用java写一个俄罗斯方块小游戏
时间: 2023-12-07 11:36:10 浏览: 217
要用Java写一个俄罗斯方块小游戏,可以按照以下步骤进行:
1. 创建一个Java项目,并添加必要的库文件。
2. 创建一个游戏窗口,可以使用Java Swing或JavaFX等GUI库。
3. 实现俄罗斯方块的基本逻辑,包括方块的生成、下落、旋转、移动等操作。
4. 实现游戏的计分、等级、速度等功能。
5. 添加游戏音效和背景音乐。
6. 测试游戏并进行调试。
以下是一个简单的示例代码,可以作为参考:
```
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.Timer;
public class Tetris extends JPanel implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 300;
private static final int HEIGHT = 600;
private static final int BLOCK_SIZE = 30;
private int[][] board = new int[20][10];
private int[][][] shapes = {
{{1, 1, 1, 1}}, // I
{{1, 1, 0}, {0, 1, 1}}, // Z
{{0, 1, 1}, {1, 1, 0}}, // S
{{1, 1, 1}, {0, 1, 0}}, // T
{{1, 1, 1}, {1, 0, 0}}, // L
{{1, 1, 1}, {0, 0, 1}}, // J
{{1, 1}, {1, 1}} // O
};
private Color[] colors = {
Color.CYAN, Color.RED, Color.GREEN, Color.MAGENTA, Color.ORANGE, Color.BLUE, Color.YELLOW
};
private int currentShape;
private int currentX;
private int currentY;
private Timer timer;
private boolean isGameOver;
public Tetris() {
setPreferredSize(new Dimension(WIDTH, HEIGHT));
setBackground(Color.WHITE);
addKeyListener(this);
timer = new Timer(500, this);
timer.start();
newShape();
}
private void newShape() {
currentShape = new Random().nextInt(shapes.length);
currentX = 4;
currentY = 0;
if (!isValidMove(currentShape, currentX, currentY)) {
isGameOver = true;
timer.stop();
}
}
private boolean isValidMove(int shape, int x, int y) {
int[][] current = shapes[shape];
for (int i = 0; i < current.length; i++) {
for (int j = 0; j < current[i].length; j++) {
if (current[i][j] != 0 && (y + i >= board.length || x + j < 0 || x + j >= board[0].length || board[y + i][x + j] != 0)) {
return false;
}
}
}
return true;
}
private void move(int dx, int dy) {
if (!isValidMove(currentShape, currentX + dx, currentY + dy)) {
return;
}
currentX += dx;
currentY += dy;
repaint();
}
private void rotate() {
int[][] current = shapes[currentShape];
int[][] rotated = new int[current[0].length][current.length];
for (int i = 0; i < current.length; i++) {
for (int j = 0; j < current[i].length; j++) {
rotated[j][current.length - 1 - i] = current[i][j];
}
}
if (isValidMove(currentShape, currentX, currentY, rotated)) {
shapes[currentShape] = rotated;
repaint();
}
}
private boolean isValidMove(int shape, int x, int y, int[][] rotated) {
for (int i = 0; i < rotated.length; i++) {
for (int j = 0; j < rotated[i].length; j++) {
if (rotated[i][j] != 0 && (y + i >= board.length || x + j < 0 || x + j >= board[0].length || board[y + i][x + j] != 0)) {
return false;
}
}
}
return true;
}
private void drop() {
while (isValidMove(currentShape, currentX, currentY + 1)) {
currentY++;
}
place();
}
private void place() {
int[][] current = shapes[currentShape];
for (int i = 0; i < current.length; i++) {
for (int j = 0; j < current[i].length; j++) {
if (current[i][j] != 0) {
board[currentY + i][currentX + j] = currentShape + 1;
}
}
}
checkLines();
newShape();
}
private void checkLines() {
int lines = 0;
for (int i = board.length - 1; i >= 0; i--) {
boolean isLine = true;
for (int j = 0; j < board[i].length; j++) {
if (board[i][j] == 0) {
isLine = false;
break;
}
}
if (isLine) {
lines++;
for (int k = i; k > 0; k--) {
System.arraycopy(board[k - 1], 0, board[k], 0, board[k].length);
}
for (int j = 0; j < board[0].length; j++) {
board[0][j] = 0;
}
}
}
if (lines > 0) {
// update score, level, speed, etc.
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (isGameOver) {
return;
}
if (isValidMove(currentShape, currentX, currentY + 1)) {
currentY++;
} else {
place();
}
repaint();
}
@Override
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_LEFT:
move(-1, 0);
break;
case KeyEvent.VK_RIGHT:
move(1, 0);
break;
case KeyEvent.VK_DOWN:
move(0, 1);
break;
case KeyEvent.VK_UP:
rotate();
break;
case KeyEvent.VK_SPACE:
drop();
break;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) {
if (board[i][j] != 0) {
g.setColor(colors[board[i][j] - 1]);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
g.setColor(Color.BLACK);
g.drawRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
int[][] current = shapes[currentShape];
for (int i = 0; i < current.length; i++) {
for (int j = 0; j < current[i].length; j++) {
if (current[i][j] != 0) {
g.setColor(colors[currentShape]);
g.fillRect((currentX + j) * BLOCK_SIZE, (currentY + i) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
g.setColor(Color.BLACK);
g.drawRect((currentX + j) * BLOCK_SIZE, (currentY + i) * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Tetris");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.add(new Tetris());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
```
阅读全文
相关推荐
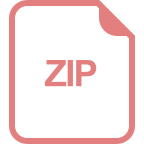





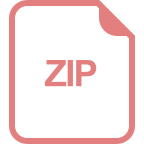
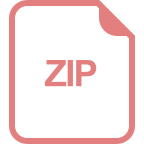
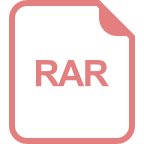
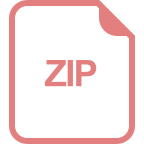


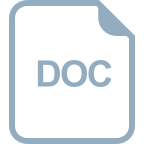
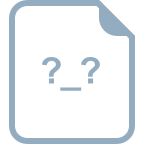