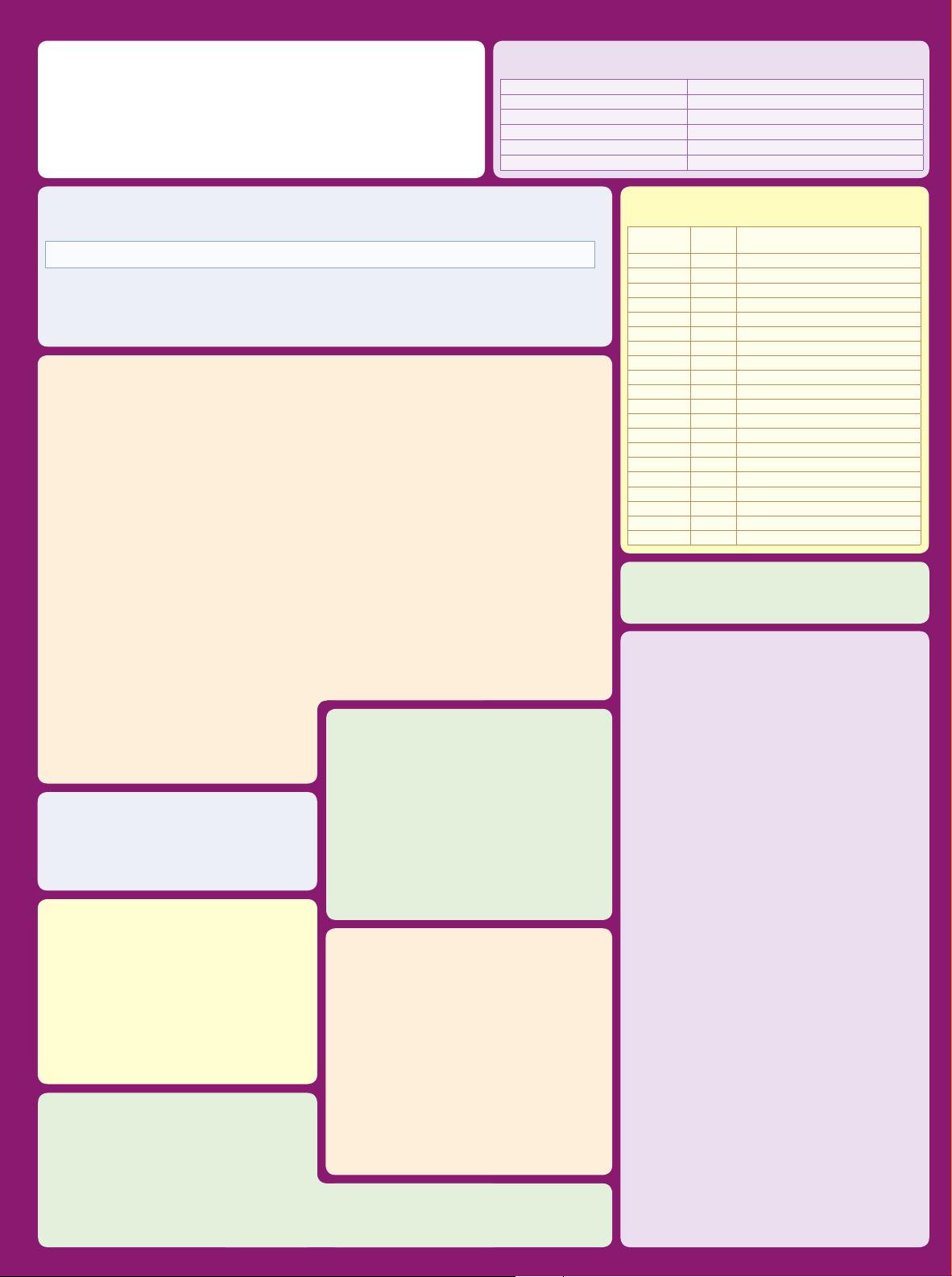
www.khronos.org/opengles©2012 Khronos Group - Rev. 0812
OpenGL ES 3.0 API Reference Card Page 1
Asynchronous Queries
[2.13, 6.1.7]
void GenQueries(sizei n, uint *ids);
void BeginQuery(enum target, uint id);
target: ANY_SAMPLES_PASSED{_CONSERVATIVE}
void EndQuery(enum target);
target: ANY_SAMPLES_PASSED{_CONSERVATIVE}
void DeleteQueries(sizei n, const uint *ids);
boolean IsQuery(uint id);
void GetQueryiv(enum target, enum pname,
int *params);
void GetQueryObjectuiv(uint id, enum pname,
uint *params);
Buer Objects
[2.9]
Buer objects hold vertex array data or indices in
high-performance server memory.
