用java写一个俄罗斯方块游戏
时间: 2023-12-18 11:03:32 浏览: 76
好的,下面是一个基于Java Swing的俄罗斯方块游戏的代码示例:
```
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.Timer;
public class Tetris extends JPanel implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int BLOCK_SIZE = 30;
private static final int BOARD_WIDTH = 10;
private static final int BOARD_HEIGHT = 20;
private static final int GAME_SPEED = 500;
private Timer timer;
private Block currentBlock;
private ArrayList<Block> blocks;
private int[][] board;
private Random random;
public Tetris() {
setPreferredSize(new Dimension(BLOCK_SIZE * BOARD_WIDTH, BLOCK_SIZE * BOARD_HEIGHT));
setBackground(Color.WHITE);
timer = new Timer(GAME_SPEED, this);
random = new Random();
board = new int[BOARD_HEIGHT][BOARD_WIDTH];
blocks = new ArrayList<Block>();
addNewBlock();
timer.start();
}
private void addNewBlock() {
int type = random.nextInt(7);
int x = BOARD_WIDTH / 2 - 1;
int y = 1;
currentBlock = new Block(type, x, y);
if (isColliding(currentBlock)) {
timer.stop();
JFrame frame = (JFrame) getTopLevelAncestor();
frame.setTitle("Game Over!");
return;
}
blocks.add(currentBlock);
}
private boolean isColliding(Block block) {
for (Point p : block.getPoints()) {
int x = block.getX() + p.x;
int y = block.getY() + p.y;
if (x < 0 || x >= BOARD_WIDTH || y >= BOARD_HEIGHT || board[y][x] != 0) {
return true;
}
}
return false;
}
private void mergeBlocks() {
for (Point p : currentBlock.getPoints()) {
int x = currentBlock.getX() + p.x;
int y = currentBlock.getY() + p.y;
board[y][x] = currentBlock.getType() + 1;
}
blocks.remove(currentBlock);
}
private void clearLines() {
int lines = 0;
for (int i = BOARD_HEIGHT - 1; i >= 0; i--) {
boolean isLineFull = true;
for (int j = 0; j < BOARD_WIDTH; j++) {
if (board[i][j] == 0) {
isLineFull = false;
break;
}
}
if (isLineFull) {
for (int j = i; j > 0; j--) {
System.arraycopy(board[j - 1], 0, board[j], 0, BOARD_WIDTH);
}
lines++;
i++;
}
}
if (lines > 0) {
JFrame frame = (JFrame) getTopLevelAncestor();
frame.setTitle(String.format("Tetris - Score: %d", lines));
}
}
@Override
public void actionPerformed(ActionEvent e) {
currentBlock.moveDown();
if (isColliding(currentBlock)) {
currentBlock.moveUp();
mergeBlocks();
addNewBlock();
clearLines();
}
repaint();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
for (int i = 0; i < BOARD_HEIGHT; i++) {
for (int j = 0; j < BOARD_WIDTH; j++) {
int type = board[i][j];
if (type > 0) {
g.setColor(getBlockColor(type));
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
g.setColor(Color.BLACK);
g.drawRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
for (Block block : blocks) {
int type = block.getType() + 1;
g.setColor(getBlockColor(type));
for (Point p : block.getPoints()) {
int x = block.getX() + p.x;
int y = block.getY() + p.y;
g.fillRect(x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
g.setColor(Color.BLACK);
g.drawRect(x * BLOCK_SIZE, y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
private Color getBlockColor(int type) {
switch (type) {
case 1:
return Color.BLUE;
case 2:
return Color.RED;
case 3:
return Color.GREEN;
case 4:
return Color.YELLOW;
case 5:
return Color.ORANGE;
case 6:
return Color.PINK;
case 7:
return Color.CYAN;
default:
return Color.WHITE;
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Tetris");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.add(new Tetris());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
private static class Block {
private int type;
private int x;
private int y;
private Point[] points;
public Block(int type, int x, int y) {
this.type = type;
this.x = x;
this.y = y;
this.points = getPointsForType(type);
}
public void moveLeft() {
x--;
}
public void moveRight() {
x++;
}
public void moveDown() {
y++;
}
public void moveUp() {
y--;
}
public int getType() {
return type;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public Point[] getPoints() {
return points;
}
private Point[] getPointsForType(int type) {
switch (type) {
case 0:
return new Point[]{new Point(0, 0), new Point(1, 0), new Point(0, 1), new Point(1, 1)};
case 1:
return new Point[]{new Point(0, 0), new Point(1, 0), new Point(2, 0), new Point(3, 0)};
case 2:
return new Point[]{new Point(0, 0), new Point(1, 0), new Point(0, 1), new Point(0, 2)};
case 3:
return new Point[]{new Point(0, 0), new Point(1, 0), new Point(1, 1), new Point(2, 1)};
case 4:
return new Point[]{new Point(0, 1), new Point(1, 1), new Point(1, 0), new Point(2, 0)};
case 5:
return new Point[]{new Point(0, 0), new Point(0, 1), new Point(1, 1), new Point(1, 2)};
case 6:
return new Point[]{new Point(1, 0), new Point(1, 1), new Point(0, 1), new Point(0, 2)};
default:
return new Point[0];
}
}
}
}
```
运行这段代码,你就可以在窗口中体验俄罗斯方块游戏了。
阅读全文
相关推荐
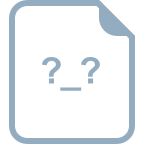
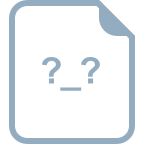
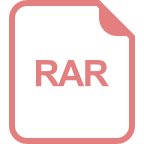












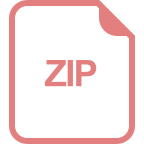
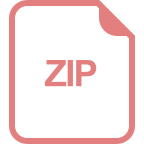
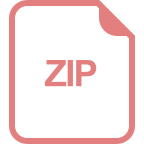
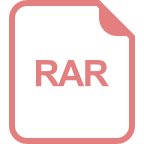