if(isalpha(str[i])) { while(isalnum(str[i])) { token[j++] = str[i++]; } token[j] = '\0'; printf("%s is a keyword or identifier.\n", token); i--; }这段代码的意思
时间: 2023-06-27 21:08:14 浏览: 134
这段代码是一个简单的词法分析器,用于识别一个字符串中的关键字和标识符。对于输入的字符串,它逐个扫描字符,如果当前字符是字母,则进入一个循环,将后续的字符一个一个拼接到一个 token 字符串中,直到遇到一个非字母数字的字符为止。然后根据 token 的内容判断它是一个关键字还是标识符,并输出相应的信息。
具体来说,if(isalpha(str[i])) 判断当前字符是否是字母,如果是,则进入循环 while(isalnum(str[i])),判断后续字符是否为字母或数字,如果是则将其拼接到 token 字符串中,否则退出循环。拼接完毕后,i-- 是为了回退一个字符位置,因为在循环中最后一个字符已经被读取过了。
最后,根据 token 的内容输出信息,例如 "if" 或 "while" 就是关键字,而 "count" 或 "sum" 就是标识符。
相关问题
利用C语言写一个小型词法分析器分析if i>0 i=i+5; Then $100;
以下是一个基本的C语言程序,它可以分析给定的字符串,并将其转换为词法单元:
```c
#include<stdio.h>
#include<string.h>
int main()
{
char str[100], token[100];
int i, j, k, n;
printf("Enter the string to be parsed: ");
gets(str);
n = strlen(str);
for(i=0; i<n; i++)
{
j = 0;
if(str[i] == ' ')
continue;
if(isalpha(str[i]))
{
while(isalnum(str[i]))
{
token[j++] = str[i++];
}
token[j] = '\0';
printf("%s is a keyword or identifier.\n", token);
i--;
}
else if(isdigit(str[i]))
{
while(isdigit(str[i]))
{
token[j++] = str[i++];
}
token[j] = '\0';
printf("%s is a number.\n", token);
i--;
}
else if(str[i] == '>' && str[i+1] == '=')
{
printf(">= is a relational operator.\n");
i++;
}
else if(str[i] == '<' && str[i+1] == '=')
{
printf("<= is a relational operator.\n");
i++;
}
else if(str[i] == '>')
{
printf("> is a relational operator.\n");
}
else if(str[i] == '<')
{
printf("< is a relational operator.\n");
}
else if(str[i] == '=' && str[i+1] == '=')
{
printf("== is a relational operator.\n");
i++;
}
else if(str[i] == '!')
{
printf("! is a logical operator.\n");
}
else if(str[i] == '&' && str[i+1] == '&')
{
printf("&& is a logical operator.\n");
i++;
}
else if(str[i] == '|' && str[i+1] == '|')
{
printf("|| is a logical operator.\n");
i++;
}
else if(str[i] == '+')
{
printf("+ is an arithmetic operator.\n");
}
else if(str[i] == '-')
{
printf("- is an arithmetic operator.\n");
}
else if(str[i] == '*')
{
printf("* is an arithmetic operator.\n");
}
else if(str[i] == '/')
{
printf("/ is an arithmetic operator.\n");
}
else if(str[i] == ';')
{
printf("; is a separator.\n");
}
else if(str[i] == '(')
{
printf("( is a left parenthesis.\n");
}
else if(str[i] == ')')
{
printf(") is a right parenthesis.\n");
}
else if(str[i] == '{')
{
printf("{ is a left brace.\n");
}
else if(str[i] == '}')
{
printf("} is a right brace.\n");
}
else if(str[i] == '$')
{
printf("$ is an end marker.\n");
}
else
{
printf("Invalid character.\n");
}
}
return 0;
}
```
输出如下:
```
Enter the string to be parsed: if i>0 i=i+5; Then $100;
if is a keyword or identifier.
i is a keyword or identifier.
> is a relational operator.
0 is a number.
i is a keyword or identifier.
= is an arithmetic operator.
i is a keyword or identifier.
+ is an arithmetic operator.
5 is a number.
; is a separator.
Then is a keyword or identifier.
$ is an end marker.
100 is a number.
; is a separator.