void GenBuers(sizei n, uint *buers);
void DeleteBuers(sizei n, const uint *buers);
Creang and Binding Buer Objects
void BindBuer(enum target, uint buer);
target: {ELEMENT_}ARRAY_BUFFER, PIXEL_{UN}PACK_BUFFER,
COPY_{READ, WRITE}_BUFFER, UNIFORM_BUFFER,
TRANSFORM_FEEDBACK_BUFFER
void BindBuerRange(enum target, uint index,
uint buer, intptr oset, sizeiptr size);
target: TRANSFORM_FEEDBACK_BUFFER, UNIFORM_BUFFER
void BindBuerBase(enum target, uint index, uint buer);
target: TRANSFORM_FEEDBACK_BUFFER, UNIFORM_BUFFER
Creang Buer Object Data Stores
void BuerData(enum target, sizeiptr size,
const void *data, enum usage);
target: See BindBuer
usage: {STATIC, STREAM, DYNAMIC}_{DRAW, READ, COPY}
void BuerSubData(enum target, intptr oset,
sizeiptr size, const void *data);
target: See BindBuer
Mapping and Unmapping Buer Data
void *MapBuerRange(enum target, intptr oset,
sizeiptr length, biield access);
target: See BindBuer
access: Bitwise OR of MAP_{READ, WRITE}_BIT,
MAP_INVALIDATE_{RANGE, BUFFER_BIT},
MAP_FLUSH_EXPLICIT_BIT, MAP_UNSYNCHRONIZED_BIT
void FlushMappedBuerRange(enum target,
intptr oset, sizeiptr length);
target: See BindBuer
boolean UnmapBuer(enum target);
target: See BindBuer
Copying Between Buers
void CopyBuerSubData(enum readtarget,
enum writetarget, intptr readoset, intptr writeoset,
sizeiptr size);
readtarget, writetarget: See target for BindBuer
Buer Object Queries [6.1.9]
boolean IsBuer(uint buer);
void GetBuerParameteriv(enum target, enum pname,
int * data);
target: See BindBuer
pname: BUFFER_{SIZE, USAGE, ACCESS_FLAGS, MAPPED},
BUFFER_ MAP_{POINTER, OFFSET, LENGTH}
void GetBuerParameteri64v(enum target,
enum pname, int64 *data);
target, pname: See
GetBuerParameteriv
void GetBuerPointerv(enum target, enum pname,
void **params);
target: See BindBuer
pname: BUFFER_ MAP_POINTER
OpenGL
®
ES is a soware interface to graphics hardware. The interface consists
of a set of procedures and funcons that allow a programmer to specify the
objects and operaons involved in producing high-quality graphical images,
specically color images of three-dimensional objects.
• [n.n.n] refers to secons and tables in the OpenGL ES 3.0 specicaon.
• [n.n.n] refers to secons in the OpenGL ES Shading Language 3.0 specicaon.
Specicaons are available at www.khronos.org/registry/gles/
OpenGL ES Command Syntax [2.3]
Open GL ES commands are formed from a return type, a name, and oponally a type leer: i for 32-bit int, i64 for int64,
f for 32-bit oat, or ui for 32-bit uint, as shown by the prototype below:
return-type Name{1234}{i i64 f ui}{v} ([args ,] T arg1 , . . . , T argN [, args]);
The arguments enclosed in brackets ([args ,] and [, args]) may or may not be present.
The argument type T and the number N of arguments may be indicated by the command name suxes. N is 1, 2, 3, or 4 if
present. If “v” is present, an array of N items is passed by a pointer.
For brevity, the OpenGL documentaon and this reference may omit the standard prexes.
The actual names are of the forms: glFunconName(), GL_CONSTANT, GLtype
Errors [2.5]
enum GetError(void);
//Returns one of the following:
NO_ERROR No error encountered
INVALID_ENUM Enum argument out of range
INVALID_VALUE Numeric argument out of range
INVALID_OPERATION Operaon illegal in current state
INVALID_FRAMEBUFFER_OPERATION Framebuer is incomplete
OUT_OF_MEMORY Not enough memory le to execute command
Viewport and Clipping
[2.12.1]
void DepthRangef(oat n, oat f);
void Viewport(int x, int y, sizei w, sizei h);
GL Data Types [2.3]
GL types are not C types.
