c语言 按先序遍历序列建立一个二叉树的二叉链表,并统计二叉树中叶子结点个数和二叉树的深度。
时间: 2024-01-23 22:41:10 浏览: 27
以下是C语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
char data;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 先序遍历建立二叉树
TreeNode* createTree() {
char c;
scanf("%c", &c);
if (c == '#') {
return NULL;
}
TreeNode *node = (TreeNode*)malloc(sizeof(TreeNode));
node->data = c;
node->left = createTree();
node->right = createTree();
return node;
}
// 统计叶子节点个数
int countLeaf(TreeNode *root) {
if (root == NULL) {
return 0;
}
if (root->left == NULL && root->right == NULL) {
return 1;
}
return countLeaf(root->left) + countLeaf(root->right);
}
// 计算树的深度
int getDepth(TreeNode *root) {
if (root == NULL) {
return 0;
}
int leftDepth = getDepth(root->left);
int rightDepth = getDepth(root->right);
return leftDepth > rightDepth ? leftDepth + 1 : rightDepth + 1;
}
int main() {
TreeNode *root = createTree();
int leafCount = countLeaf(root);
int depth = getDepth(root);
printf("叶子结点个数:%d\n", leafCount);
printf("二叉树的深度:%d\n", depth);
return 0;
}
```
输入的先序遍历序列中,`#`表示空节点。在建立二叉树的过程中,如果遇到空节点则返回`NULL`。统计叶子节点个数的函数中,如果当前节点为叶子节点,则返回1,否则递归统计左右子树的叶子节点个数。计算树的深度的函数中,先递归计算左右子树的深度,然后比较左右子树深度的大小,取较大值再加上1即为整棵树的深度。
相关推荐
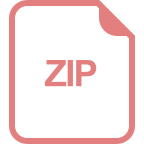
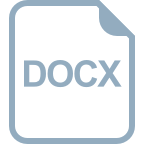














