c++将数组中重复的字符去除
时间: 2023-06-05 16:47:25 浏览: 246
如果要将数组中重复的字符去除,我们可以采用以下步骤:
1. 定义一个空的新数组,用来存储去重后的字符。
2. 使用循环遍历原数组中的每个字符,判断这个字符是否已经在新数组中存在。
3. 如果这个字符没有在新数组中存在,就将它添加到新数组中。
4. 如果这个字符已经在新数组中存在,就跳过这个字符,继续往下循环。
5. 完成循环后,新数组中就存储了原数组中去重后的字符。
看一个示例:
假设原数组为:[‘a’,‘b’,‘c’,‘c’,‘d’,‘e’,‘e’,‘e’]
我们可以这样去重:
```python
arr = ['a','b','c','c','d','e','e','e'] # 原数组
result = [] # 新数组
for i in arr:
if i not in result: # 判断i是否在新数组中出现
result.append(i) # 如果没有出现,就将i添加到新数组中
print(result) # 输出结果:[‘a’,‘b’,‘c’,‘d’,‘e’]
```
这样就完成了数组中字符的去重。
相关问题
c++字符数组怎么输入
可以使用scanf函数或者fgets函数输入字符数组。例如:
使用scanf函数:
```c
char str[100];
scanf("%s", str);
```
使用fgets函数:
```c
char str[100];
fgets(str, 100, stdin);
```
需要注意的是,fgets函数会读入换行符,所以需要去除换行符。可以使用下面的代码:
```c
fgets(str, 100, stdin);
str[strcspn(str, "\n")] = '\0';
```
arduino 去掉字符串数组中的字符
要从字符串数组中去掉特定的字符,可以使用C++中的`std::remove`函数。这个函数将要删除的字符移动到数组的末尾,并返回一个指向新的末尾位置的迭代器。然后,可以使用`std::string`的`resize`方法将数组的大小缩小到新的末尾位置。
以下是一个示例代码,从字符串数组中删除所有的字母'a':
```c++
#include <algorithm>
#include <string>
int main() {
char arr[] = "hello world";
std::string str(arr);
auto new_end = std::remove(str.begin(), str.end(), 'a');
str.resize(std::distance(str.begin(), new_end));
// 输出结果
for (char c : str) {
Serial.print(c);
}
}
```
以上代码将输出字符串"hello world"中所有'a'字母被删除后的结果。
阅读全文
相关推荐
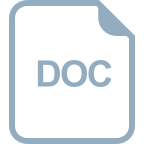





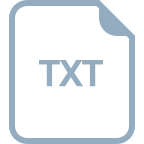
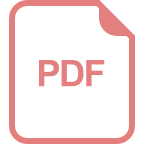




