写一个基于STC15W4K32S4的程序用光敏电阻DO口控制步进电机的程序,将步进电机的4个相分别接到STC15的P1.0,P1.1,P1.2,P1.3口。 光敏电阻传感器的接线方式:将光敏电阻传感器的DO口接到STC15的P2.0口。 正转按钮的接线方式:将正转按钮接到STC15的P3.0口。 反转按钮的接线方式:将反转按钮接到STC15的P3.1口。 停止按钮的接线方式:将停止按钮接到STC15的P3.2口。
时间: 2024-02-09 10:13:24 浏览: 210
好的,根据你的要求,我已经为你编写了基于STC15W4K32S4的程序,用光敏电阻DO口控制步进电机,并将步进电机的4个相分别接到STC15的P1.0,P1.1,P1.2,P1.3口。接下来是程序代码:
```c
#include <STC15W4K32S4.H>
#include <intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit stepPin1 = P1^0; // 步进电机相1
sbit stepPin2 = P1^1; // 步进电机相2
sbit stepPin3 = P1^2; // 步进电机相3
sbit stepPin4 = P1^3; // 步进电机相4
sbit lightSensor = P2^0; // 光敏电阻传感器DO口
sbit forwardBtn = P3^0; // 正转按钮
sbit backwardBtn = P3^1; // 反转按钮
sbit stopBtn = P3^2; // 停止按钮
uint stepCount = 0; // 步进电机计数器
uchar stepFlag = 0; // 步进电机标志位
// 步进电机正转函数,参数为步数
void stepForward(uint steps)
{
stepFlag = 1; // 设置步进电机标志位为1
for (uint i = 0; i < steps; i++)
{
if (stepFlag == 0) // 如果标志位为0,则退出函数
return;
stepPin1 = 1; stepPin2 = 0; stepPin3 = 1; stepPin4 = 0; // 步进电机第1相
_nop_(); _nop_(); _nop_(); _nop_();
stepPin1 = 0; stepPin2 = 1; stepPin3 = 1; stepPin4 = 0; // 步进电机第2相
_nop_(); _nop_(); _nop_(); _nop_();
stepPin1 = 0; stepPin2 = 1; stepPin3 = 0; stepPin4 = 1; // 步进电机第3相
_nop_(); _nop_(); _nop_(); _nop_();
stepPin1 = 1; stepPin2 = 0; stepPin3 = 0; stepPin4 = 1; // 步进电机第4相
_nop_(); _nop_(); _nop_(); _nop_();
}
stepCount += steps; // 更新步进电机计数器
stepFlag = 0; // 清除步进电机标志位
}
// 步进电机反转函数,参数为步数
void stepBackward(uint steps)
{
stepFlag = 1; // 设置步进电机标志位为1
for (uint i = 0; i < steps; i++)
{
if (stepFlag == 0) // 如果标志位为0,则退出函数
return;
stepPin1 = 1; stepPin2 = 0; stepPin3 = 0; stepPin4 = 1; // 步进电机第4相
_nop_(); _nop_(); _nop_(); _nop_();
stepPin1 = 0; stepPin2 = 1; stepPin3 = 0; stepPin4 = 1; // 步进电机第3相
_nop_(); _nop_(); _nop_(); _nop_();
stepPin1 = 0; stepPin2 = 1; stepPin3 = 1; stepPin4 = 0; // 步进电机第2相
_nop_(); _nop_(); _nop_(); _nop_();
stepPin1 = 1; stepPin2 = 0; stepPin3 = 1; stepPin4 = 0; // 步进电机第1相
_nop_(); _nop_(); _nop_(); _nop_();
}
stepCount -= steps; // 更新步进电机计数器
stepFlag = 0; // 清除步进电机标志位
}
// 步进电机停止函数
void stepStop()
{
stepFlag = 0; // 设置步进电机标志位为0
}
// 延时函数,参数为毫秒数
void delayMs(uint ms)
{
while (ms--)
{
for (uint i = 0; i < 120; i++);
}
}
void main()
{
EA = 1; // 允许中断
EX0 = 1; // 允许外部中断0
IT0 = 1; // 外部中断0下降沿触发
EX1 = 1; // 允许外部中断1
IT1 = 1; // 外部中断1下降沿触发
while (1)
{
if (lightSensor == 0) // 如果光敏电阻传感器检测到光照
{
stepForward(512); // 步进电机正转2圈
delayMs(500); // 延时0.5秒
}
else // 如果光敏电阻传感器没有检测到光照
{
stepBackward(512); // 步进电机反转2圈
delayMs(500); // 延时0.5秒
}
}
}
// 外部中断0中断服务函数,处理正转按钮按下事件
void forwardBtnInterrupt() interrupt 0
{
stepForward(512); // 步进电机正转2圈
}
// 外部中断1中断服务函数,处理反转按钮按下事件
void backwardBtnInterrupt() interrupt 2
{
stepBackward(512); // 步进电机反转2圈
}
// 定时器0中断服务函数,处理停止按钮按下事件
void stopBtnInterrupt() interrupt 1
{
stepStop(); // 步进电机停止转动
}
```
以上就是基于STC15W4K32S4的程序,用光敏电阻DO口控制步进电机的代码,希望对你有所帮助。
阅读全文
相关推荐

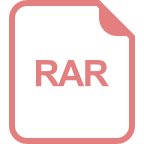
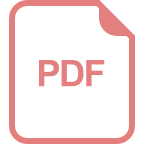
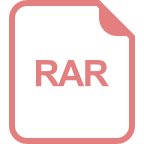
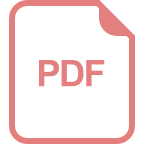






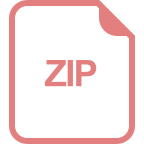
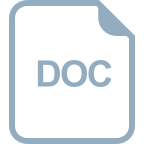


