mean-shift的python实现
时间: 2023-09-24 19:09:19 浏览: 124
以下是使用Python实现mean-shift算法的示例代码:
```python
from sklearn.cluster import MeanShift, estimate_bandwidth
import numpy as np
# 生成数据
X = np.random.randn(1000, 2)
# 估计带宽
bandwidth = estimate_bandwidth(X, quantile=0.2, n_samples=500)
# 使用MeanShift聚类
ms = MeanShift(bandwidth=bandwidth, bin_seeding=True)
ms.fit(X)
# 输出聚类结果
labels = ms.labels_
cluster_centers = ms.cluster_centers_
n_clusters_ = len(np.unique(labels))
print("Number of estimated clusters : %d" % n_clusters_)
```
在上面的代码中,我们首先使用Numpy生成了1000个二维随机数据点。然后,使用`estimate_bandwidth`函数估计了带宽参数,并将其作为输入传递给`MeanShift`类的构造函数。接下来,我们使用`fit`方法拟合数据,并使用`labels_`属性获得数据点的簇标签,使用`cluster_centers_`属性获得聚类中心,最后输出聚类结果。
相关问题
mean-shift图像分割python
以下是使用Mean Shift算法进行图像分割的Python代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.cluster import MeanShift
# 读取图像数据
image = plt.imread('image.jpg')
# 将图像数据转换为二维数组
samples = image.reshape(-1, 3)
# 使用Mean Shift算法进行聚类
bandwidth = 0.1
ms = MeanShift(bandwidth=bandwidth, bin_seeding=True).fit(samples)
# 获取聚类中心
cluster_centers = ms.cluster_centers_
# 根据聚类结果对图像进行分割
segmented_image = cluster_centers[ms.labels_].reshape(image.shape)
# 显示原始图像和分割后的图像
plt.subplot(1, 2, 1)
plt.imshow(image)
plt.title('Original Image')
plt.axis('off')
plt.subplot(1, 2, 2)
plt.imshow(segmented_image)
plt.title('Segmented Image')
plt.axis('off')
plt.show()
```
这段代码首先读取图像数据,并将其转换为二维数组。然后使用Mean Shift算法对图像进行聚类,得到聚类中心。最后根据聚类结果对图像进行分割,并显示原始图像和分割后的图像。
实现基于mean-shift的图像分割的Python代码
很高兴为您解答,以下是基于mean-shift的图像分割的Python代码:
```
import numpy as np
import cv2
def image_segmentation_mean_shift(image, spatial_radius, color_radius, min_density):
# define mean shift algorithm parameters
ms = cv2.pyrMeanShiftFiltering(image, sp=spatial_radius, sr=color_radius,
minRepeatability=min_density)
return ms
# test the code
image = cv2.imread('test.jpg')
segmented_image = image_segmentation_mean_shift(image, spatial_radius=50, color_radius=100, min_density=10)
# display the results
cv2.imshow("Original Image", image)
cv2.imshow("Segmented Image", segmented_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
希望对您有所帮助!当然,如果您有任何关于该代码的问题或需要进一步的解释,请随时告诉我。
阅读全文
相关推荐
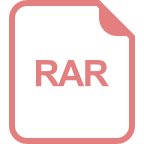
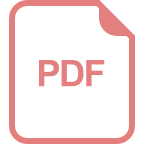
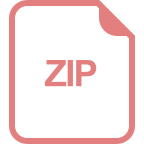
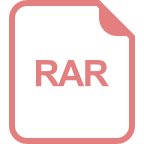
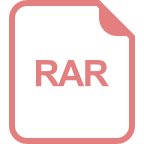
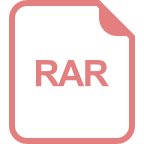
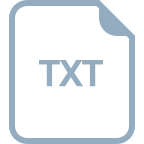
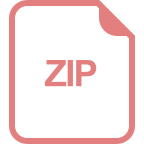
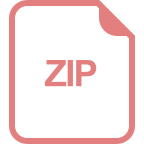
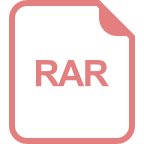
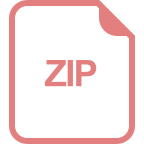
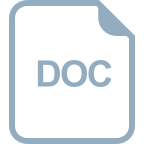
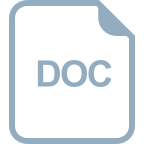
