用python写一段建立一阶直线倒立摆模型的代码
时间: 2023-09-08 22:04:42 浏览: 66
### 回答1:
以下是用 Python 编写的一阶直线倒立摆模型的代码。
```python
import numpy as np
import matplotlib.pyplot as plt
# 模型参数
M = 1 # 摆杆质量
m = 0.1 # 小球质量
L = 1 # 摆杆长度
g = 9.8 # 重力加速度
# 定义状态向量 x = [theta, dtheta, x, dx]
# theta - 摆杆的倾角
# dtheta - 摆杆的角速度
# x - 小球的水平位置
# dx - 小球的水平速度
# 初始化状态向量
x0 = np.array([np.pi/6, 0, 0, 0])
# 控制输入向量 u = F
# F - 小球的水平受力
# 初始化控制输入向量
u0 = np.array([0])
# 定义状态函数 f(x, u)
def f(x, u):
theta, dtheta, x, dx = x
sin_theta = np.sin(theta)
cos_theta = np.cos(theta)
num1 = m*L*sin_theta*(dtheta**2) + u*cos_theta - m*g*sin_theta*cos_theta
den1 = M + m*(1 - cos_theta**2)
num2 = (M + m)*g*sin_theta - u - m*L*sin_theta*(dtheta**2)*cos_theta
den2 = L*(M + m*(1 - cos_theta**2))
dtheta_dot = num1 / den1
dx_dot = num2 / den2
return np.array([dtheta, dtheta_dot, dx, dx_dot])
# 定义欧拉法离散化函数
def euler_discretization(x, u, f, dt):
x_next = x + f(x, u)*dt
return x_next
# 模拟函数
def simulate(x0, u0, f, dt, T):
timesteps = int(T/dt)
x_hist = np.zeros((timesteps+1, x0.shape[0]))
x_hist[0] = x0
u_hist = np.zeros((timesteps, u0.shape[0]))
u_hist[0] = u0
for i in range(1, timesteps+1):
x_next = euler_discretization(x_hist[i-1], u_hist[i-1], f, dt)
x_hist[i] = x_next
if i < timesteps:
u_hist[i] = u0 # 控制输入不变
return x_hist, u_hist
# 参数设置
dt = 0.01 # 时间步长
T = 10 # 模拟时长
# 运行模拟
x_hist, u_hist = simulate(x0, u0, f, dt, T)
# 绘图
plt.figure()
plt.plot(np.arange(0, T+dt, dt), x_hist[:, 0])
plt.title('Inverted Pendulum')
plt.xlabel('Time (s)')
plt.ylabel('Theta (rad)')
plt.show()
```
可以看到,这段代码使用欧拉法离散化方法,模拟了一阶直线倒立摆模型,并绘制了摆杆的倾角随时间的变化。
### 回答2:
要用Python编写一段代码来建立一阶直线倒立摆模型,可以按照以下步骤进行:
1. 导入所需的库:numpy、matplotlib等。
2. 定义模型的参数,包括摆杆的长度、质量以及重力加速度等。
3. 定义模型的初始条件,包括初始角度和角速度等。
4. 定义模型的微分方程,即倒立摆的动力学方程。可以使用欧拉方法或其他数值积分方法对微分方程进行离散化处理。
5. 定义模型的控制策略,比如使用PID控制器或LQR控制器等。
6. 根据控制策略,计算控制输入,即施加在摆杆上的力或力矩。
7. 使用数值积分方法对微分方程进行数值求解,得到下一个时间步的角度和角速度。
8. 循环进行步骤6和步骤7,直到达到所需的仿真时间或满足停止条件。
9. 可选地,通过绘制图表来可视化模型的运动过程,如倒立摆的角度随时间的变化曲线。
总之,通过使用适当的库和数值方法,结合适当的控制策略,可以用Python编写出一段建立一阶直线倒立摆模型的代码。
相关推荐
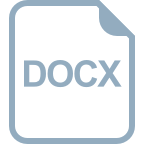

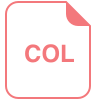
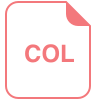
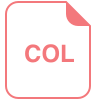
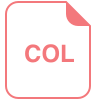









