生成一个射击游戏的C语言代码
时间: 2024-06-09 15:06:48 浏览: 95
以下是一个简单的射击游戏的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAP_WIDTH 20
#define MAP_HEIGHT 10
#define PLAYER_SYMBOL 'P'
#define ENEMY_SYMBOL 'E'
#define BULLET_SYMBOL '*'
#define EMPTY_SYMBOL ' '
#define MAX_ENEMIES 5
#define MAX_BULLETS 10
typedef struct {
int x;
int y;
} Position;
typedef struct {
Position pos;
int health;
} Player;
typedef struct {
Position pos;
int health;
} Enemy;
typedef struct {
Position pos;
int active;
} Bullet;
void clear_screen() {
system("cls");
}
void print_map(Player player, Enemy enemies[], Bullet bullets[]) {
int i, j;
char map[MAP_HEIGHT][MAP_WIDTH];
// Initialize the map with empty symbols
for (i = 0; i < MAP_HEIGHT; i++) {
for (j = 0; j < MAP_WIDTH; j++) {
map[i][j] = EMPTY_SYMBOL;
}
}
// Place the player symbol on the map
map[player.pos.y][player.pos.x] = PLAYER_SYMBOL;
// Place the enemy symbols on the map
for (i = 0; i < MAX_ENEMIES; i++) {
if (enemies[i].health > 0) {
map[enemies[i].pos.y][enemies[i].pos.x] = ENEMY_SYMBOL;
}
}
// Place the bullet symbols on the map
for (i = 0; i < MAX_BULLETS; i++) {
if (bullets[i].active) {
map[bullets[i].pos.y][bullets[i].pos.x] = BULLET_SYMBOL;
}
}
// Print the map
for (i = 0; i < MAP_HEIGHT; i++) {
for (j = 0; j < MAP_WIDTH; j++) {
printf("%c", map[i][j]);
}
printf("\n");
}
}
void update_player(Player *player, char input) {
switch (input) {
case 'w':
player->pos.y--;
break;
case 'a':
player->pos.x--;
break;
case 's':
player->pos.y++;
break;
case 'd':
player->pos.x++;
break;
}
// Keep the player within the bounds of the map
if (player->pos.x < 0) player->pos.x = 0;
if (player->pos.x >= MAP_WIDTH) player->pos.x = MAP_WIDTH - 1;
if (player->pos.y < 0) player->pos.y = 0;
if (player->pos.y >= MAP_HEIGHT) player->pos.y = MAP_HEIGHT - 1;
}
void update_enemies(Enemy enemies[], Bullet bullets[]) {
int i, j;
for (i = 0; i < MAX_ENEMIES; i++) {
if (enemies[i].health > 0) {
// Move the enemy randomly
enemies[i].pos.x += rand() % 3 - 1;
enemies[i].pos.y += rand() % 3 - 1;
// Keep the enemy within the bounds of the map
if (enemies[i].pos.x < 0) enemies[i].pos.x = 0;
if (enemies[i].pos.x >= MAP_WIDTH) enemies[i].pos.x = MAP_WIDTH - 1;
if (enemies[i].pos.y < 0) enemies[i].pos.y = 0;
if (enemies[i].pos.y >= MAP_HEIGHT) enemies[i].pos.y = MAP_HEIGHT - 1;
// Check if the enemy collides with any bullets
for (j = 0; j < MAX_BULLETS; j++) {
if (bullets[j].active && bullets[j].pos.x == enemies[i].pos.x && bullets[j].pos.y == enemies[i].pos.y) {
bullets[j].active = 0;
enemies[i].health--;
}
}
}
}
}
void update_bullets(Bullet bullets[]) {
int i;
for (i = 0; i < MAX_BULLETS; i++) {
if (bullets[i].active) {
bullets[i].pos.y--;
if (bullets[i].pos.y < 0) {
bullets[i].active = 0;
}
}
}
}
void fire_bullet(Player player, Bullet bullets[]) {
int i;
for (i = 0; i < MAX_BULLETS; i++) {
if (!bullets[i].active) {
bullets[i].active = 1;
bullets[i].pos.x = player.pos.x;
bullets[i].pos.y = player.pos.y - 1;
break;
}
}
}
int main() {
// Initialize random seed
srand(time(NULL));
// Initialize player
Player player;
player.pos.x = MAP_WIDTH / 2;
player.pos.y = MAP_HEIGHT - 1;
player.health = 3;
// Initialize enemies
Enemy enemies[MAX_ENEMIES];
int i;
for (i = 0; i < MAX_ENEMIES; i++) {
enemies[i].pos.x = rand() % MAP_WIDTH;
enemies[i].pos.y = rand() % MAP_HEIGHT;
enemies[i].health = 1;
}
// Initialize bullets
Bullet bullets[MAX_BULLETS];
for (i = 0; i < MAX_BULLETS; i++) {
bullets[i].active = 0;
}
// Game loop
while (player.health > 0) {
// Clear the screen
clear_screen();
// Print the map
print_map(player, enemies, bullets);
// Update the player
char input;
printf("Enter movement (w,a,s,d) or fire (f): ");
scanf(" %c", &input);
if (input == 'f') {
fire_bullet(player, bullets);
} else {
update_player(&player, input);
}
// Update the enemies
update_enemies(enemies, bullets);
// Update the bullets
update_bullets(bullets);
// Wait for a short time to slow down the game
usleep(100000);
}
// Game over
printf("Game over!\n");
return 0;
}
```
该代码使用了以下的数据结构和常量:
- `Position`:包含 x 和 y 坐标的结构体。
- `Player`:包含玩家位置和生命值的结构体。
- `Enemy`:包含敌人位置和生命值的结构体。
- `Bullet`:包含子弹位置和是否激活的结构体。
- `MAP_WIDTH`:地图的宽度。
- `MAP_HEIGHT`:地图的高度。
- `PLAYER_SYMBOL`:玩家的符号。
- `ENEMY_SYMBOL`:敌人的符号。
- `BULLET_SYMBOL`:子弹的符号。
- `EMPTY_SYMBOL`:空格的符号。
- `MAX_ENEMIES`:敌人的最大数量。
- `MAX_BULLETS`:子弹的最大数量。
该代码实现了以下功能:
- 生成随机的敌人和玩家位置。
- 玩家可以使用 w、a、s、d 键移动,并且可以使用 f 键发射子弹。
- 敌人会在地图上随机移动并且会被子弹击中减少生命值。
- 子弹会在地图上向上移动并且会在到达顶部或者击中敌人时消失。
- 游戏会在玩家生命值为 0 时结束并显示 Game over!。
相关推荐
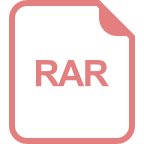
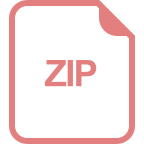
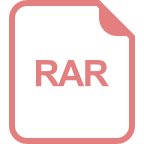














