用用c #写以下代码,使用get方法表示以下代码this.year = year; this.manufacturer = manufacturer; this.model = model; this.price = price; this.isDrivable = isDrivable;
时间: 2024-03-26 11:35:31 浏览: 35
假设代码是在一个名为"Car"的类中,可以按照如下方式使用get方法来表示:
```csharp
public int Year
{
get { return year; }
}
public string Manufacturer
{
get { return manufacturer; }
}
public string Model
{
get { return model; }
}
public double Price
{
get { return price; }
}
public bool IsDrivable
{
get { return isDrivable; }
}
public Car(int year, string manufacturer, string model, double price, bool isDrivable)
{
this.year = year;
this.manufacturer = manufacturer;
this.model = model;
this.price = price;
this.isDrivable = isDrivable;
}
```
在上面的代码中,我们创建了5个属性:Year,Manufacturer,Model,Price和IsDrivable,每个属性都通过get方法来获取其值。最后,我们在构造函数中使用this关键字来给每个属性赋值。
相关问题
Use c # to complete the following code,Creating a Car class A class is a self-contained piece of software that is able to function on it own. We will model a car in software. Car Class Properties Year : int «private set» Manufacturer : string «private set» Model : string «private set» IsDrivable : bool «private set» Price : double «private set» Methods«constructor» Car(year : int, manufacturer : string, model : string, price : double, isDrivable: bool = true) PrintInfo() : void Description of field members: 1. year: this private member represents the year value of this object 2. manufacturer: this private member represents the manufacturer of this car 3. model: this private member represents the model of this car 4. isDrivable: this private member indicates if this car be driven 5. price: this private member represents the selling price of this car Constructor: 1. public Car(int year, string manufacturer, string model, double price, bool isDrivable = true): this is the constructor of this class. It takes five arguments and assigns them to the appropriate fields. You will assume that all the arguments will be sane (i.e. the values will not break your program). Apology: UML and some newer languages specify the type after the identifier. C# on the other hand requires that you specify the type and then the identifier
Sure, here is the completed code:
```
public class Car
{
public int Year { get; private set; }
public string Manufacturer { get; private set; }
public string Model { get; private set; }
public bool IsDrivable { get; private set; }
public double Price { get; private set; }
public Car(int year, string manufacturer, string model, double price, bool isDrivable = true)
{
Year = year;
Manufacturer = manufacturer;
Model = model;
Price = price;
IsDrivable = isDrivable;
}
public void PrintInfo()
{
Console.WriteLine($"Year: {Year}, Manufacturer: {Manufacturer}, Model: {Model}, IsDrivable: {IsDrivable}, Price: {Price}");
}
}
```
This code defines a Car class with the specified properties and methods. The constructor takes in the necessary arguments to initialize the object, and the PrintInfo method prints out the information about the car object.
用c#写一下代码,使用get和set方法表示以下代码,this.year = year; this.manufacturer = manufacturer; this.model = model; this.price = price; this.isDrivable = isDrivable;
假设代码是在一个名为"Car"的类中,可以按照如下方式使用get和set方法来表示:
```csharp
public int Year
{
get { return year; }
set { year = value; }
}
public string Manufacturer
{
get { return manufacturer; }
set { manufacturer = value; }
}
public string Model
{
get { return model; }
set { model = value; }
}
public double Price
{
get { return price; }
set { price = value; }
}
public bool IsDrivable
{
get { return isDrivable; }
set { isDrivable = value; }
}
public Car(int year, string manufacturer, string model, double price, bool isDrivable)
{
this.Year = year;
this.Manufacturer = manufacturer;
this.Model = model;
this.Price = price;
this.IsDrivable = isDrivable;
}
```
在上面的代码中,我们创建了5个属性:Year,Manufacturer,Model,Price和IsDrivable,每个属性都通过get和set方法来获取和设置其值。最后,我们在构造函数中使用this关键字来给每个属性赋值。当我们给这些属性赋值时,会自动调用set方法来设置属性的值。例如,当我们调用"this.Year = year;"时,会自动调用Year属性的set方法来设置属性的值。而当我们获取这些属性的值时,会自动调用get方法来获取属性的值。例如,当我们调用"int year = this.Year;"时,会自动调用Year属性的get方法来获取属性的值。
阅读全文
相关推荐



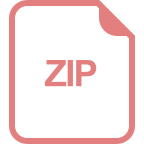
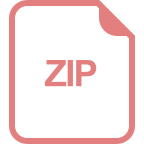
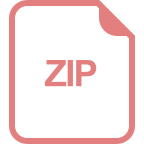
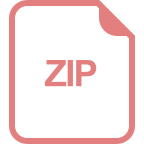
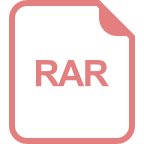
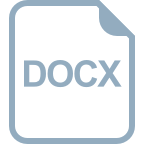
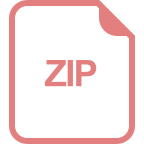
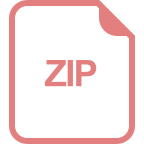
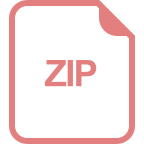
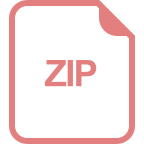