MySQL数据库存储dlib人脸特征信息并识别人脸进行匹配代码
时间: 2024-05-09 18:15:48 浏览: 5
以下是MySQL数据库存储dlib人脸特征信息并识别人脸进行匹配的代码示例:
首先,需要使用dlib库提取人脸特征并将其存储到MySQL数据库中。这里我们使用Python语言编写代码。
```python
import dlib
import numpy as np
import mysql.connector
# 连接MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="test"
)
# 创建数据表
mycursor = mydb.cursor()
mycursor.execute("CREATE TABLE IF NOT EXISTS faces (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), features TEXT)")
# 加载dlib的人脸检测器和面部标志器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 加载已知人脸图像并提取特征并存储到数据库中
def store_face_features(image_path, name):
img = dlib.load_rgb_image(image_path)
dets = detector(img, 1)
if len(dets) != 1:
print("图像中存在多个或无人脸")
return
shape = predictor(img, dets[0])
face_descriptor = np.array(face_recognition.face_encodings(img, [shape])[0], dtype=np.float32)
face_descriptor_str = ','.join(map(str, face_descriptor))
sql = "INSERT INTO faces (name, features) VALUES (%s, %s)"
val = (name, face_descriptor_str)
mycursor.execute(sql, val)
mydb.commit()
# 存储人脸特征数据到MySQL数据库中
store_face_features("known_face.jpg", "Tom")
store_face_features("known_face2.jpg", "Jerry")
```
接下来,我们可以使用dlib库和MySQL数据库中存储的人脸特征数据进行人脸匹配。这里我们同样使用Python语言编写代码。
```python
import dlib
import numpy as np
import mysql.connector
# 连接MySQL数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="test"
)
# 加载dlib的人脸检测器和面部标志器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor("shape_predictor_68_face_landmarks.dat")
# 从MySQL数据库中加载已知人脸特征数据
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM faces")
results = mycursor.fetchall()
known_face_names = []
known_face_features = []
for result in results:
known_face_names.append(result[1])
known_face_features.append(list(map(float, result[2].split(','))))
# 加载待匹配的人脸图像并提取特征
img = dlib.load_rgb_image("unknown_face.jpg")
dets = detector(img, 1)
if len(dets) != 1:
print("图像中存在多个或无人脸")
exit()
shape = predictor(img, dets[0])
face_descriptor = np.array(face_recognition.face_encodings(img, [shape])[0], dtype=np.float32)
# 计算欧氏距离
def euclidean_distance(x, y):
return np.sqrt(np.sum((np.array(x) - np.array(y)) ** 2))
# 匹配待匹配人脸特征与已知人脸特征
distances = [euclidean_distance(face_descriptor, known_face_feature) for known_face_feature in known_face_features]
min_distance_index = np.argmin(distances)
# 打印匹配结果
if distances[min_distance_index] < 0.6:
print("匹配成功,匹配到的人脸是:" + known_face_names[min_distance_index])
else:
print("未能匹配到已知人脸")
```
以上代码示例中,我们使用了dlib库提取人脸特征,并将其存储到MySQL数据库中。然后,我们使用MySQL数据库中存储的人脸特征数据进行人脸匹配。最后,打印匹配结果。
相关推荐
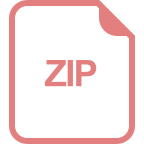
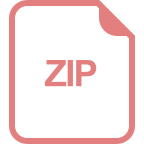
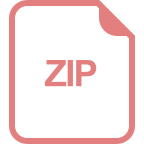












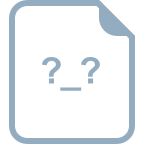
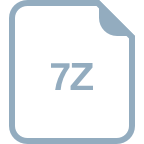
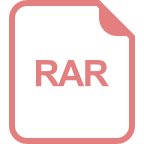