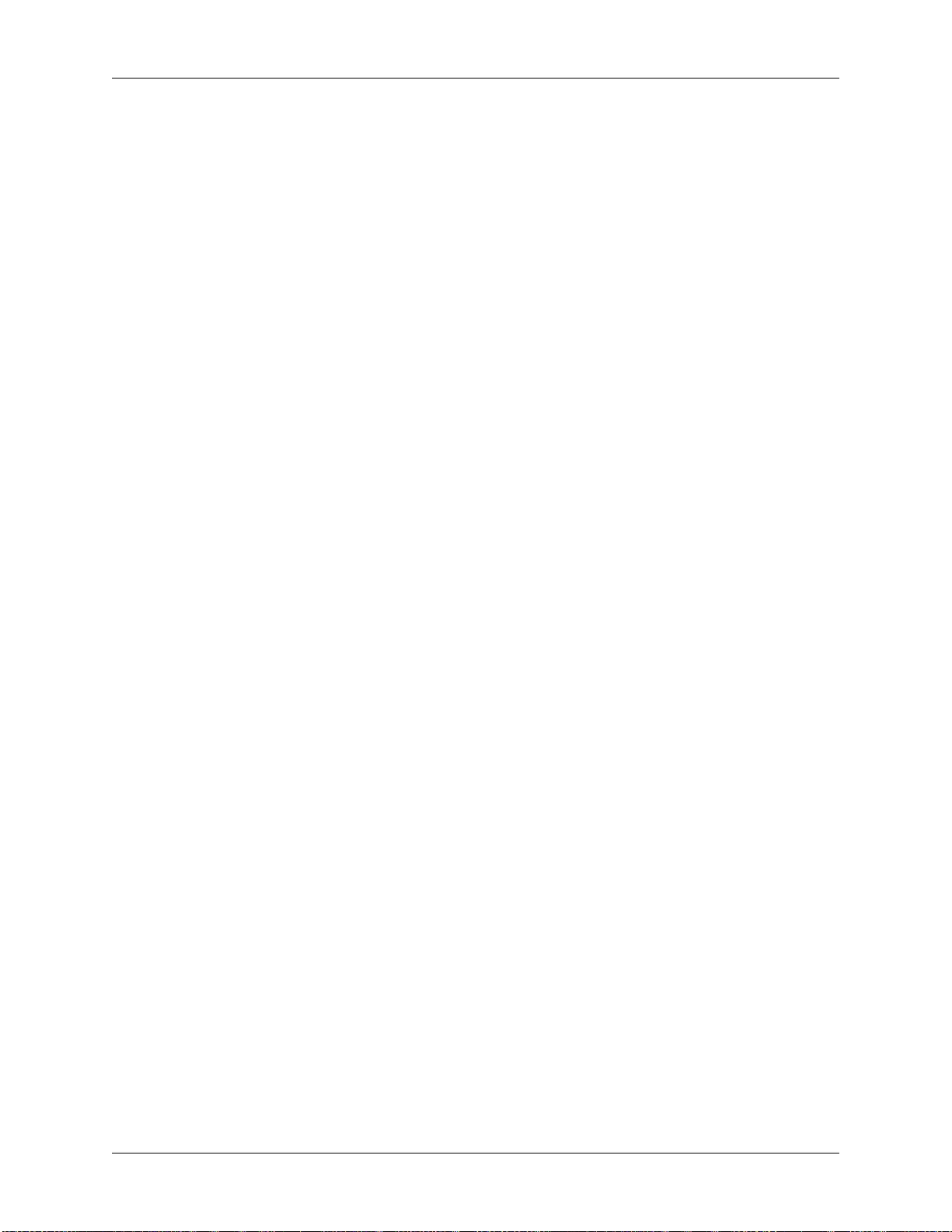
2
Table of Contents
1. Getting Started ................................................................................................................ 4
Chunks ...................................................................................................................... 4
Some Lexical Conventions ........................................................................................... 6
Global Variables ......................................................................................................... 7
Types and Values ........................................................................................................ 7
Nil .................................................................................................................... 8
Booleans ............................................................................................................ 8
The Stand-Alone Interpreter .......................................................................................... 9
2. Interlude: The Eight-Queen Puzzle .................................................................................... 12
3. Numbers ....................................................................................................................... 15
Numerals .................................................................................................................. 15
Arithmetic Operators .................................................................................................. 16
Relational Operators .................................................................................................. 17
The Mathematical Library ........................................................................................... 18
Random-number generator .................................................................................. 18
Rounding functions ............................................................................................ 18
Representation Limits ................................................................................................. 19
Conversions .............................................................................................................. 21
Precedence ............................................................................................................... 22
Lua Before Integers ................................................................................................... 22
4. Strings ......................................................................................................................... 24
Literal strings ............................................................................................................ 24
Long strings ............................................................................................................. 25
Coercions ................................................................................................................. 26
The String Library ..................................................................................................... 27
Unicode ................................................................................................................... 29
5. Tables .......................................................................................................................... 33
Table Indices ............................................................................................................ 33
Table Constructors ..................................................................................................... 35
Arrays, Lists, and Sequences ....................................................................................... 36
Table Traversal ......................................................................................................... 38
Safe Navigation ......................................................................................................... 38
The Table Library ..................................................................................................... 39
6. Functions ...................................................................................................................... 42
Multiple Results ........................................................................................................ 43
Variadic Functions ..................................................................................................... 45
The function table.unpack .................................................................................... 47
Proper Tail Calls ....................................................................................................... 48
7. The External World ........................................................................................................ 50
The Simple I/O Model ............................................................................................... 50
The Complete I/O Model ............................................................................................ 53
Other Operations on Files ........................................................................................... 54
Other System Calls .................................................................................................... 55
Running system commands ................................................................................. 55
8. Filling some Gaps .......................................................................................................... 57
Local Variables and Blocks ......................................................................................... 57
Control Structures ...................................................................................................... 58
if then else ....................................................................................................... 58
while ............................................................................................................... 59
repeat ............................................................................................................. 59
Numerical for ................................................................................................... 60