python 提取图像特征点 并 均匀化特征点
时间: 2023-07-27 12:19:51 浏览: 70
要提取图像特征点,可以使用一些经典的算法,比如 SIFT、SURF、ORB 等。这些算法可以在不同的场景中提取出代表性的特征点,这些特征点可以用于图像匹配、图像分类等任务。
下面以 SIFT 算法为例,介绍一下如何提取图像特征点并均匀化特征点。
1. 提取图像特征点
使用 OpenCV 库中的 SIFT 算法可以提取图像的关键点和特征描述符。下面是一个简单的示例代码:
```python
import cv2
# 读取图像
img = cv2.imread('test.jpg')
# 创建 SIFT 对象
sift = cv2.xfeatures2d.SIFT_create()
# 检测关键点和特征描述符
keypoints, descriptors = sift.detectAndCompute(img, None)
# 在图像上绘制关键点
img_with_keypoints = cv2.drawKeypoints(img, keypoints, None)
# 显示图像
cv2.imshow('img', img_with_keypoints)
cv2.waitKey()
```
2. 均匀化特征点
在实际任务中,我们可能需要均匀化特征点的分布,以便于更好地进行图像匹配等操作。一种方法是使用 k-means 算法对特征点进行聚类,然后选择每个簇中距离中心点最近的特征点作为代表点。下面是一个简单的示例代码:
```python
import numpy as np
from sklearn.cluster import KMeans
# 假设有 100 个特征点
n_features = 100
# 生成随机特征点坐标
keypoints = np.random.rand(n_features, 2) * 100
# 将特征点坐标转换为整数类型
keypoints = keypoints.astype(np.int32)
# 使用 k-means 算法对特征点进行聚类
n_clusters = 10
kmeans = KMeans(n_clusters=n_clusters)
kmeans.fit(keypoints)
# 找到每个簇中距离中心点最近的特征点
new_keypoints = []
for i in range(n_clusters):
cluster_points = keypoints[kmeans.labels_ == i]
center = kmeans.cluster_centers_[i]
dists = np.sqrt(np.sum((cluster_points - center) ** 2, axis=1))
min_idx = np.argmin(dists)
new_keypoints.append(cluster_points[min_idx])
# 显示均匀化后的特征点
img = np.zeros((100, 100), dtype=np.uint8)
for pt in new_keypoints:
img[pt[1], pt[0]] = 255
cv2.imshow('img', img)
cv2.waitKey()
```
上述代码中,首先生成了 100 个随机特征点坐标,然后使用 k-means 算法将其聚类为 10 个簇。对于每个簇,找到距离中心点最近的特征点,并将其作为代表点。最后在图像上显示均匀化后的特征点。
相关推荐
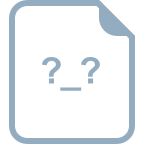
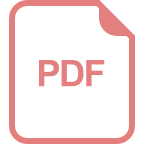












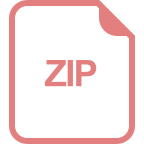
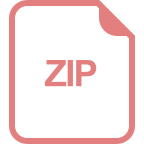
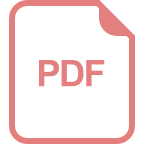