Excel横向合并是A到H,纵向是1-5,Java如何获取合并表格里的数据
时间: 2024-05-06 07:19:37 浏览: 96
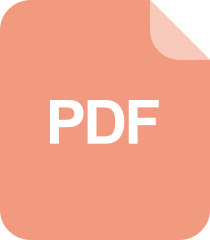
JAVA如何读取Excel数据
Java可以使用Apache POI库来读取Excel文件中的数据,其中包括合并单元格的数据。以下是一个示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelReader {
public static void main(String[] args) throws IOException {
String filePath = "path/to/excel/file.xlsx";
FileInputStream fileInputStream = new FileInputStream(new File(filePath));
Workbook workbook = WorkbookFactory.create(fileInputStream);
Sheet sheet = workbook.getSheetAt(0);
for (int i = 0; i < 5; i++) {
Row row = sheet.getRow(i);
for (int j = 0; j < 8; j++) {
Cell cell = row.getCell(j);
if (cell != null) {
if (cell.getCellType() == Cell.CELL_TYPE_FORMULA) {
System.out.print(cell.getCellFormula() + "\t");
} else if (cell.getCellType() == Cell.CELL_TYPE_NUMERIC) {
System.out.print(cell.getNumericCellValue() + "\t");
} else if (cell.getCellType() == Cell.CELL_TYPE_STRING) {
System.out.print(cell.getStringCellValue() + "\t");
} else if (cell.getCellType() == Cell.CELL_TYPE_BOOLEAN) {
System.out.print(cell.getBooleanCellValue() + "\t");
} else {
System.out.print("\t");
}
} else {
System.out.print("\t");
}
}
System.out.println();
}
workbook.close();
fileInputStream.close();
}
}
```
在这个示例中,我们打开Excel文件,获取第一个工作表(Sheet),然后循环遍历所有行和列。对于每个单元格,我们检查其单元格类型,并打印相应的值。
需要注意的是,当单元格被合并时,只有左上角的单元格中包含了数据,其他单元格中的数据为空。因此,在读取合并单元格时,我们需要注意只读取左上角的单元格。可以使用`CellRangeAddress`类来获取合并单元格的范围,然后只读取左上角的单元格。
```java
import org.apache.poi.ss.util.CellRangeAddress;
...
for (int i = 0; i < 5; i++) {
Row row = sheet.getRow(i);
for (int j = 0; j < 8; j++) {
Cell cell = row.getCell(j);
if (cell != null) {
if (cell.getCellType() == Cell.CELL_TYPE_FORMULA) {
System.out.print(cell.getCellFormula() + "\t");
} else if (cell.getCellType() == Cell.CELL_TYPE_NUMERIC) {
System.out.print(cell.getNumericCellValue() + "\t");
} else if (cell.getCellType() == Cell.CELL_TYPE_STRING) {
System.out.print(cell.getStringCellValue() + "\t");
} else if (cell.getCellType() == Cell.CELL_TYPE_BOOLEAN) {
System.out.print(cell.getBooleanCellValue() + "\t");
} else {
System.out.print("\t");
}
// Check if the cell is merged
CellRangeAddress range = getMergedRange(sheet, cell.getRowIndex(), cell.getColumnIndex());
if (range != null && range.getFirstRow() == cell.getRowIndex() && range.getFirstColumn() == cell.getColumnIndex()) {
// This is the left-top cell of the merged range
System.out.print("[Merged]");
}
} else {
System.out.print("\t");
}
}
System.out.println();
}
...
private static CellRangeAddress getMergedRange(Sheet sheet, int row, int column) {
for (CellRangeAddress range : sheet.getMergedRegions()) {
if (range.isInRange(row, column)) {
return range;
}
}
return null;
}
```
上面的代码中,我们添加了一个`getMergedRange`方法,用于获取包含指定行和列的合并单元格范围。如果单元格是合并的,我们会在输出中添加`[Merged]`标记。
阅读全文
相关推荐
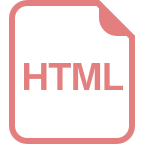
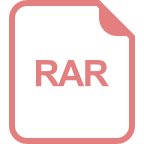
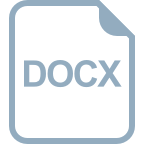
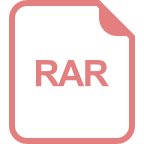
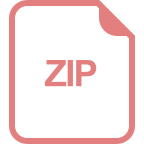
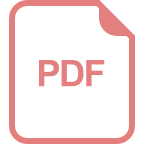
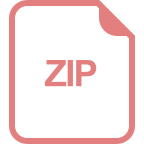
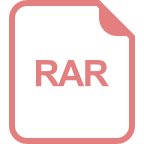
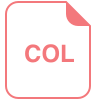

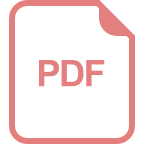
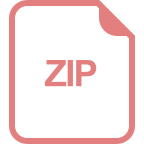
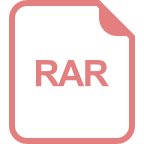
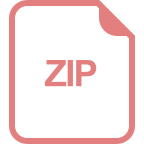