写python代码,调用opencv库与sklearn库,读取文件夹中所有图像,对这些图像进行SIFT特征提取,再对提取到的特征进行mean shift聚类,并得出轮廓系数,将聚类后的图像保存到新的文件夹中
时间: 2024-05-05 21:19:20 浏览: 11
好的,这是一个需要用到多个技能的问题。我需要先回答一些基础知识,才能回答你的问题。
首先,OpenCV是一个广泛使用的开源计算机视觉库,可以用于图像和视频处理。它支持多种编程语言,包括Python。sklearn是一个python编写的AI框架,它包含了大量常用的机器学习算法和工具,能够方便地进行各种机器学习任务。
其次,SIFT是一种常用的特征描述算法,可用于计算图像中特征点的位置、方向和尺度等信息。而mean shift是一种非参数聚类算法,它适用于任何形状的分布,能够自动调整聚类中心的位置。
对于你的问题,我们可以考虑如下操作:
1.导入需要的库和模块,如OpenCV和sklearn等。
2.获取文件夹中的所有图像。
3.循环遍历每个图像,对其进行SIFT特征提取,得到一组特征向量。
4.利用sklearn库中的mean shift算法对这些特征向量进行聚类,得到多个聚类中心。
5.计算每个聚类的轮廓系数,并保存聚类后的图像到新的文件夹中。
具体的实现步骤和代码可以参考下面的代码示例:
```
import cv2
import numpy as np
from sklearn.cluster import MeanShift, estimate_bandwidth
from sklearn import metrics
import os
#1.导入需要的库和模块
#2.获取文件夹中的所有图像
image_folder = 'your/image/folder/path'
output_folder = 'your/output/folder/path'
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for filename in os.listdir(image_folder):
img_path = os.path.join(image_folder, filename)
#3.对每个图像进行SIFT特征提取
img = cv2.imread(img_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
sift = cv2.xfeatures2d.SIFT_create()
keypoints, features = sift.detectAndCompute(gray, None)
#4.聚类特征向量
bandwidth = estimate_bandwidth(features, quantile=0.2, n_samples=500)
if bandwidth == 0:
continue
ms = MeanShift(bandwidth=bandwidth, bin_seeding=True)
ms.fit(features)
cluster_centers = ms.cluster_centers_
#5.计算每个聚类的轮廓系数,并保存聚类后的图像到新的文件夹中
labels = ms.labels_
n_clusters_ = len(cluster_centers)
silhouette_score = metrics.silhouette_score(features, labels, metric='euclidean')
for i in range(n_clusters_):
cluster_center = cluster_centers[i]
cluster_keypoints = [keypoints[j] for j in range(len(keypoints)) if labels[j] == i]
img_copy = img.copy()
for kp in cluster_keypoints:
cv2.circle(img_copy, (int(kp.pt[0]), int(kp.pt[1])), 2, (0, 0, 255), -1)
output_path = os.path.join(output_folder, filename.split('.')[0] + '_cluster_' + str(i) + '.jpg')
cv2.imwrite(output_path, img_copy)
```
希望能够对你有所帮助,有什么问题可以随时问我。
相关推荐
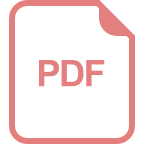
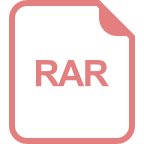
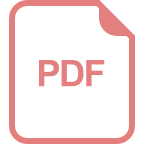














