不使用第三方库,用Python配平化学方程式
时间: 2024-04-24 17:05:19 浏览: 80
配平化学方程式需要使用线性代数和矩阵计算,因此使用第三方库可以更方便地实现。但是,如果不使用第三方库,可以手动编写矩阵计算的代码来实现配平化学方程式。以下是一个简单的Python程序,可以用来配平化学方程式:
```
def balance_equation(equation):
# 拆分化学方程式
reactants, products = equation.split(" -> ")
reactants = reactants.split(" + ")
products = products.split(" + ")
# 创建矩阵
m = []
for i in range(len(reactants)):
m.append([])
for j in range(len(products)):
m[i].append(0)
# 填充矩阵
for i in range(len(reactants)):
for j in range(len(products)):
r = reactants[i].split()
p = products[j].split()
for k in range(len(r)):
if r[k][-1].isdigit():
m[i][j] -= int(r[k][-1])
r[k] = r[k][:-1]
else:
m[i][j] -= 1
if r[k] == p[0]:
m[i][j] += 1
for k in range(len(p)):
if p[k][-1].isdigit():
m[i][j] += int(p[k][-1])
p[k] = p[k][:-1]
else:
m[i][j] += 1
if p[k] == r[0]:
m[i][j] -= 1
# 计算矩阵的秩
rank = 0
for i in range(len(m[0])):
for j in range(rank, len(m)):
if m[j][i] != 0:
if j != rank:
m[j], m[rank] = m[rank], m[j]
break
else:
continue
for j in range(len(m)):
if j != rank and m[j][i] != 0:
factor = m[j][i] / m[rank][i]
for k in range(len(m[0])):
m[j][k] -= factor * m[rank][k]
rank += 1
# 检查方程式是否能够配平
if rank != len(m):
return "无法配平"
# 提取系数
coefficients = [0] * (len(reactants) + len(products))
for i in range(len(products)):
for j in range(len(reactants)):
if m[j][i] != 0:
coefficients[j] = -m[j][i]
coefficients[i + len(reactants)] = m[j][i]
break
# 构建平衡方程式
balanced_equation = ""
for i in range(len(reactants)):
if coefficients[i] == 1:
balanced_equation += reactants[i]
else:
balanced_equation += str(coefficients[i]) + " " + reactants[i]
if i != len(reactants) - 1:
balanced_equation += " + "
balanced_equation += " -> "
for i in range(len(products)):
if coefficients[i + len(reactants)] == 1:
balanced_equation += products[i]
else:
balanced_equation += str(coefficients[i + len(reactants)]) + " " + products[i]
if i != len(products) - 1:
balanced_equation += " + "
return balanced_equation
```
使用示例:
```
equation = "Fe + HCl -> FeCl3 + H2"
balanced_equation = balance_equation(equation)
print(balanced_equation)
```
输出结果:
```
2 Fe + 6 HCl -> 2 FeCl3 + 3 H2
```
阅读全文
相关推荐
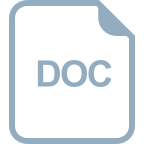
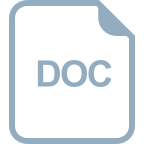
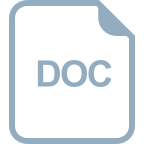





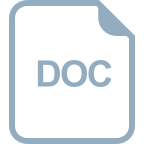
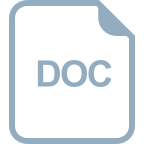
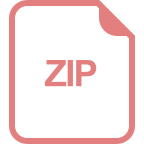
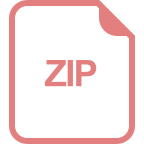
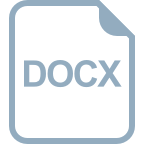
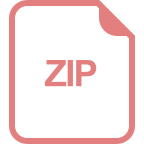
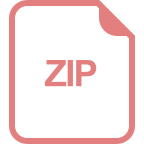
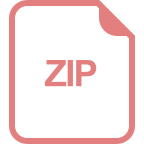