python orb方法提取图像特征点 并 用四叉树方法将提取到的特征带你均匀化
时间: 2024-05-11 20:18:42 浏览: 343
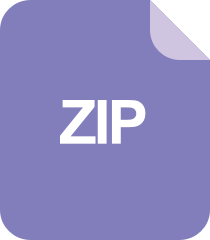
图像匹配、图像特征提取、图像配准、误匹配剔除+ORB-RANSAC
好的,我来为您讲解一下。
首先,ORB是一种特征点检测和描述算法,可以用于图像匹配、目标跟踪等应用。
使用OpenCV库中的ORB函数可以提取图像特征点,示例代码如下:
```python
import cv2
# 读取图像
img = cv2.imread('image.jpg', 0)
# 初始化ORB检测器
orb = cv2.ORB_create()
# 检测图像特征点
kp = orb.detect(img, None)
# 计算特征点描述子
kp, des = orb.compute(img, kp)
# 绘制特征点
img_with_kp = cv2.drawKeypoints(img, kp, None, color=(0, 255, 0), flags=0)
# 显示图像
cv2.imshow('image', img_with_kp)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
接下来,我们可以使用四叉树方法将提取到的特征点均匀化。四叉树方法是一种空间分割方法,可以将空间划分为四个象限,从而实现对空间的均匀划分。
示例代码如下:
```python
import numpy as np
# 定义四叉树节点类
class Node:
def __init__(self, x, y, w, h):
self.x = x
self.y = y
self.w = w
self.h = h
self.children = []
self.kp = []
# 判断是否为叶子节点
def is_leaf(self):
return len(self.children) == 0
# 判断点是否在当前节点内
def contains(self, kp):
return kp.pt[0] >= self.x and kp.pt[0] <= self.x + self.w and kp.pt[1] >= self.y and kp.pt[1] <= self.y + self.h
# 定义四叉树类
class QuadTree:
def __init__(self, max_depth=4, max_kp=4):
self.max_depth = max_depth
self.max_kp = max_kp
self.root = None
# 插入特征点
def insert(self, kp):
if self.root is None:
x, y, w, h = kp.pt[0], kp.pt[1], 512, 512
self.root = Node(x, y, w, h)
else:
self._insert(kp, self.root, 0)
def _insert(self, kp, node, depth):
if node.is_leaf():
node.kp.append(kp)
# 如果叶子结点中的特征点超过了最大值,则进行划分
if len(node.kp) > self.max_kp and depth < self.max_depth:
self._split(node, depth)
else:
# 如果当前节点不是叶子节点,则递归插入到子节点
for child in node.children:
if child.contains(kp):
self._insert(kp, child, depth+1)
break
# 划分节点
def _split(self, node, depth):
x, y, w, h = node.x, node.y, node.w/2, node.h/2
node.children.append(Node(x, y, w, h))
node.children.append(Node(x+w, y, w, h))
node.children.append(Node(x, y+h, w, h))
node.children.append(Node(x+w, y+h, w, h))
# 将特征点插入到子节点中
for kp in node.kp:
for child in node.children:
if child.contains(kp):
self._insert(kp, child, depth+1)
break
node.kp = []
# 获取特定区域内的特征点
def get_kp_in_region(self, region):
kp_list = []
self._get_kp_in_region(self.root, region, kp_list)
return kp_list
def _get_kp_in_region(self, node, region, kp_list):
if node.is_leaf():
for kp in node.kp:
if region.contains(kp):
kp_list.append(kp)
else:
for child in node.children:
if region.intersects(child):
self._get_kp_in_region(child, region, kp_list)
```
接下来,我们可以将提取到的特征点插入到四叉树中,并使用特定区域内的特征点进行均匀化处理。示例代码如下:
```python
# 插入特征点到四叉树中
qt = QuadTree()
for k in kp:
qt.insert(k)
# 定义均匀化区域
region = Rect(200, 200, 100, 100)
# 获取区域内的特征点
kp_list = qt.get_kp_in_region(region)
# 计算区域内的特征点平均位置
x = np.mean([kp.pt[0] for kp in kp_list])
y = np.mean([kp.pt[1] for kp in kp_list])
# 将区域中的特征点移动到平均位置
for kp in kp_list:
kp.pt = (x, y)
```
最后,我们可以将均匀化后的特征点绘制出来,示例代码如下:
```python
# 绘制均匀化后的特征点
img_with_kp_uniform = cv2.drawKeypoints(img, kp, None, color=(0, 255, 0), flags=0)
# 显示图像
cv2.imshow('image with keypoints', img_with_kp_uniform)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
希望能对您有所帮助!
阅读全文
相关推荐
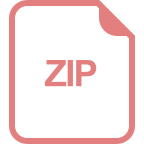












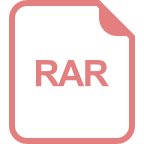
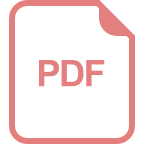
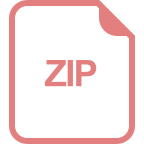
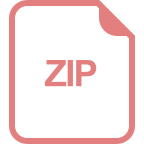