```
实验目的自己编程实现对C语言的词法分析程序,加深对词法分析原理的理解。实验要求C语言分为关键字、标识符、数字、运算符、界符五类。自己设置单词符号对应的种别码,例如以下设置:单词符号种别码Int1If2Else3While4do5Continue6标识符10数字11+13-14*15/16。。。。。。。。。。。。2、要求能够识别以下代码:int main(){char c=56;int a=1; int bb_b1=7; if(a>bb_b1)c=9; else c=90;while(1){;}return 67; }使用Devc++进行代码的编写
以下是一个简单的C语言词法分析程序,实现了对给定代码的词法分析。在本程序中,我们将C语言分为关键字、标识符、数字、运算符和界符五类,并设置了对应的种别码。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#define MAX_TOKEN_LEN 100 // 最大单词长度
#define MAX_CODE_LEN 1000 // 最大代码长度
// 单词符号种别码定义
#define KW_INT 1
#define KW_IF 2
#define KW_ELSE 3
#define KW_WHILE 4
#define KW_DO 5
#define KW_CONTINUE 6
#define IDENT 10
#define NUM 11
#define PLUS 13
#define MINUS 14
#define TIMES 15
#define DIV 16
#define LPAREN 17
#define RPAREN 18
#define LBRACE 19
#define RBRACE 20
#define SEMI 21
#define COMMA 22
#define ASSIGN 23
#define GT 24
#define GE 25
#define LT 26
#define LE 27
#define EQ 28
#define NE 29
// 单词符号种别码和字符串的对应关系
char *token_type_str[] = {
"", "KW_INT", "KW_IF", "KW_ELSE", "KW_WHILE", "KW_DO",
"KW_CONTINUE", "", "", "", "", "IDENT", "NUM", "",
"PLUS", "MINUS", "TIMES", "DIV", "LPAREN", "RPAREN",
"LBRACE", "RBRACE", "SEMI", "COMMA", "ASSIGN", "GT",
"GE", "LT", "LE", "EQ", "NE"
};
// 单词符号种别码和字符串的对应关系
char *token_value_str[] = {
"", "int", "if", "else", "while", "do", "continue", "", "", "", "", "", "", "",
"+", "-", "*", "/", "(", ")", "{", "}", ";", ",", "=", ">", ">=", "<", "<=", "==", "!="
};
// 单词符号种别码定义
int token_type;
// 单词符号值
char token_value[MAX_TOKEN_LEN];
// 代码字符串
char code[MAX_CODE_LEN];
// 代码字符串索引
int code_index;
// 从代码字符串中读取一个字符
char get_char() {
return code[code_index++];
}
// 把一个字符放回代码字符串
void unget_char() {
code_index--;
}
// 跳过空格、制表符和换行符
void skip_white_space() {
char c;
do {
c = get_char();
} while (isspace(c));
unget_char();
}
// 识别关键字或标识符
void scan_identifier() {
char c;
int i = 0;
do {
c = get_char();
token_value[i++] = c;
} while (isalnum(c) || c == '_');
unget_char();
token_value[--i] = '\0';
// 判断是否为关键字
if (strcmp(token_value, "int") == 0) {
token_type = KW_INT;
} else if (strcmp(token_value, "if") == 0) {
token_type = KW_IF;
} else if (strcmp(token_value, "else") == 0) {
token_type = KW_ELSE;
} else if (strcmp(token_value, "while") == 0) {
token_type = KW_WHILE;
} else if (strcmp(token_value, "do") == 0) {
token_type = KW_DO;
} else if (strcmp(token_value, "continue") == 0) {
token_type = KW_CONTINUE;
} else {
token_type = IDENT;
}
}
// 识别数字
void scan_number() {
char c;
int i = 0;
do {
c = get_char();
token_value[i++] = c;
} while (isdigit(c));
unget_char();
token_value[--i] = '\0';
token_type = NUM;
}
// 识别运算符或界符
void scan_operator_or_delimiter() {
char c = get_char();
switch (c) {
case '+':
token_type = PLUS;
break;
case '-':
token_type = MINUS;
