C实现生产者和消费者代码
时间: 2023-10-17 12:05:33 浏览: 119
生产者和消费者是经典的多线程编程问题,下面是C语言实现生产者和消费者的代码:
```
#include <stdio.h>
#include <pthread.h>
#include <semaphore.h>
#define MAX_BUFFER_SIZE 10
int buffer[MAX_BUFFER_SIZE];
int count = 0;
int in = 0;
int out = 0;
sem_t empty;
sem_t full;
pthread_mutex_t mutex;
void *producer(void *arg)
{
int item;
while (1) {
item = rand() % 100;
sem_wait(&empty);
pthread_mutex_lock(&mutex);
buffer[in] = item;
in = (in + 1) % MAX_BUFFER_SIZE;
count++;
printf("Producer produced item %d, count = %d\n", item, count);
pthread_mutex_unlock(&mutex);
sem_post(&full);
sleep(rand() % 3);
}
}
void *consumer(void *arg)
{
int item;
while (1) {
sem_wait(&full);
pthread_mutex_lock(&mutex);
item = buffer[out];
out = (out + 1) % MAX_BUFFER_SIZE;
count--;
printf("Consumer consumed item %d, count = %d\n", item, count);
pthread_mutex_unlock(&mutex);
sem_post(&empty);
sleep(rand() % 3);
}
}
int main()
{
pthread_t producer_thread, consumer_thread;
sem_init(&empty, 0, MAX_BUFFER_SIZE);
sem_init(&full, 0, 0);
pthread_mutex_init(&mutex, NULL);
pthread_create(&producer_thread, NULL, producer, NULL);
pthread_create(&consumer_thread, NULL, consumer, NULL);
pthread_join(producer_thread, NULL);
pthread_join(consumer_thread, NULL);
sem_destroy(&empty);
sem_destroy(&full);
pthread_mutex_destroy(&mutex);
return 0;
}
```
该代码使用了信号量和互斥锁来实现多线程同步。其中,empty和full是两个信号量,用于控制缓冲区的状态;mutex是一个互斥锁,用于保护共享变量count、in和out的修改。生产者线程产生随机数并将其放入缓冲区中,消费者线程从缓冲区中取出随机数并消费。在每次操作之后,需要通过信号量和互斥锁来保证缓冲区状态的正确性和共享变量的原子性。
阅读全文
相关推荐
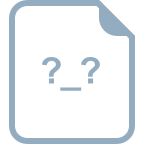
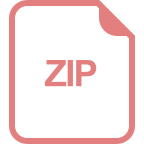
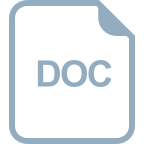
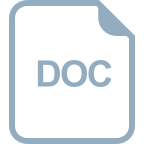
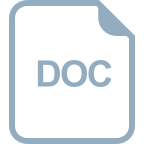











