用C语言easyx写贪吃蛇代码
时间: 2023-06-14 16:03:50 浏览: 200
以下是使用EasyX图形库和C语言编写的贪吃蛇游戏代码:
```c
#include <graphics.h>
#include <conio.h>
#include <time.h>
#include <stdlib.h>
#define HEIGHT 480 // 窗口高度
#define WIDTH 640 // 窗口宽度
#define SIZE 20 // 蛇身大小
#define MAXLEN 100 // 蛇的最大长度
int snakeX[MAXLEN]; // 蛇身X坐标
int snakeY[MAXLEN]; // 蛇身Y坐标
int len = 5; // 蛇的初始长度
int dir = 0; // 蛇头方向
int foodX, foodY; // 食物坐标
// 初始化蛇的位置和食物的位置
void init() {
for (int i = 0; i < len; i++) {
snakeX[i] = WIDTH / 2 - i * SIZE;
snakeY[i] = HEIGHT / 2;
}
foodX = rand() % (WIDTH / SIZE - 2) * SIZE + SIZE;
foodY = rand() % (HEIGHT / SIZE - 2) * SIZE + SIZE;
}
// 判断蛇是否撞墙或者撞自己
bool isGameOver() {
if (snakeX[0] >= WIDTH || snakeX[0] < 0 || snakeY[0] >= HEIGHT || snakeY[0] < 0) {
return true;
}
for (int i = 1; i < len; i++) {
if (snakeX[0] == snakeX[i] && snakeY[0] == snakeY[i]) {
return true;
}
}
return false;
}
// 画蛇
void drawSnake() {
setfillcolor(YELLOW);
for (int i = 0; i < len; i++) {
fillrectangle(snakeX[i], snakeY[i], snakeX[i] + SIZE, snakeY[i] + SIZE);
}
}
// 更新蛇
void updateSnake() {
for (int i = len - 1; i > 0; i--) {
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
switch (dir) {
case 0: snakeX[0] += SIZE; break; // 向右
case 1: snakeY[0] -= SIZE; break; // 向上
case 2: snakeX[0] -= SIZE; break; // 向左
case 3: snakeY[0] += SIZE; break; // 向下
}
}
// 随机生成食物
void newFood() {
foodX = rand() % (WIDTH / SIZE - 2) * SIZE + SIZE;
foodY = rand() % (HEIGHT / SIZE - 2) * SIZE + SIZE;
}
// 画食物
void drawFood() {
setfillcolor(GREEN);
fillrectangle(foodX, foodY, foodX + SIZE, foodY + SIZE);
}
int main() {
srand(time(NULL)); // 随机数种子
initgraph(WIDTH, HEIGHT);
init();
while (true) {
drawSnake();
drawFood();
updateSnake();
if (snakeX[0] == foodX && snakeY[0] == foodY) { // 吃到食物
len++;
newFood();
}
if (isGameOver()) { // 游戏结束
break;
}
Sleep(100); // 控制速度
cleardevice(); // 清屏
if (_kbhit()) { // 按键检测
switch (_getch()) {
case 'w': dir = 1; break; // 向上
case 'a': dir = 2; break; // 向左
case 's': dir = 3; break; // 向下
case 'd': dir = 0; break; // 向右
}
}
}
closegraph();
return 0;
}
```
运行代码后,即可看到贪吃蛇游戏窗口,并可以使用键盘的 WASD 键控制蛇的移动。
阅读全文
相关推荐
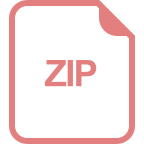
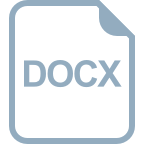















