用Java编写一个俄罗斯方块游戏
时间: 2023-08-29 20:04:25 浏览: 75
好的,下面是一个简单的Java实现俄罗斯方块游戏的示例代码:
```java
import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Tetris extends JPanel implements KeyListener {
private static final long serialVersionUID = 1L;
private final int SIZE = 20; // 方格大小
private final int WIDTH = 10; // 游戏区宽度
private final int HEIGHT = 20; // 游戏区高度
private int[][] board = new int[HEIGHT][WIDTH]; // 游戏区矩阵
private int[][][] shapes = { // 七种不同形状的方块
{ { 1, 1, 1, 1 } },
{ { 1, 1, 0 }, { 0, 1, 1 } },
{ { 0, 1, 1 }, { 1, 1, 0 } },
{ { 1, 0, 0 }, { 1, 1, 1 } },
{ { 0, 0, 1 }, { 1, 1, 1 } },
{ { 1, 1 }, { 1, 1 } },
{ { 0, 1, 0 }, { 1, 1, 1 } } };
private Color[] colors = { // 每种方块的颜色
Color.cyan, Color.blue, Color.orange, Color.yellow, Color.green, Color.pink, Color.red };
private int[][] shape; // 当前方块
private int shapeType; // 当前方块类型
private int shapeX, shapeY; // 当前方块位置
private boolean gameOver; // 游戏是否结束
public Tetris() {
setPreferredSize(new java.awt.Dimension(WIDTH * SIZE, HEIGHT * SIZE));
setBackground(Color.black);
addKeyListener(this);
newGame();
}
private void newGame() {
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
board[i][j] = 0;
}
}
gameOver = false;
newShape();
repaint();
}
private void newShape() {
shapeType = (int) (Math.random() * shapes.length);
shape = shapes[shapeType];
shapeX = WIDTH / 2 - shape[0].length / 2;
shapeY = 0;
if (collision()) {
gameOver = true;
}
}
private boolean collision() {
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0 && (shapeY + i >= HEIGHT || shapeX + j < 0 || shapeX + j >= WIDTH || board[shapeY + i][shapeX + j] != 0)) {
return true;
}
}
}
return false;
}
private void merge() {
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0) {
board[shapeY + i][shapeX + j] = shapeType + 1;
}
}
}
checkLines();
newShape();
}
private void checkLines() {
for (int i = HEIGHT - 1; i >= 0; i--) {
boolean full = true;
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] == 0) {
full = false;
}
}
if (full) {
for (int k = i; k > 0; k--) {
for (int j = 0; j < WIDTH; j++) {
board[k][j] = board[k - 1][j];
}
}
i++;
}
}
}
private void moveDown() {
shapeY++;
if (collision()) {
shapeY--;
merge();
}
repaint();
}
private void moveLeft() {
shapeX--;
if (collision()) {
shapeX++;
}
repaint();
}
private void moveRight() {
shapeX++;
if (collision()) {
shapeX--;
}
repaint();
}
private void rotate() {
int[][] newShape = new int[shape[0].length][shape.length];
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
newShape[j][shape.length - 1 - i] = shape[i][j];
}
}
shape = newShape;
if (collision()) {
shape = new int[][] { { 1, 1, 1, 1 } };
}
repaint();
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyPressed(KeyEvent e) {
switch (e.getKeyCode()) {
case KeyEvent.VK_UP:
rotate();
break;
case KeyEvent.VK_DOWN:
moveDown();
break;
case KeyEvent.VK_LEFT:
moveLeft();
break;
case KeyEvent.VK_RIGHT:
moveRight();
break;
case KeyEvent.VK_SPACE:
while (!collision()) {
moveDown();
}
moveDown();
break;
case KeyEvent.VK_ENTER:
if (gameOver) {
newGame();
}
break;
}
}
@Override
public void keyReleased(KeyEvent e) {
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (board[i][j] != 0) {
g.setColor(colors[board[i][j] - 1]);
g.fillRect(j * SIZE, i * SIZE, SIZE, SIZE);
}
}
}
if (!gameOver) {
g.setColor(colors[shapeType]);
for (int i = 0; i < shape.length; i++) {
for (int j = 0; j < shape[i].length; j++) {
if (shape[i][j] != 0) {
g.fillRect((shapeX + j) * SIZE, (shapeY + i) * SIZE, SIZE, SIZE);
}
}
}
} else {
g.setColor(Color.white);
g.drawString("GAME OVER", WIDTH * SIZE / 2 - 30, HEIGHT * SIZE / 2 - 10);
g.drawString("Press ENTER to start a new game", WIDTH * SIZE / 2 - 80, HEIGHT * SIZE / 2 + 10);
}
}
public static void main(String[] args) {
JFrame frame = new JFrame("Tetris");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.setLocationRelativeTo(null);
frame.add(new Tetris());
frame.pack();
frame.setVisible(true);
}
}
```
这个代码会在一个游戏窗口中实现俄罗斯方块游戏,你可以通过上下左右箭头键来控制方块的移动和旋转,按空格键可以快速下落,按回车键可以重新开始游戏。
阅读全文
相关推荐
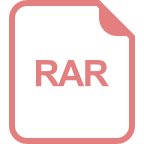
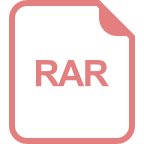
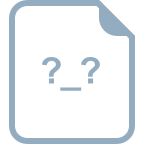
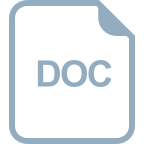
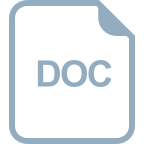

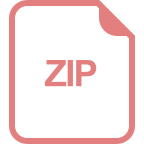
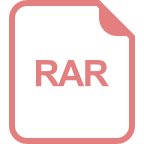
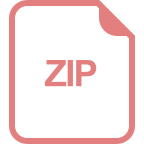
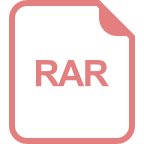
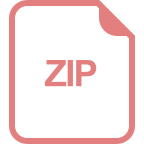
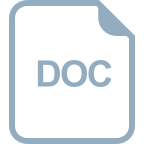
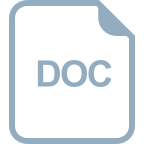
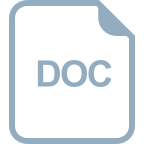

