c语言easyx有图像的双人射击小游戏代码
时间: 2023-07-31 09:14:10 浏览: 215
以下是一个简单的双人射击小游戏的代码,使用C语言和EasyX图形库编写。
游戏规则:
- 每个玩家控制一个坦克,可以移动和射击。
- 玩家每次射击会发射一枚子弹,子弹可以击中对方坦克或者砖头。
- 每个玩家初始有3条生命,被对方击中后生命减1,生命为0时游戏结束。
- 当其中一个玩家的生命为0时,游戏结束。
代码如下:
```c
#include <graphics.h>
#include <conio.h>
#include <time.h>
// 定义窗口大小和坦克、子弹的大小
const int WIDTH = 800;
const int HEIGHT = 600;
const int TANK_SIZE = 30;
const int BULLET_SIZE = 5;
// 定义玩家的生命
int life1 = 3;
int life2 = 3;
// 定义坦克的位置和速度
int x1 = 100, y1 = 100, speed1 = 5;
int x2 = 500, y2 = 400, speed2 = 5;
// 定义子弹的位置和速度
int bx1 = 0, by1 = 0, bspeed1 = 10;
int bx2 = 0, by2 = 0, bspeed2 = 10;
// 定义砖头的位置和大小
int brick_x = 300, brick_y = 200, brick_size = 50;
// 判断两个矩形是否相交
bool isOverlap(int x1, int y1, int w1, int h1, int x2, int y2, int w2, int h2) {
return x1 + w1 > x2 && x2 + w2 > x1 && y1 + h1 > y2 && y2 + h2 > y1;
}
int main() {
// 初始化窗口和随机数生成器
initgraph(WIDTH, HEIGHT);
srand(time(NULL));
// 游戏循环
while (true) {
// 清空屏幕
cleardevice();
// 绘制砖头
setfillcolor(YELLOW);
solidrectangle(brick_x, brick_y, brick_x + brick_size, brick_y + brick_size);
// 绘制玩家1的坦克
setfillcolor(RED);
solidrectangle(x1, y1, x1 + TANK_SIZE, y1 + TANK_SIZE);
// 绘制玩家2的坦克
setfillcolor(GREEN);
solidrectangle(x2, y2, x2 + TANK_SIZE, y2 + TANK_SIZE);
// 移动玩家1的坦克
if (GetAsyncKeyState('A') & 0x8000) x1 -= speed1;
if (GetAsyncKeyState('D') & 0x8000) x1 += speed1;
if (GetAsyncKeyState('W') & 0x8000) y1 -= speed1;
if (GetAsyncKeyState('S') & 0x8000) y1 += speed1;
// 移动玩家2的坦克
if (GetAsyncKeyState(VK_LEFT) & 0x8000) x2 -= speed2;
if (GetAsyncKeyState(VK_RIGHT) & 0x8000) x2 += speed2;
if (GetAsyncKeyState(VK_UP) & 0x8000) y2 -= speed2;
if (GetAsyncKeyState(VK_DOWN) & 0x8000) y2 += speed2;
// 玩家1发射子弹
if (GetAsyncKeyState('J') & 0x8000) {
if (bx1 == 0 && by1 == 0) {
bx1 = x1 + TANK_SIZE / 2 - BULLET_SIZE / 2;
by1 = y1 - BULLET_SIZE;
}
}
// 玩家2发射子弹
if (GetAsyncKeyState(VK_SPACE) & 0x8000) {
if (bx2 == 0 && by2 == 0) {
bx2 = x2 + TANK_SIZE / 2 - BULLET_SIZE / 2;
by2 = y2 + TANK_SIZE;
}
}
// 移动玩家1的子弹
if (by1 > 0) {
by1 -= bspeed1;
setfillcolor(RED);
solidcircle(bx1 + BULLET_SIZE / 2, by1 + BULLET_SIZE / 2, BULLET_SIZE / 2);
} else {
bx1 = 0;
by1 = 0;
}
// 移动玩家2的子弹
if (by2 < HEIGHT) {
by2 += bspeed2;
setfillcolor(GREEN);
solidcircle(bx2 + BULLET_SIZE / 2, by2 + BULLET_SIZE / 2, BULLET_SIZE / 2);
} else {
bx2 = 0;
by2 = 0;
}
// 判断玩家1的子弹是否击中砖头或者玩家2的坦克
if (bx1 > 0 && by1 > 0) {
if (isOverlap(bx1, by1, BULLET_SIZE, BULLET_SIZE, brick_x, brick_y, brick_size, brick_size)) {
bx1 = 0;
by1 = 0;
} else if (isOverlap(bx1, by1, BULLET_SIZE, BULLET_SIZE, x2, y2, TANK_SIZE, TANK_SIZE)) {
bx1 = 0;
by1 = 0;
life2--;
if (life2 == 0) {
outtextxy(WIDTH / 2 - 50, HEIGHT / 2 - 50, "Player 1 win!");
_getch();
break;
}
}
}
// 判断玩家2的子弹是否击中砖头或者玩家1的坦克
if (bx2 > 0 && by2 > 0) {
if (isOverlap(bx2, by2, BULLET_SIZE, BULLET_SIZE, brick_x, brick_y, brick_size, brick_size)) {
bx2 = 0;
by2 = 0;
} else if (isOverlap(bx2, by2, BULLET_SIZE, BULLET_SIZE, x1, y1, TANK_SIZE, TANK_SIZE)) {
bx2 = 0;
by2 = 0;
life1--;
if (life1 == 0) {
outtextxy(WIDTH / 2 - 50, HEIGHT / 2 - 50, "Player 2 win!");
_getch();
break;
}
}
}
// 显示玩家的生命
settextcolor(RED);
char str1[20];
sprintf_s(str1, "Player 1 life: %d", life1);
outtextxy(10, 10, str1);
settextcolor(GREEN);
char str2[20];
sprintf_s(str2, "Player 2 life: %d", life2);
outtextxy(WIDTH - 120, 10, str2);
// 延时
Sleep(20);
}
// 关闭窗口
closegraph();
return 0;
}
```
以上就是一个简单的双人射击小游戏的代码,希望能够对你有所帮助。
阅读全文
相关推荐
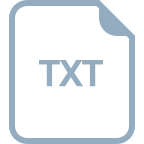
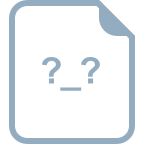
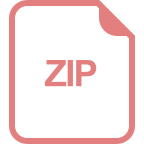
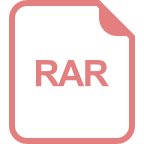
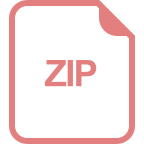











