c语言贪吃蛇easyx排行榜
时间: 2023-09-21 22:11:07 浏览: 227
实现C语言贪吃蛇的排行榜需要以下步骤:
1. 创建一个结构体来存储玩家的信息,包括玩家的名称和分数。
2. 使用文件来保存玩家的信息,每个玩家的信息占用一行,包括玩家的名称和分数。
3. 实现读取文件并将信息存储到结构体数组中的函数。
4. 实现将新玩家的信息加入到结构体数组中的函数,并将结构体数组按照分数从高到低排序。
5. 实现将结构体数组中的信息写入文件的函数。
6. 在游戏结束后调用上述函数将玩家的信息保存到文件中,并输出排行榜。
以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_PLAYERS 10
#define MAX_NAME_LEN 20
struct player {
char name[MAX_NAME_LEN+1];
int score;
};
void read_players(struct player players[]) {
FILE *file = fopen("players.txt", "r");
if (file == NULL) {
perror("Failed to open file");
exit(EXIT_FAILURE);
}
int i = 0;
char name[MAX_NAME_LEN+1];
int score;
while (fscanf(file, "%s %d", name, &score) == 2 && i < MAX_PLAYERS) {
strncpy(players[i].name, name, MAX_NAME_LEN+1);
players[i].score = score;
i++;
}
fclose(file);
}
void add_player(struct player players[], const char *name, int score) {
int i = MAX_PLAYERS - 1;
while (i > 0 && score > players[i-1].score) {
players[i] = players[i-1];
i--;
}
strncpy(players[i].name, name, MAX_NAME_LEN+1);
players[i].score = score;
}
void write_players(struct player players[]) {
FILE *file = fopen("players.txt", "w");
if (file == NULL) {
perror("Failed to open file");
exit(EXIT_FAILURE);
}
for (int i = 0; i < MAX_PLAYERS; i++) {
fprintf(file, "%s %d\n", players[i].name, players[i].score);
}
fclose(file);
}
void print_players(struct player players[]) {
printf("Rank\tName\tScore\n");
for (int i = 0; i < MAX_PLAYERS; i++) {
printf("%d\t%s\t%d\n", i+1, players[i].name, players[i].score);
}
}
int main() {
struct player players[MAX_PLAYERS];
read_players(players);
char name[MAX_NAME_LEN+1];
int score;
printf("Enter your name: ");
scanf("%s", name);
printf("Enter your score: ");
scanf("%d", &score);
add_player(players, name, score);
write_players(players);
print_players(players);
return 0;
}
```
该程序可以读取名为“players.txt”的文件中的玩家信息,并将新的玩家信息添加到该文件中,最后输出排行榜。由于该程序只是一个示例,排行榜只会显示最高分的10个玩家。如果要显示更多的玩家,可以将MAX_PLAYERS的值增加到所需的数量。
阅读全文
相关推荐
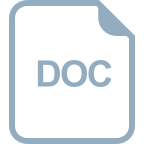
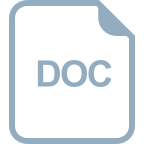
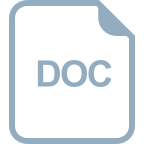


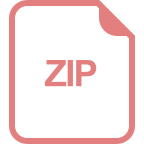
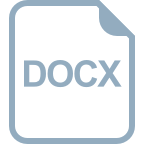

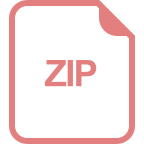
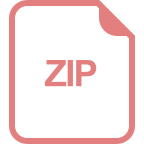
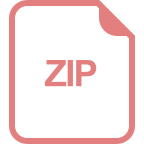
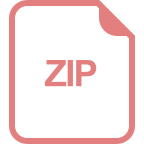
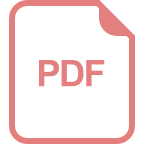
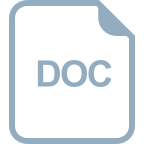


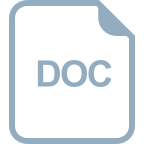