用python编写基于pid控制倒立摆的代码
时间: 2024-05-16 19:15:31 浏览: 12
好的,下面是基于PID控制的倒立摆的Python代码。需要注意的是,这个代码是一个简单的模拟,其中的参数可能需要根据实际情况进行调整。
```python
import math
import numpy as np
import matplotlib.pyplot as plt
# 倒立摆模拟参数
m_cart = 1.0 # 小车质量
m_pole = 0.1 # 杆的质量
pole_length = 1.0 # 杆的长度
g = 9.81 # 重力加速度
# PID控制器参数
kp = 100.0 # 比例系数
ki = 50.0 # 积分系数
kd = 20.0 # 微分系数
# 仿真参数
dt = 0.01 # 仿真步长
total_time = 10.0 # 总仿真时间
# 初始化状态
x = 0.0 # 小车位置
theta = math.pi # 杆的初始角度
x_dot = 0.0 # 小车速度
theta_dot = 0.0 # 杆的角速度
# 记录仿真结果的数组
t = np.arange(0.0, total_time, dt)
x_arr = np.zeros_like(t)
theta_arr = np.zeros_like(t)
x_dot_arr = np.zeros_like(t)
theta_dot_arr = np.zeros_like(t)
force_arr = np.zeros_like(t)
# PID控制器的误差积分项
error_sum = 0.0
last_error = 0.0
# 开始仿真
for i in range(len(t)):
# 计算PID控制器的输出
error = -theta # 当前误差
error_sum += error * dt # 误差积分项
error_diff = (error - last_error) / dt # 误差微分项
force = kp * error + ki * error_sum + kd * error_diff # 控制力
last_error = error
# 记录控制力
force_arr[i] = force
# 计算小车和杆的加速度
cos_theta = math.cos(theta)
sin_theta = math.sin(theta)
temp = (force + m_pole * sin_theta * (pole_length * theta_dot ** 2 + g * cos_theta)) / (m_cart + m_pole * sin_theta ** 2)
x_acceleration = temp - m_pole * cos_theta * sin_theta * theta_dot ** 2 / (m_cart + m_pole * sin_theta ** 2)
theta_acceleration = (g * sin_theta - cos_theta * x_acceleration) / pole_length
# 更新小车和杆的状态
x += x_dot * dt
theta += theta_dot * dt
x_dot += x_acceleration * dt
theta_dot += theta_acceleration * dt
# 记录状态
x_arr[i] = x
theta_arr[i] = theta
x_dot_arr[i] = x_dot
theta_dot_arr[i] = theta_dot
# 绘制结果
plt.figure(figsize=(10, 6))
plt.subplot(221)
plt.plot(t, x_arr)
plt.xlabel('Time (s)')
plt.ylabel('Cart Position (m)')
plt.grid()
plt.subplot(222)
plt.plot(t, theta_arr)
plt.xlabel('Time (s)')
plt.ylabel('Pole Angle (rad)')
plt.grid()
plt.subplot(223)
plt.plot(t, x_dot_arr)
plt.xlabel('Time (s)')
plt.ylabel('Cart Velocity (m/s)')
plt.grid()
plt.subplot(224)
plt.plot(t, theta_dot_arr)
plt.xlabel('Time (s)')
plt.ylabel('Pole Angular Velocity (rad/s)')
plt.grid()
plt.tight_layout()
plt.show()
```
希望对你有所帮助!
相关推荐
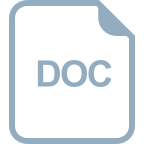
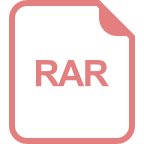












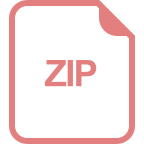
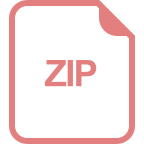