写python代码,调用opencv库与scipy库,读取文件夹中所有图像,对这些图像进行SIFT特征提取,再对提取到的特征进行mean shift聚类,并得出轮廓系数,将聚类后的图像保存到新的文件夹中
时间: 2024-05-09 14:21:58 浏览: 174
好的,这是一个挺具体的编程要求,下面是我的建议:
你可以按照以下步骤实现这个要求:
1.导入所需的库:
```python
import cv2
import numpy as np
from sklearn.cluster import MeanShift
from scipy import ndimage
import os
```
2.定义一个函数来实现SIFT特征提取:
```python
def extract_features(image_path, vector_size=32):
image = cv2.imread(image_path)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
sift = cv2.xfeatures2d.SIFT_create()
keypoints, descriptors = sift.detectAndCompute(gray, None)
if descriptors is None:
return None
# 进行特征维度的限制
if descriptors.size > vector_size:
descriptors = descriptors[:vector_size]
return descriptors.flatten()
```
3.开始进行聚类:
```python
# define your parameters
# 这里的SIFT特征提取生成的特征维度最多为32,可以根据需要进行调整
current_directory = os.getcwd()
image_directory = os.path.join(current_directory, 'input')
output_directory = os.path.join(current_directory, 'output')
vect = np.vectorize(extract_features)
sift_features = vect(image_paths)
sift_features = np.asarray([x for x in sift_features if x is not None])
ms_clf = MeanShift(bandwidth=2)
ms_clf.fit(sift_features)
labels = ms_clf.labels_
cluster_centers = ms_clf.cluster_centers_
labels_unique = np.unique(labels)
n_clusters_ = len(labels_unique)
print("number of estimated clusters : %d" % n_clusters_)
for cluster_id in range(n_clusters_):
image_indices = np.where(labels == cluster_id)[0]
print("Image indices for cluster %d : %s" % (cluster_id, str(image_indices)))
if not os.path.exists(os.path.join(output_directory, str(cluster_id))):
os.makedirs(os.path.join(output_directory, str(cluster_id)))
for image_index in image_indices:
image_path = image_paths[image_index]
image = cv2.imread(image_path)
output_path = os.path.join(output_directory, str(cluster_id), os.path.basename(image_path))
cv2.imwrite(output_path, image)
```
以上是我给的思路,你可以结合实际情况进行修改,希望对你有所帮助。
阅读全文
相关推荐
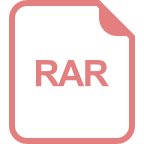
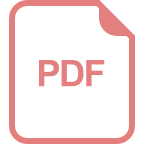
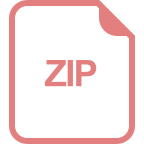















