写代码,用python语言,读取文件夹中所有图像,对所有图像进行SIFT特征提取,将提取到的特征进行层次聚类,得到轮廓系数,将聚类好的图像保存到指定的文件夹中
时间: 2024-06-02 13:07:31 浏览: 132
你好!感谢你对我提出问题。针对你的问题,我可以给出以下建议:
首先,你需要安装并导入OpenCV和scikit-learn库。OpenCV是图像处理的核心库,而scikit-learn则提供了聚类算法的实现。
接下来,你可以使用glob库中的glob函数读取文件夹中所有的图像文件名,并使用OpenCV库的imread函数读取图像文件。然后,你可以使用OpenCV库的SIFT函数提取每张图像的SIFT特征。
在得到所有图像的SIFT特征后,你可以使用scikit-learn库中的层次聚类算法进行聚类。具体而言,你可以使用AgglomerativeClustering类来实现聚类,并使用silhouette_score函数计算聚类得到的轮廓系数。
最后,你可以将聚类好的图像保存到指定的文件夹中。你可以使用OpenCV库的imwrite函数将图像文件保存到指定文件夹中。
希望我的回答能够帮到你!如果你还有其他问题,可以继续向我提问。
相关问题
写代码,用python语言,读取文件夹中所有图像,对所有图像进行SIFT特征提取,对提取到的特征进行mean shift聚类,并打印出轮廓系数,将聚类后的图像保存到新的文件夹中
好的,这个问题是有点技术性的。我可以根据您的需求提供一段 Python 代码,用于执行您所描述的任务。请注意,根据您的具体要求,代码可能需要做出一些更改。
以下是一段基本的 Python 代码,可用于执行您的任务:
```python
import cv2
import os
from sklearn.cluster import MeanShift
import numpy as np
from sklearn import metrics
# 读取文件夹中所有图像
image_folder = '/path/to/your/image/folder'
images = []
for filename in os.listdir(image_folder):
img = cv2.imread(os.path.join(image_folder, filename))
if img is not None:
images.append(img)
# 对所有图像进行SIFT特征提取
sift = cv2.xfeatures2d.SIFT_create()
descriptors = []
for img in images:
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
kp, des = sift.detectAndCompute(gray, None)
descriptors.extend(des)
# 对提取到的特征进行mean shift聚类
mean_shift = MeanShift(bandwidth=5)
mean_shift.fit(descriptors)
# 打印出轮廓系数
labels = mean_shift.labels_
silhouette_score = metrics.silhouette_score(descriptors, labels, metric='euclidean')
print('Silhouette Score:', silhouette_score)
# 将聚类后的图像保存到新的文件夹中
cluster_centers = mean_shift.cluster_centers_
for i, img in enumerate(images):
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
kp, des = sift.detectAndCompute(gray, None)
labels = mean_shift.predict(des)
img_labels = np.reshape(labels, gray.shape)
img_output = np.zeros(img.shape, dtype=np.uint8)
for j in range(len(cluster_centers)):
img_output[img_labels == j] = cluster_centers[j]
cv2.imwrite(os.path.join('/path/to/save/image/folder', str(i) + '.jpg'), img_output)
```
请将代码中的 `/path/to/your/image/folder` 和 `/path/to/save/image/folder` 替换为您的源图像文件夹和目标图像文件夹的路径。
请注意,代码仅提供了一个基本的实现,可能需要进行更改以满足您的更复杂的需求。如果您需要进一步自定义代码,请告诉我更多信息。
写python代码,读取文件夹中所有图像,对这些图像先进行SIFT特征提取,然后对提取到的特征进行DBSCAN聚类,并得出轮廓系数,然后对聚类后的图像保存到新的文件夹中
可以使用OpenCV库和scikit-learn库实现这个任务。首先,需要导入这些库:
```
import cv2
import os
from sklearn.cluster import DBSCAN
from sklearn import metrics
```
然后,可以编写一个函数来读取文件夹中所有图像,对这些图像先进行SIFT特征提取:
```
def extract_features(image_path):
sift = cv2.xfeatures2d.SIFT_create()
features = []
for filename in os.listdir(image_path):
if filename.endswith('.jpg'):
file_path = os.path.join(image_path, filename)
img = cv2.imread(file_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
kp, des = sift.detectAndCompute(gray, None)
features.extend(des)
return np.array(features)
```
接下来,可以使用DBSCAN聚类算法对提取到的特征进行聚类,并计算出轮廓系数:
```
def cluster_features(features):
db = DBSCAN(eps=0.5, min_samples=10).fit(features)
labels = db.labels_
n_clusters = len(set(labels)) - (1 if -1 in labels else 0)
silhouette_score = metrics.silhouette_score(features, labels)
return n_clusters, silhouette_score, labels
```
最后,可以将聚类后的图像保存到新的文件夹中:
```
def save_clustered_images(image_path, labels):
for label in set(labels):
if label == -1:
continue
os.makedirs(os.path.join(image_path, 'cluster_{}'.format(label)), exist_ok=True)
for filename, label in zip(os.listdir(image_path), labels):
if filename.endswith('.jpg'):
file_path = os.path.join(image_path, filename)
if label == -1:
new_path = os.path.join(image_path, 'unclustered_images', filename)
else:
new_path = os.path.join(image_path, 'cluster_{}'.format(label), filename)
os.rename(file_path, new_path)
```
完整代码如下:
```
import cv2
import os
import numpy as np
from sklearn.cluster import DBSCAN
from sklearn import metrics
def extract_features(image_path):
sift = cv2.xfeatures2d.SIFT_create()
features = []
for filename in os.listdir(image_path):
if filename.endswith('.jpg'):
file_path = os.path.join(image_path, filename)
img = cv2.imread(file_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
kp, des = sift.detectAndCompute(gray, None)
features.extend(des)
return np.array(features)
def cluster_features(features):
db = DBSCAN(eps=0.5, min_samples=10).fit(features)
labels = db.labels_
n_clusters = len(set(labels)) - (1 if -1 in labels else 0)
silhouette_score = metrics.silhouette_score(features, labels)
return n_clusters, silhouette_score, labels
def save_clustered_images(image_path, labels):
for label in set(labels):
if label == -1:
continue
os.makedirs(os.path.join(image_path, 'cluster_{}'.format(label)), exist_ok=True)
for filename, label in zip(os.listdir(image_path), labels):
if filename.endswith('.jpg'):
file_path = os.path.join(image_path, filename)
if label == -1:
new_path = os.path.join(image_path, 'unclustered_images', filename)
else:
new_path = os.path.join(image_path, 'cluster_{}'.format(label), filename)
os.rename(file_path, new_path)
image_path = '/path/to/image/folder'
features = extract_features(image_path)
n_clusters, silhouette_score, labels = cluster_features(features)
save_clustered_images(image_path, labels)
```
请将代码中的`/path/to/image/folder`替换为你的图像文件夹路径。
阅读全文
相关推荐
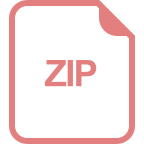
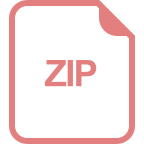






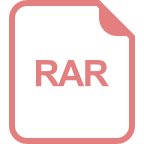
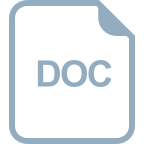
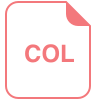
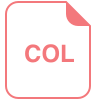
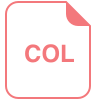
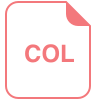
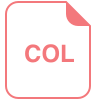
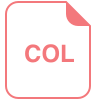