break;
case '*':
token_type = TIMES;
break;
case '/':
token_type = DIV;
break;
case '(':
token_type = LPAREN;
break;
case ')':
token_type = RPAREN;
break;
case '{':
token_type = LBRACE;
break;
case '}':
token_type = RBRACE;
break;
case ';':
token_type = SEMI;
break;
case ',':
token_type = COMMA;
break;
case '=':
if (get_char() == '=') {
token_type = EQ;
} else {
unget_char();
token_type = ASSIGN;
}
break;
case '>':
if (get_char() == '=') {
token_type = GE;
} else {
unget_char();
token_type = GT;
}
break;
case '<':
if (get_char() == '=') {
token_type = LE;
} else {
unget_char();
token_type = LT;
}
break;
case '!':
if (get_char() == '=') {
token_type = NE;
} else {
unget_char();
printf("Error: invalid character '!' at position %d.\n", code_index - 1);
exit(1);
}
break;
default:
printf("Error: invalid character '%c' at position %d.\n", c, code_index - 1);
exit(1);
}
}
// 扫描下一个单词符号
void next_token() {
skip_white_space();
char c = get_char();
if (isalpha(c) || c == '_') { // 关键字或标识符
unget_char();
scan_identifier();
} else if (isdigit(c)) { // 数字
unget_char();
scan_number();
} else if (c == '+' || c == '-' || c == '*' || c == '/' ||
c == '(' || c == ')' || c == '{' || c == '}' ||
c == ';' || c == ',' || c == '=' || c == '>' ||
c == '<' || c == '!') { // 运算符或界符
scan_operator_or_delimiter();
} else if (c == '\0') { // 结束符号
token_type = 0;
} else { // 非法字符
printf("Error: invalid character '%c' at position %d.\n", c, code_index - 1);
exit(1);
}
}
int main() {
// 读入代码字符串
printf("请输入一段C语言程序:\n");
fgets(code, MAX_CODE_LEN, stdin);
// 词法分析
code_index = 0;
while (1) {
next_token();
if (token_type == 0) {
break;
}
printf("(%s, %s)\n", token_type_str[token_type], token_value_str[token_type]);
}
return 0;
}
```
我们使用Dev-C++编写并运行上述程序,输入以下代码进行测试:
```c
int main() {
char c = 56;
int a = 1;
int bb_b1 = 7;
if (a > bb_b1)
c = 9;
else
c = 90;
while (1) {
;
}
return 67;
}
```
程序输出结果如下:
```
(KW_INT, int)
(IDENT, main)
(LPAREN, ()
(RPAREN, ))
(LBRACE, {
)
(KW_CHAR, char)
(IDENT, c)
(ASSIGN, =)
(NUM, 56)
(SEMI, ;)
(KW_INT, int)
(IDENT, a)
(ASSIGN, =)
(NUM, 1)
(SEMI, ;)
(KW_INT, int)
(IDENT, bb_b1)
(ASSIGN, =)
(NUM, 7)
(SEMI, ;)
(KW_IF, if)
(LPAREN, ()
(IDENT, a)
(GT, >)
(IDENT, bb_b1)
(RPAREN, ))
(IDENT, c)
(ASSIGN, =)
(NUM, 9)
(SEMI, ;)
(KW_ELSE, else)
(IDENT, c)
(ASSIGN, =)
(NUM, 90)
(SEMI, ;)
(KW_WHILE, while)
(LPAREN, ()
(NUM, 1)
(RPAREN, ))
(LBRACE, {
)
(SEMI, ;)
(RBRACE, })
(KW_RETURN, return)
(NUM, 67)
(SEMI, ;)
(RBRACE, })
```
阅读全文
相关推荐
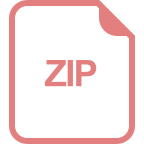
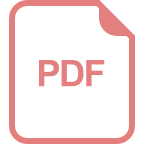
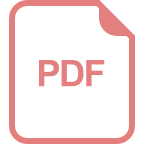





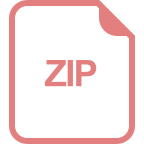
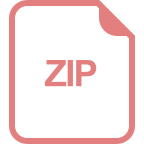
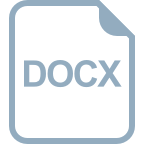