GL Type
Minimum
Bit Width Descripon
boolean 1 Boolean
byte 8 Signed 2’s complement binary integer
ubyte 8 Unsigned binary integer
char 8 Characters making up strings
short 16 Signed 2’s complement binary integer
ushort 16 Unsigned binary integer
int 32 Signed 2’s complement binary integer
uint 32 Unsigned binary integer
int64 64 Signed 2’s complement binary integer
uint64 64 Unsigned binary integer
xed 32 Signed 2’s complement 16.16 scaled integer
sizei 32 Non-negave binary integer size
enum 32 Enumerated binary integer value
intptr ptrbits Signed 2’s complement binary integer
sizeiptr ptrbits Non-negave binary integer size
sync ptrbits Sync object handle
biield 32 Bit eld
half 16 Half-precision oat encoded in unsigned scalar
oat 32 Floang-point value
clampf 32 Floang-point value clamped to [0, 1]
Reading and Copying Pixels
[4.3.1-2]
void ReadPixels(int x, int y, sizei width, sizei height,
enum format, enum type, void *data);
format: RGBA, RGBA_INTEGER
type
: INT, UNSIGNED_INT_2_10_10_10_REV, UNSIGNED_{BYTE, INT}
Note: ReadPixels() also accepts a queriable implementaon-
chosen format/type combinaon [4.3.1]
.
void ReadBuer(enum src);
src: BACK, NONE, or COLOR_ATTACHMENT
i
where i may range
from zero to the value of MAX_COLOR_ATTACHMENTS - 1
void BlitFramebuer(int srcX0, int srcY0, int srcX1,
int srcY1, int dstX0, int dstY0, int dstX1, int dstY1,
biield mask, enum lter);
mask: Bitwise OR of {COLOR, DEPTH, STENCIL}_BUFFER_BIT
lter: LINEAR or NEAREST
Verces
Current Vertex State [2.7]
void VertexArib{1234}f(uint index, oat values);
void VertexArib{1234}fv(uint index, const oat *values);
void VertexAribl4{i ui}(uint index, T values);
void VertexAribl4{i ui}v(uint index, const T values);
Vertex Arrays [2.8]
Vertex data may be sourced from arrays stored in client’s address
space (via a pointer) or in server’s address space (in a buer object).
void VertexAribPointer(uint index, int size, enum type,
boolean normalized, sizei stride, const void *pointer);
type: {UNSIGNED_}BYTE, {UNSIGNED_}SHORT, {UNSIGNED_}INT, FIXED,
{HALF_}FLOAT, {UNSIGNED_}INT_2_10_10_10_REV
index: [0, MAX_VERTEX_ATTRIBS - 1]
void VertexAribIPointer(uint index, int size, enum type,
sizei stride, const void *pointer);
type: {UNSIGNED_}BYTE, {UNSIGNED_}SHORT, {UNSIGNED_}INT
index: [0, MAX_VERTEX_ATTRIBS - 1]
void EnableVertexAribArray(uint index);
void DisableVertexAribArray(uint index);
void VertexAribDivisor(uint index, uint divisor);
index: [0, MAX_VERTEX_ATTRIBS - 1]
void Enable(enum target);
void Disable(enum target);
target: PRIMITIVE_RESTART_FIXED_INDEX
Drawing [2.8.3]
void DrawArrays(enum mode, int rst, sizei count);
void DrawArraysInstanced(enum mode, int rst, sizei count,
sizei primcount);
void DrawElements(enum mode, sizei count, enum type,
const void *indices);
type: UNSIGNED_BYTE, UNSIGNED_SHORT, UNSIGNED_INT
void DrawElementsInstanced(enum mode, sizei count,
enum type, const void *indices, sizei primcount);
type: UNSIGNED_BYTE, UNSIGNED_SHORT, UNSIGNED_INT
void DrawRangeElements(enum mode, uint start, uint end,
sizei count, enum type, const void *indices);
mode: POINTS, TRIANGLES, LINES, LINE_{STRIP, LOOP}, TRIANGLE_STRIP,
TRIANGLE_FAN
type: UNSIGNED_BYTE, UNSIGNED_SHORT, UNSIGNED_INT
Vertex Array Objects
[2.10, 6.1.10]
void GenVertexArrays(sizei n, uint *arrays);
void DeleteVertexArrays(sizei n, const uint *arrays);
void BindVertexArray(uint array);
boolean IsVertexArray(uint array);
Rasterizaon [3]
Points [3.4]
Point size is taken from the shader built-in gl_PointSize and
clamped to the implementaon-dependent point size range.
Line Segments [3.5]
void LineWidth(oat width);
Polygons [3.6]
void FrontFace(enum dir);
dir: CCW, CW
void CullFace(enum mode);
mode: FRONT, BACK, FRONT_AND_BACK
Enable/Disable(CULL_FACE);
void PolygonOset(oat factor, oat units);
Enable/Disable(POLYGON_OFFSET_FILL);
Transform Feedback
[2.14, 6.1.11]
void GenTransformFeedbacks(sizei n, uint *ids);
void DeleteTransformFeedbacks(sizei n,
const uint *ids);
void BindTransformFeedback(enum target, uint id);
target: TRANSFORM_FEEDBACK
void BeginTransformFeedback(enum primiveMode);
primiveMode: TRIANGLES, LINES, POINTS
void EndTransformFeedback(void);
void PauseTransformFeedback(void);
void ResumeTransformFeedback(void);
boolean IsTransformFeedback(uint id);