请用C语言编程:按先序遍历序列建立一个二叉树的二叉链表,并统计二叉树中叶子结点个数和二叉树的深度。
时间: 2024-01-23 14:45:37 浏览: 98
以下是C语言实现,其中假设二叉树的节点结构体为`TreeNode`,包含左右子节点指针和节点值。另外,为了方便统计叶子结点个数和深度,我们在函数返回时将这两个值存储在`result`数组中,`result[0]`表示叶子结点个数,`result[1]`表示深度。
```
#include <stdio.h>
#include <stdlib.h>
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
void buildTree(TreeNode **root) {
int val;
scanf("%d", &val);
if (val == -1) {
*root = NULL;
} else {
*root = (TreeNode *) malloc(sizeof(TreeNode));
(*root)->val = val;
buildTree(&((*root)->left));
buildTree(&((*root)->right));
}
}
void countLeafAndDepth(TreeNode *root, int depth, int result[]) {
if (root == NULL) {
return;
}
if (root->left == NULL && root->right == NULL) {
result[0]++;
if (depth > result[1]) {
result[1] = depth;
}
return;
}
countLeafAndDepth(root->left, depth + 1, result);
countLeafAndDepth(root->right, depth + 1, result);
}
int main() {
TreeNode *root;
buildTree(&root);
int result[2] = {0};
countLeafAndDepth(root, 1, result);
printf("叶子结点个数:%d\n", result[0]);
printf("二叉树深度:%d\n", result[1]);
return 0;
}
```
阅读全文
相关推荐
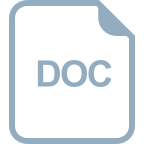
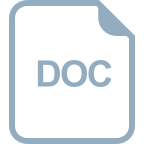
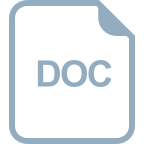















