包含指针和结构体的代码以及相关注释
时间: 2023-07-13 17:10:09 浏览: 53
以下是一个简单的示例代码,包含指针和结构体的使用:
```c
#include <stdio.h>
// 定义结构体
struct student {
char name[20];
int age;
float score;
};
// 函数声明
void print_student(struct student *s);
int main() {
// 创建结构体变量并初始化
struct student s1 = {"Tom", 18, 89.5};
// 创建指向结构体的指针变量并赋值为结构体变量的地址
struct student *p = &s1;
// 通过指针访问结构体成员
printf("Name: %s\n", p->name);
printf("Age: %d\n", p->age);
printf("Score: %.2f\n", p->score);
// 通过指针修改结构体成员
p->age = 19;
p->score = 92.0;
// 调用函数打印结构体
print_student(p);
return 0;
}
// 函数定义
void print_student(struct student *s) {
printf("Name: %s\n", s->name);
printf("Age: %d\n", s->age);
printf("Score: %.2f\n", s->score);
}
```
注释如下:
- 第 4 行:定义了一个名为 `student` 的结构体,包含了学生的姓名、年龄和分数。
- 第 7 行:函数声明,用于打印结构体变量的内容。
- 第 9 行:`main` 函数的开始。
- 第 11 行:创建结构体变量 `s1` 并初始化。
- 第 13 行:创建指向结构体的指针变量 `p`,并将其赋值为结构体变量 `s1` 的地址。
- 第 16 行:通过指针访问结构体成员的值,并打印输出。
- 第 20 行:通过指针修改结构体成员的值。
- 第 23 行:调用函数 `print_student`,并将结构体指针 `p` 作为参数传递。
- 第 28 行:函数 `print_student` 的定义,接收一个结构体指针作为参数,打印结构体成员的值。
阅读全文
相关推荐








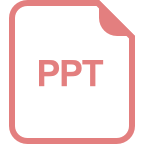
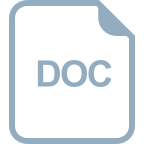
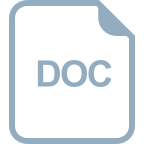
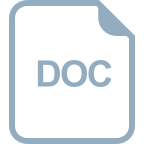
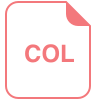

