基于tensorflow中的keras编写代码,数据目录 rawdata 1. 编写数据加载函数; 2. 根据任务要求对数据集进行划分; 3. 数据增强; 4. 构建深度学习模型和损失函数; 5. 编写模型训练相关代码,完成模型训练 6. 使用可视化库 Matplotlib 对训练过程进行可视化展示,如损失值变化、准确率变化、召回率、ROC曲线等。 7. 将训练好的模型保存
时间: 2023-11-28 19:50:27 浏览: 69
下面是一个基于tensorflow中的keras编写的代码示例,以MNIST数据集为例:
1. 数据加载函数:
```python
import tensorflow as tf
def load_data():
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1).astype('float32') / 255
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1).astype('float32') / 255
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
return x_train, y_train, x_test, y_test
```
2. 数据集划分:
```python
x_train, y_train, x_test, y_test = load_data()
x_train, x_valid = x_train[:50000], x_train[50000:]
y_train, y_valid = y_train[:50000], y_train[50000:]
```
3. 数据增强:
```python
datagen = tf.keras.preprocessing.image.ImageDataGenerator(rotation_range=10, width_shift_range=0.1, height_shift_range=0.1, shear_range=0.1, zoom_range=0.1, fill_mode='nearest')
datagen.fit(x_train)
```
4. 构建模型和损失函数:
```python
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), padding='same', activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Dropout(0.25),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dropout(0.5),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
```
5. 模型训练:
```python
history = model.fit_generator(datagen.flow(x_train, y_train, batch_size=32), epochs=10, validation_data=(x_valid, y_valid))
```
6. 可视化展示:
```python
import matplotlib.pyplot as plt
plt.plot(history.history['loss'], label='train_loss')
plt.plot(history.history['val_loss'], label='val_loss')
plt.plot(history.history['accuracy'], label='train_acc')
plt.plot(history.history['val_accuracy'], label='val_acc')
plt.legend()
plt.show()
```
7. 模型保存:
```python
model.save('my_model.h5')
```
阅读全文
相关推荐
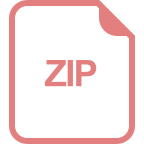
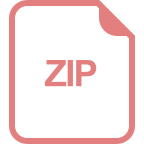
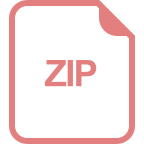
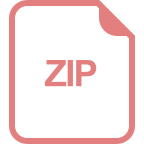
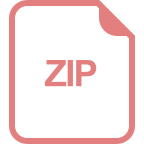
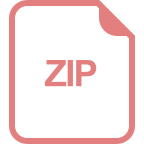
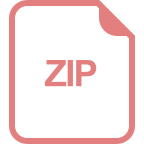
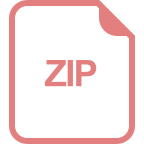
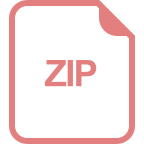
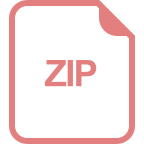
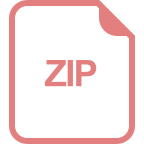
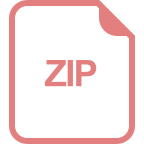
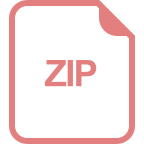
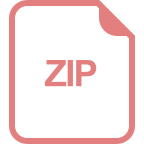
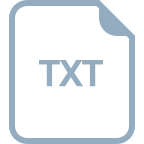
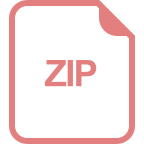
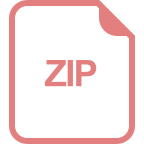