帮我写一程序,用C语言写,实现的功能包括创建二叉树的二叉链表,实现二叉树的3种遍历递归算法并分别打印
时间: 2023-11-22 14:55:59 浏览: 39
好的,以下是使用 C 语言实现创建二叉树的二叉链表,实现二叉树的3种遍历递归算法并分别打印的程序:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树节点结构体
typedef struct TreeNode {
char data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 创建二叉树
TreeNode* createTree() {
char c;
TreeNode *root = NULL;
scanf("%c", &c);
if (c == '#') {
root = NULL;
} else {
root = (TreeNode*)malloc(sizeof(TreeNode));
root->data = c;
root->left = createTree();
root->right = createTree();
}
return root;
}
// 前序遍历
void preOrder(TreeNode *root) {
if (root != NULL) {
printf("%c ", root->data); // 打印节点数据
preOrder(root->left); // 遍历左子树
preOrder(root->right); // 遍历右子树
}
}
// 中序遍历
void inOrder(TreeNode *root) {
if (root != NULL) {
inOrder(root->left); // 遍历左子树
printf("%c ", root->data); // 打印节点数据
inOrder(root->right); // 遍历右子树
}
}
// 后序遍历
void postOrder(TreeNode *root) {
if (root != NULL) {
postOrder(root->left); // 遍历左子树
postOrder(root->right); // 遍历右子树
printf("%c ", root->data); // 打印节点数据
}
}
int main() {
printf("请输入二叉树的前序遍历序列(#代表空节点):\n");
TreeNode *root = createTree(); // 创建二叉树
printf("前序遍历结果为:");
preOrder(root); // 前序遍历
printf("\n中序遍历结果为:");
inOrder(root); // 中序遍历
printf("\n后序遍历结果为:");
postOrder(root); // 后序遍历
printf("\n");
return 0;
}
```
该程序通过递归的方式实现了创建二叉树的二叉链表,以及前序遍历、中序遍历、后序遍历三种遍历算法,并将遍历结果打印输出。
相关推荐
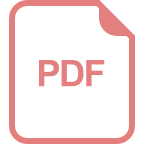
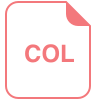
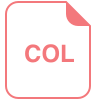
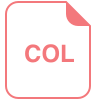
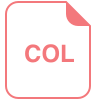
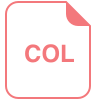









